
Voice-based Intelligent Virtual Assistance for Windows Project
Introduction
Voice technology has been making waves in the consumer market, but its potential for transforming the way we interact with our Windows PCs is just beginning to be realized. Intelligent Virtual Assistants are no longer just for setting reminders or sending texts; they are evolving into powerful tools that can handle complex tasks and improve productivity. This article delves into the features, advantages, and limitations of using an Intelligent Virtual Assistant on your Windows computer.
Features
Command Execution
With simple voice commands like “Open Notepad” or “Open Google Chrome,” the assistant can swiftly launch applications, saving you the time and effort of navigating through menus.
Text-to-Speech and Speech-to-Text
The assistant is capable of converting text to speech and vice versa, making it easier for users to interact with their PCs without using a keyboard or mouse.
Search Capabilities
By using the command “search,” users can easily find files, applications, or even browse the internet, all through voice commands.
System Control
Need to shut down or restart your computer? Just say the word, and the assistant will handle it for you.

Advantages
- Ease of Use: The assistant’s intuitive voice recognition makes it accessible to users of all ages and tech-savviness levels.
- Efficiency: By converting text to speech and executing commands swiftly, the assistant can significantly speed up tasks and improve productivity.
- Compatibility: The assistant is designed to work seamlessly with Windows 7 and above, making it widely accessible.
Limitations
- Internet Dependency: The assistant requires an active internet connection for optimal functionality.
- Accuracy: The assistant’s performance can be compromised if the user’s commands are unclear or ambiguous.
Conclusion
Intelligent Virtual Assistants for Windows are not just a futuristic concept; they are a reality that can significantly enhance your computing experience. While there are some limitations, the benefits far outweigh the drawbacks, making it a must-try feature for any Windows user.
Sample Code
First, install the required packages:
pip install SpeechRecognition
pip install pyttsx3
import speech_recognition as sr
import pyttsx3
import os
# Initialize text-to-speech engine
engine = pyttsx3.init()
# Function to speak text
def speak(text):
engine.say(text)
engine.runAndWait()
# Function to recognize speech
def recognize_speech():
r = sr.Recognizer()
mic = sr.Microphone()
with mic as source:
print("Listening...")
audio_data = r.listen(source)
try:
print("Recognizing...")
text = r.recognize_google(audio_data)
return text.lower()
except:
speak("Sorry, I didn't get that.")
return None
# Main function
if __name__ == "__main__":
speak("Hello, how can I assist you today?")
while True:
command = recognize_speech()
if command is None:
continue
if "open notepad" in command:
os.system("notepad.exe")
speak("Opening Notepad.")
elif "open chrome" in command:
os.system("chrome.exe")
speak("Opening Google Chrome.")
elif "shutdown" in command:
speak("Shutting down the system.")
os.system("shutdown /s /t 1")
elif "restart" in command:
speak("Restarting the system.")
os.system("shutdown /r /t 1")
elif "exit" in command:
speak("Goodbye!")
break
This is a very basic example and doesn’t cover all the functionalities mentioned in the article. However, it should give you a good starting point. You can extend this code to include more advanced features like natural language understanding, more complex command execution, and integration with other services or databases.
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Voice-based Intelligent Virtual Assistance for Windows Project PDF
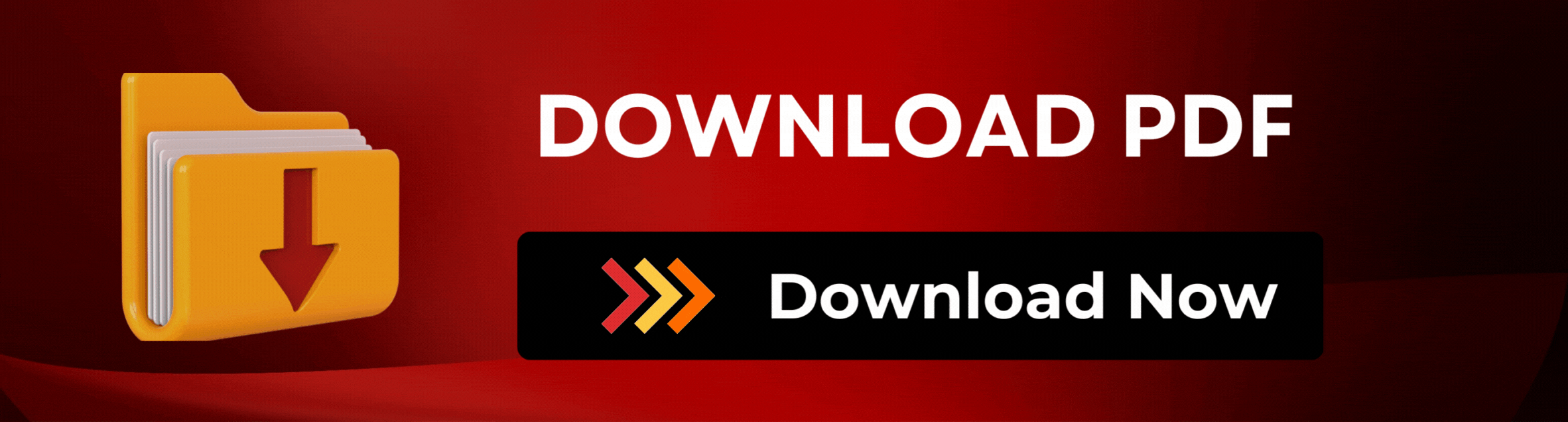