
Detecting Phishing Websites Using Advanced Machine Learning Techniques
Introduction
With the exponential growth of E-commerce and online transactions, the risk of encountering phishing websites has also escalated. These malicious websites often mimic legitimate platforms to steal sensitive user information such as usernames, passwords, and credit card details. This article delves into an advanced system that employs machine learning algorithms for phishing detection, thereby enhancing the security of online transactions.
The Mechanism Behind Phishing Detection Using Machine Learning
The core of this system lies in its use of classification algorithms in data mining. These algorithms analyze various characteristics of a website, including its URL structure, domain identity, and security protocols. By comparing these features against a database of known phishing criteria, the system can effectively classify a website as either legitimate or a phishing attempt.

Key Features
- URL and Domain Identity: The system scrutinizes the URL structure and domain identity to check for any suspicious elements commonly found in phishing websites.
- Security and Encryption Criteria: The presence or absence of security certificates and encryption protocols also play a crucial role in the classification process.
- Dynamic Learning: One of the standout features is the system’s ability to learn dynamically. It uses machine learning techniques to update its database with new suspicious keywords and criteria, making the system more robust over time.
Advantages
- Enhanced Security for E-commerce Platforms: This system can be integrated into various E-commerce platforms to provide an additional layer of security.
- User Confidence: Knowing that a robust phishing detection system is in place, users can make online payments without hesitation.
- Superior Performance: The data mining algorithms used in this system outperform traditional classification algorithms, offering more accurate and faster results.
Limitations
- Internet Dependency: The system requires a stable internet connection to function effectively.
- Centralized Data Storage: All website-related data is stored in a single location, which could be a point of vulnerability if not properly secured.
Conclusion
The integration of machine learning algorithms in phishing detection offers a promising avenue for enhancing online security. While the system has its limitations, its benefits far outweigh the drawbacks, making it an essential tool for any E-commerce platform concerned with security and customer satisfaction.
Sample Code
First, install the required packages if you haven’t already:
pip install numpy scikit-learn
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
import numpy as np
# Sample dataset: [URL Length, Has HTTPS, Domain Age, Has @ Symbol]
# Label: 1 for phishing, 0 for legitimate
X = np.array([
[10, 1, 5, 0], # Legitimate
[8, 1, 2, 0], # Legitimate
[15, 0, 1, 1], # Phishing
[20, 0, 1, 1], # Phishing
[6, 1, 3, 0], # Legitimate
[18, 0, 1, 1], # Phishing
])
y = np.array([0, 0, 1, 1, 0, 1])
# Split dataset into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Initialize and train classifier
clf = RandomForestClassifier(n_estimators=10)
clf.fit(X_train, y_train)
# Make predictions
y_pred = clf.predict(X_test)
# Evaluate the model
print("Accuracy:", accuracy_score(y_test, y_pred))
# To predict a new website
# new_website = np.array([[12, 0, 1, 1]]) # Replace these features with real ones
# prediction = clf.predict(new_website)
# print("Prediction:", prediction)
In this example, we use a RandomForestClassifier from Scikit-learn to classify websites as either phishing or legitimate based on some made-up features:
- URL Length
- Whether it uses HTTPS (1 for yes, 0 for no)
- Domain Age
- Presence of ‘@’ symbol in the URL (common in phishing URLs)
The labels are 1 for phishing websites and 0 for legitimate ones.
Please note that this is a very simplified example. In a real-world application, you’d collect a large dataset, extract various features, fine-tune the model, and possibly use more advanced techniques.
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Detecting Phishing Websites Using Advanced Machine Learning Techniques PDF
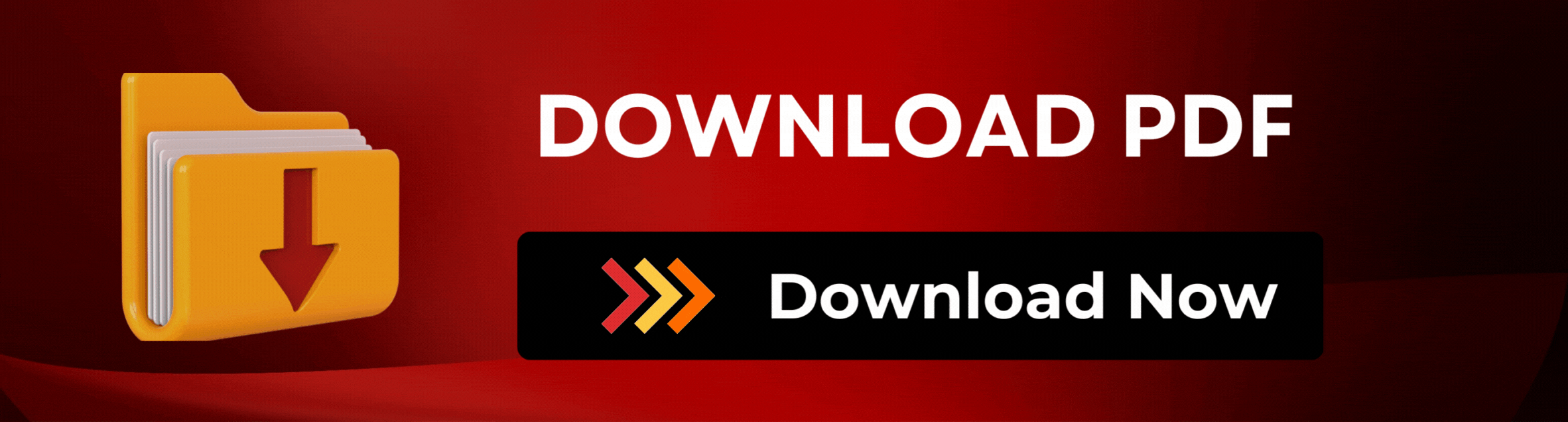