
Web Design with Web Page Builder
Introduction
In the digital age, having a web presence is crucial, but not everyone has the time or skills to write code from scratch. Enter the Web Page Builder: a user-friendly tool that allows anyone to create beautiful, functional websites without needing to know a single line of coding. This application is designed for both personal and professional use, providing an array of customizable templates and a simple drag-and-drop interface.

How the Web Page Builder Works
- Template Selection: Users start by choosing from a variety of web page templates. These templates are designed to suit different styles and industries, ensuring there’s something for everyone.
- Customization: Through an intuitive graphical user interface (GUI), users can add, remove, or modify elements on their web page. This includes text, images, buttons, and more.
- Drag & Drop Interface: The core of the builder’s ease of use is its drag-and-drop functionality. Users can visually construct their web pages by moving elements around the template.
- Preview and Publish: At any time, users can preview their web page. Once satisfied, they can publish it directly from the Web Page Builder, making their site live on the internet.
Advantages
- No Coding Required: Users don’t need any programming knowledge to create a website, making web design accessible to everyone.
- Quick and Easy: The builder’s intuitive design and pre-made templates mean users can create web pages quickly, focusing on content and design rather than technical details.
- Customizable: Users aren’t locked into rigid designs. The builder allows for customization, enabling users to create unique web pages that stand out.
- Saves Time: By streamlining the web design process, users can get their websites up and running faster, allowing them to concentrate on other aspects of their work or business.
Disadvantages
- Static Content Limitation: Without coding knowledge, users are typically limited to creating static websites, which might not suit all purposes.
- Template Dependency: The creativity and uniqueness of a website might be limited by the number and variety of templates available within the application.
Conclusion
Our Web Page Builder is a gateway to the world of web design, democratizing the ability to create and publish websites. It’s an ideal solution for individuals and businesses looking to establish a web presence quickly and efficiently. With a focus on user-friendly design and functionality, the Web Page Builder empowers users to bring their vision to the digital world.

Sample Code
HTML Structure:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Simple Web Page Builder</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div id="toolbar">
<button onclick="addText()">Add Text</button>
<button onclick="addImage()">Add Image</button>
</div>
<div id="canvas">
<!-- Users will build their webpage here -->
</div>
<script src="script.js"></script>
</body>
</html>
CSS Styling:
#canvas {
min-height: 500px;
border: 2px dashed #ccc;
position: relative;
overflow: auto;
}
.draggable {
width: 100px;
height: 100px;
position: absolute;
cursor: move;
}
.text-box {
border: 1px solid black;
padding: 5px;
}
.image-box {
width: 100px;
height: 100px;
background: url('example-image.jpg') no-repeat center center;
background-size: cover;
}
JavaScript for Drag and Drop Functionality:
function addText() {
const box = document.createElement('div');
box.classList.add('draggable', 'text-box');
box.innerText = 'Edit Text';
box.contentEditable = true;
box.style.left = '10px';
box.style.top = '10px';
document.getElementById('canvas').appendChild(box);
addDraggableFunctionality(box);
}
function addImage() {
const box = document.createElement('div');
box.classList.add('draggable', 'image-box');
box.style.left = '10px';
box.style.top = '50px';
document.getElementById('canvas').appendChild(box);
addDraggableFunctionality(box);
}
function addDraggableFunctionality(elem) {
elem.onmousedown = function(event) {
let shiftX = event.clientX - elem.getBoundingClientRect().left;
let shiftY = event.clientY - elem.getBoundingClientRect().top;
elem.style.position = 'absolute';
elem.style.zIndex = 1000;
document.body.append(elem);
moveAt(event.pageX, event.pageY);
// moves the elem at (pageX, pageY) coordinates
// taking initial shifts into account
function moveAt(pageX, pageY) {
elem.style.left = pageX - shiftX + 'px';
elem.style.top = pageY - shiftY + 'px';
}
function onMouseMove(event) {
moveAt(event.pageX, event.pageY);
}
// move the elem on mousemove
document.addEventListener('mousemove', onMouseMove);
// drop the elem, remove unneeded handlers
elem.onmouseup = function() {
document.removeEventListener('mousemove', onMouseMove);
elem.onmouseup = null;
};
};
elem.ondragstart = function() {
return false;
};
}
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Web Design with Web Page Builder PDF
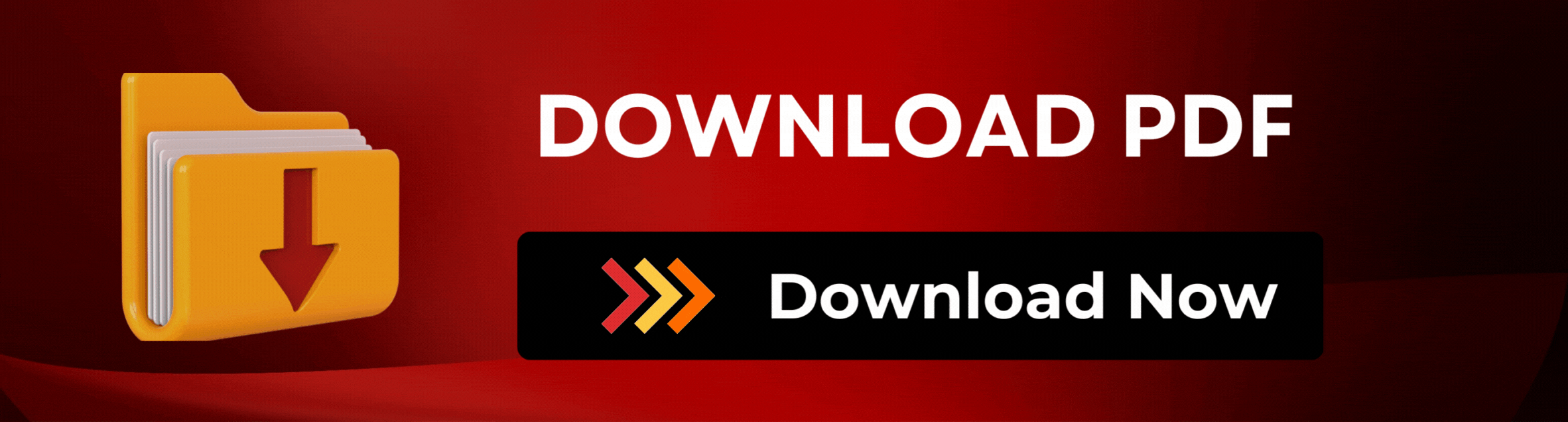