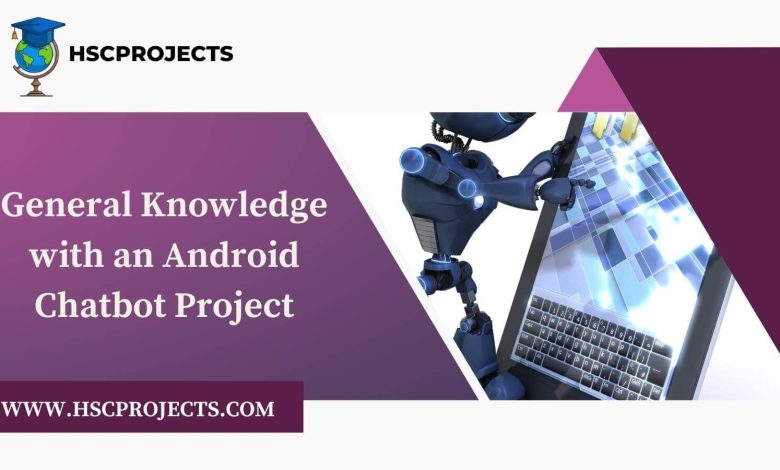
General Knowledge with an Android Chatbot Project
Introduction
As artificial intelligence and machine learning continue to evolve, the applications are endless. One such groundbreaking application is a chatbot using Android Studio, specifically designed to tackle general knowledge queries. Unlike standard search engines that provide a list of links, this Android chat bot directly supplies concise and accurate answers, revolutionizing how we access information.
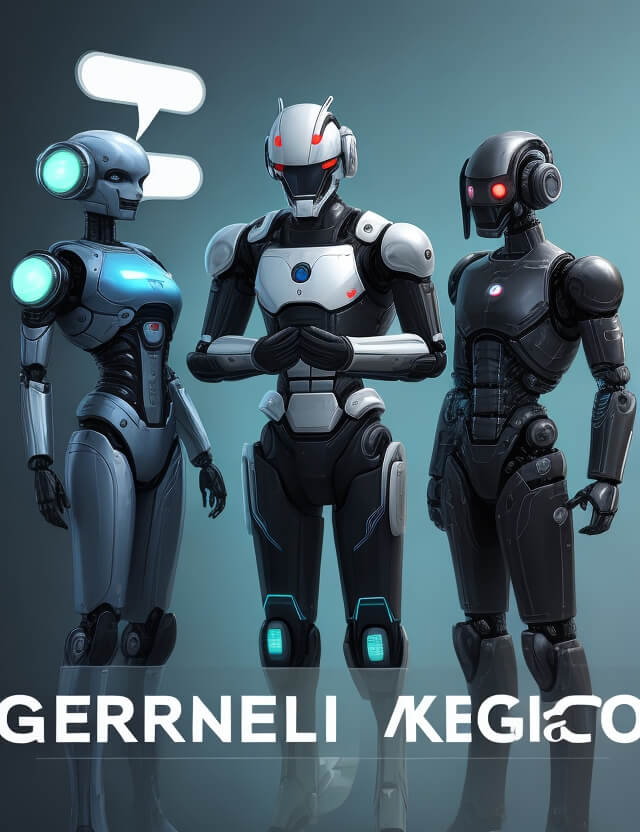
The Technology Behind the Chatbot
Building a chatbot android app isn’t just about coding; it’s about creating a sophisticated, user-friendly interface. Here are the core components:
- Algorithm: A highly optimized algorithm that analyses user queries to provide accurate responses.
- Database: A comprehensive collection of data across multiple domains like sports, health, and education.
- Admin Control: A dashboard for the admin to manage users, questions, and feedback.
Features and Advantages
- Fast Responses: Offers quick, reliable answers to a wide array of general knowledge questions.
- User Feedback: Allows users to rate the accuracy of answers, helping the system to continuously improve.
- One-Stop Solution: Eliminates the need to browse multiple sources for information.
Limitations and Solutions
While the chatbot android app offers numerous advantages, there are some constraints:
- Internet Dependency: Requires an active internet connection.
- Data Accuracy: May provide incorrect information if the database is not up-to-date.
However, these limitations can be mitigated by including an option to search Google for answers and by continuously updating the database based on user feedback.
How It’s Transforming Information Gathering
In today’s fast-paced world, this chat bot for Android offers a convenient, quick, and interactive way to access a wealth of general knowledge. No more aimless searching; get your answers instantly with our state-of-the-art chatbot android app.
Sample Code
MainActivity.java
package com.example.gkchatbot;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.EditText;
import android.widget.TextView;
import android.widget.Button;
public class MainActivity extends AppCompatActivity {
private EditText userQuery;
private TextView botReply;
private Button sendButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
userQuery = findViewById(R.id.userQuery);
botReply = findViewById(R.id.botReply);
sendButton = findViewById(R.id.sendButton);
sendButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String query = userQuery.getText().toString().toLowerCase();
String reply = analyzeQuery(query);
botReply.setText(reply);
}
});
}
private String analyzeQuery(String query) {
String reply;
switch (query) {
case "who is the president of the united states":
reply = "As of my last training data in January 2022, Joe Biden is the President of the United States.";
break;
case "what is the capital of france":
reply = "The capital of France is Paris.";
break;
default:
reply = "I'm sorry, I don't have the answer to that question.";
break;
}
return reply;
}
}
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<EditText
android:id="@+id/userQuery"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:hint="Enter your query here"/>
<Button
android:id="@+id/sendButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Send"
android:layout_below="@id/userQuery"
android:layout_marginTop="20dp"/>
<TextView
android:id="@+id/botReply"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/sendButton"
android:layout_marginTop="20dp"
android:text="Bot will reply here"/>
</RelativeLayout>
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download General Knowledge with an Android Chatbot Project PDF
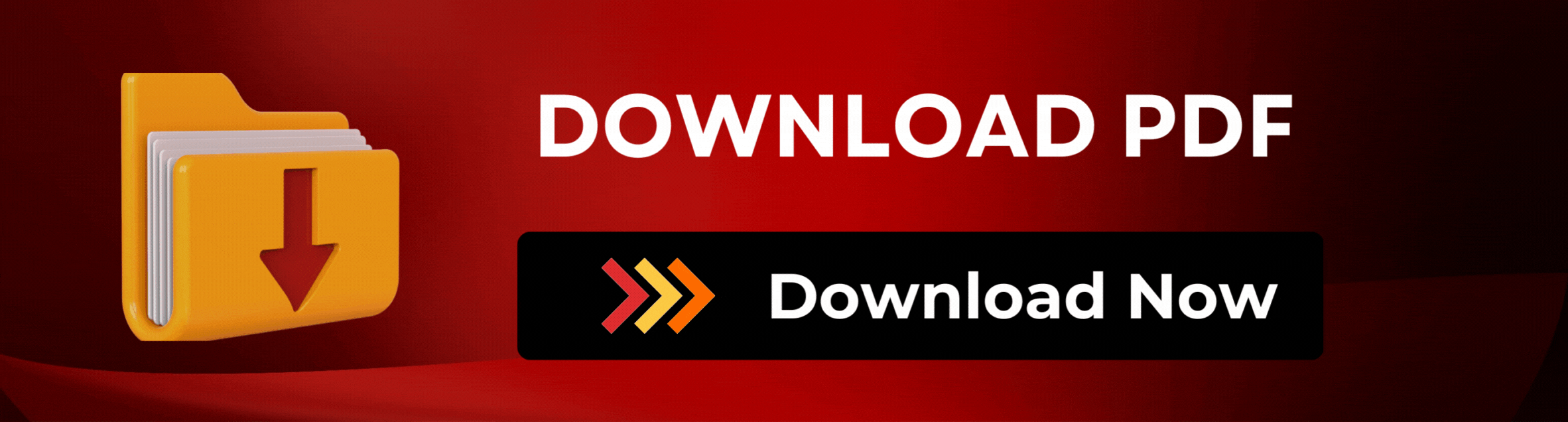