
School Bus Tracking System Project
Introduction
The advancement of technology has brought a plethora of benefits to our daily lives, especially in terms of safety and convenience. One such boon is the School Bus Tracking System, a combination of web and Android applications designed to enhance the safety and monitoring of school transportation. This guide provides a deep dive into the features, setup, and benefits of the bus tracking system project, as well as a downloadable PDF and source code for your convenience.

System Architecture
The project is segmented into two primary interfaces: the web application for administrators and parents, and the Android application exclusively for drivers. The Android application, once logged in, triggers the GPS location tracker that updates the bus’s location at regular intervals (every 5 minutes) into the system database.
Features and Functionalities
- Driver’s Module: The Android application focuses on the driver. Here, after logging in, the GPS coordinates of the bus are updated in the database in real-time.
- Admin Module: The admin has the role of registering new students and assigning them to a bus. They can also update bus and route details.
- Parent’s Dashboard: Parents have a separate login where they can track the real-time location of their child’s bus, ensuring peace of mind.
Advantages
- Real-Time Tracking: The bus tracking system for school offers parents the ability to track their children’s whereabouts during transit.
- Attendance Management: The system not only tracks the bus but also helps in monitoring the attendance of drivers.
- Data Accuracy: The GPS coordinates are updated regularly to ensure the data’s reliability and accuracy.
Disadvantages
- Smartphone Requirement: All drivers must have an Android smartphone to access the tracking application.
- Internet Dependency: A reliable internet connection is essential for the tracking system to function efficiently.
Technical Requirements
- Bus GPS Tracker: The central hardware requirement
- Android Phone: For the driver’s app
- Web Application: Accessible from any browser for parents and admins
Source Code and PDF
For those interested in a deeper technical exploration, we provide a comprehensive bus tracking system project source code and a downloadable PDF outlining the project’s specifics.
Conclusion
The School Bus Tracking System is a multifaceted project aimed at ensuring the safety and monitoring of school transportation. With a focus on real-time tracking, it provides a reliable and efficient means for parents to keep tabs on their children during their daily commute.
Sample Code
AndroidManifest.xml
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
MainActivity.java
package com.example.schoolbustrackingsystem;
import android.Manifest;
import android.content.pm.PackageManager;
import android.location.Location;
import android.os.Bundle;
import android.util.Log;
import android.widget.Toast;
import androidx.annotation.NonNull;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.app.ActivityCompat;
import com.google.android.gms.location.FusedLocationProviderClient;
import com.google.android.gms.location.LocationServices;
import com.google.android.gms.tasks.OnSuccessListener;
public class MainActivity extends AppCompatActivity {
private FusedLocationProviderClient fusedLocationClient;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
fusedLocationClient = LocationServices.getFusedLocationProviderClient(this);
if (ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
ActivityCompat.requestPermissions(this, new String[]{Manifest.permission.ACCESS_FINE_LOCATION}, 1);
} else {
getDeviceLocation();
}
}
private void getDeviceLocation() {
fusedLocationClient.getLastLocation()
.addOnSuccessListener(this, new OnSuccessListener<Location>() {
@Override
public void onSuccess(Location location) {
if (location != null) {
// TODO: Send this location data to your server
Log.d("Location", "Latitude: " + location.getLatitude() + ", Longitude: " + location.getLongitude());
Toast.makeText(MainActivity.this, "Location Captured", Toast.LENGTH_SHORT).show();
}
}
});
}
@Override
public void onRequestPermissionsResult(int requestCode, @NonNull String[] permissions, @NonNull int[] grantResults) {
super.onRequestPermissionsResult(requestCode, permissions, grantResults);
if (requestCode == 1) {
if (grantResults.length > 0 && grantResults[0] == PackageManager.PERMISSION_GRANTED) {
getDeviceLocation();
} else {
Toast.makeText(this, "Permission denied!", Toast.LENGTH_SHORT).show();
}
}
}
}
Server-side (Python Flask API)
from flask import Flask, request
app = Flask(__name__)
@app.route('/location', methods=['POST'])
def get_location():
latitude = request.json.get('latitude')
longitude = request.json.get('longitude')
# TODO: Save these into your database
print(f'Latitude: {latitude}, Longitude: {longitude}')
return {"message": "Location received"}, 200
if __name__ == '__main__':
app.run(debug=True)
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download School Bus Tracking System Project PDF
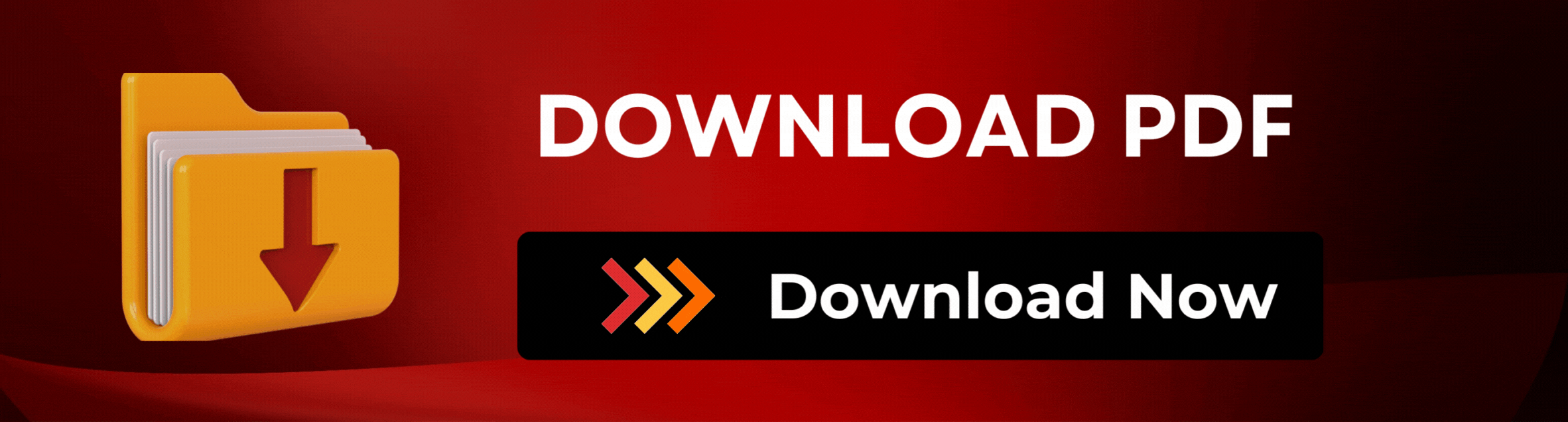