
Car Sales System Mini Project
The Car Sales System Mini Project has evolved to be an essential tool for both consumers and administrators in the car sales industry. This innovative platform provides a seamless environment for users to explore various car options and for admins to manage transactions efficiently. Here’s a closer look at its key features and functionalities.

Overview
This system is specifically designed to facilitate a hassle-free car-buying experience. From viewing car details to sorting options based on multiple criteria, users get to navigate through a comprehensive catalog of choices. Administrators, on the other hand, can keep track of transactions and inventories through a robust SQL database.
How to Use the Car Sales System Mini Project
- Download and Unzip: The first step is to download the project files and unzip them on your local machine.
- Install Required Software: Install Visual Studio and SQL Server Management Studio for database management.
- Link Database: Link your SQL database, preferably SQL 2008 or higher, to store transaction and car data.
- Run Project: Open the project in Visual Studio and run the application to start your Car Sales System Mini Project.
Key Features
- Car Sales System Project Synopsis: The project offers a synopsis feature that outlines the scope, objectives, and methodologies involved.
- Project Documentation: Detailed car sales system project documentation is provided for a better understanding of the project’s architecture and functionalities.
- PPT Download: The car sales system project ppt download is available, offering a slide-by-slide presentation on the project overview, design, and features.
Resources
- Car Sales Database Download: Get access to a downloadable database that contains car details and transaction records.
- Car for Sale Download: Users can download car details and brochures for offline viewing and comparisons.
Additional Information
For those interested in delving deeper into this project, there is an option to download the entire project, including its documentation and PPT presentation. This is particularly useful for researchers, students, and businesses looking to adopt or adapt the system for their specific needs.
Sample Code
Database Setup (MySQL)
CREATE DATABASE CarSalesDB;
USE CarSalesDB;
CREATE TABLE cars (
id INT AUTO_INCREMENT PRIMARY KEY,
make VARCHAR(50),
model VARCHAR(50),
year INT,
price INT
);
INSERT INTO cars (make, model, year, price) VALUES
('Toyota', 'Camry', 2021, 24000),
('Honda', 'Civic', 2020, 20000),
('Ford', 'Fusion', 2022, 23000);
HTML & JavaScript
<!DOCTYPE html>
<html>
<head>
<title>Car Sales System</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<h1>Car Sales System Mini Project</h1>
<button onclick="sortCars('price')">Sort by Price</button>
<div id="carList">
<!-- Car details will be populated here -->
</div>
<script>
let cars = [];
function fetchCars() {
// Normally you would fetch data from server here.
// For the sake of simplicity, we are using static data.
$.ajax({
url: 'fetch_cars.php',
type: 'GET',
success: function(response) {
cars = JSON.parse(response);
displayCars();
}
});
}
function displayCars() {
let html = '';
cars.forEach(car => {
html += `<div>
<h2>${car.make} ${car.model}</h2>
<p>Year: ${car.year}</p>
<p>Price: ${car.price}</p>
</div>`;
});
$('#carList').html(html);
}
function sortCars(key) {
cars.sort((a, b) => a[key] - b[key]);
displayCars();
}
fetchCars();
</script>
</body>
</html>
PHP (fetch_cars.php)
<?php
$servername = "localhost";
$username = "root";
$password = "";
$dbname = "CarSalesDB";
$conn = new mysqli($servername, $username, $password, $dbname);
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
$sql = "SELECT id, make, model, year, price FROM cars";
$result = $conn->query($sql);
$cars = [];
if ($result->num_rows > 0) {
while($row = $result->fetch_assoc()) {
$cars[] = $row;
}
}
echo json_encode($cars);
$conn->close();
?>
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Car Sales System Mini Project PDF
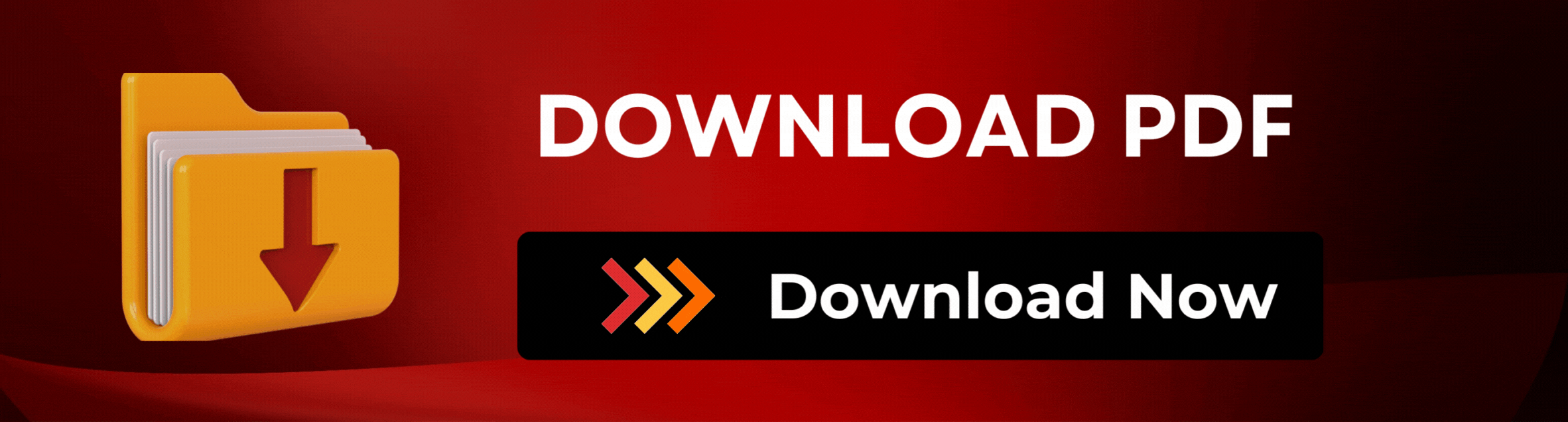