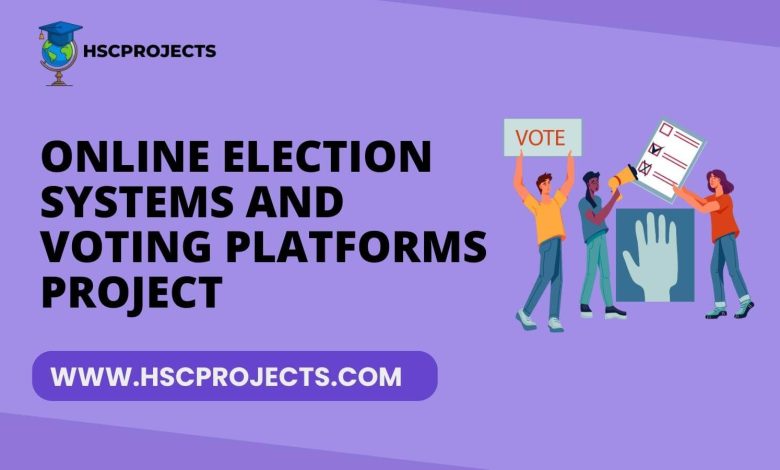
Online Election Systems and Voting Platforms Project
Introduction
The digital age has brought about significant changes in various sectors, including governance and democracy. One of the most impactful innovations is the Online Election System, a platform that streamlines the electoral process, making it more efficient, transparent, and secure. This article delves into the features, advantages, and challenges of implementing an online voting system project.

Key Modules of an Online Election System
- Admin Login: Controlled by the Election Commission, this module allows for the overall management of the election process.
- Candidate Document Verification: Ensures that all candidates meet the necessary legal requirements.
- Candidate Login: A secure portal where candidates can upload their credentials and campaign milestones.
- Voters Login: A secure environment where voters can cast their votes.
- Election Monitoring Dashboard: Real-time analytics and monitoring of the election process.
- Result Calculation Module: Automated and secure calculation of election results.
- Election Creation Module: Allows the admin to create new elections, define constituencies, and set election dates.
- Voting Conduction Module: Manages the actual process of voting, ensuring it is secure and transparent.
Advantages
- Efficiency: Online voting systems significantly speed up the election process.
- Transparency: Voters can view the background and credentials of each candidate, making for a more informed electorate.
- Security: System-generated unique IDs and passwords ensure secure logins.
- Accessibility: Enables people to vote from the comfort of their homes, thereby increasing voter turnout.
- Admin Oversight: The admin dashboard allows for real-time monitoring and immediate action in case of discrepancies.
Challenges
- Cybersecurity Risks: The system is susceptible to hacking, which could compromise the integrity of the election.
- Digital Divide: Not all voters have access to a stable internet connection or the necessary hardware to participate in online voting.
Conclusion
Online election systems offer a promising avenue for making democratic processes more efficient and inclusive. However, there are challenges that need to be addressed, particularly in the areas of cybersecurity and accessibility. With advancements in technology and a focus on security, online voting systems could very well be the future of democratic elections.
Sample Code
First, install Flask if you haven’t already:
pip install Flask
Create a new Python file app.py
and add the following code:
from flask import Flask, render_template, request, redirect, url_for
app = Flask(__name__)
# Dummy data for candidates and votes
candidates = {'Alice': 0, 'Bob': 0, 'Charlie': 0}
admin_password = 'admin123'
@app.route('/')
def index():
return render_template('index.html', candidates=candidates)
@app.route('/vote', methods=['POST'])
def vote():
candidate = request.form.get('candidate')
if candidate in candidates:
candidates[candidate] += 1
return redirect(url_for('index'))
@app.route('/admin', methods=['GET', 'POST'])
def admin():
if request.method == 'POST':
password = request.form.get('password')
if password == admin_password:
return render_template('admin.html', candidates=candidates)
return render_template('admin_login.html')
if __name__ == '__main__':
app.run(debug=True)
Create an HTML file templates/index.html
:
<!DOCTYPE html>
<html>
<head>
<title>Online Election System</title>
</head>
<body>
<h1>Vote for Your Candidate</h1>
<form action="/vote" method="post">
{% for candidate, votes in candidates.items() %}
<input type="radio" name="candidate" value="{{ candidate }}"> {{ candidate }}<br>
{% endfor %}
<input type="submit" value="Vote">
</form>
</body>
</html>
Create another HTML file templates/admin_login.html
:
<!DOCTYPE html>
<html>
<head>
<title>Admin Login</title>
</head>
<body>
<h1>Admin Login</h1>
<form action="/admin" method="post">
<input type="password" name="password" placeholder="Password"><br>
<input type="submit" value="Login">
</form>
</body>
</html>
create templates/admin.html
:
<!DOCTYPE html>
<html>
<head>
<title>Admin Dashboard</title>
</head>
<body>
<h1>Admin Dashboard</h1>
<h2>Candidate Votes</h2>
<ul>
{% for candidate, votes in candidates.items() %}
<li>{{ candidate }}: {{ votes }} votes</li>
{% endfor %}
</ul>
</body>
</html>
To run the project, execute app.py
:
python app.py
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Online Election Systems and Voting Platforms Project PDF
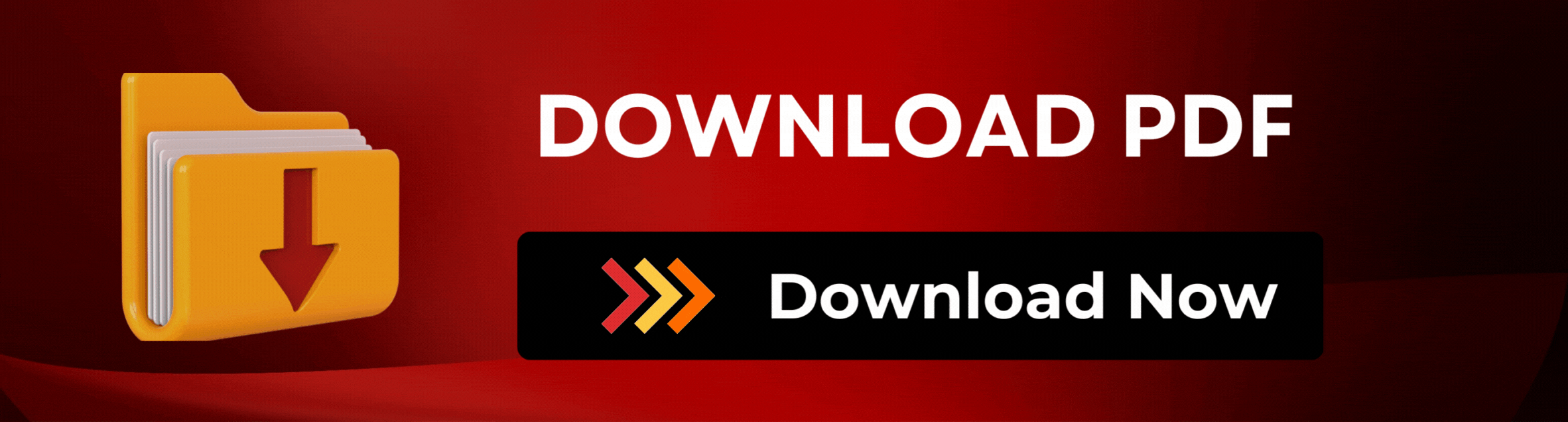