
Android Patient Tracker Project
Introduction
Healthcare management is undergoing a digital transformation, and one of the tools leading this change is the Android Patient Tracker Project. This project aims to provide a convenient and user-friendly platform for doctors to manage patient data efficiently. With features like patient history recording, medication tracking, and quick search options, this application has become a must-have tool for healthcare providers.
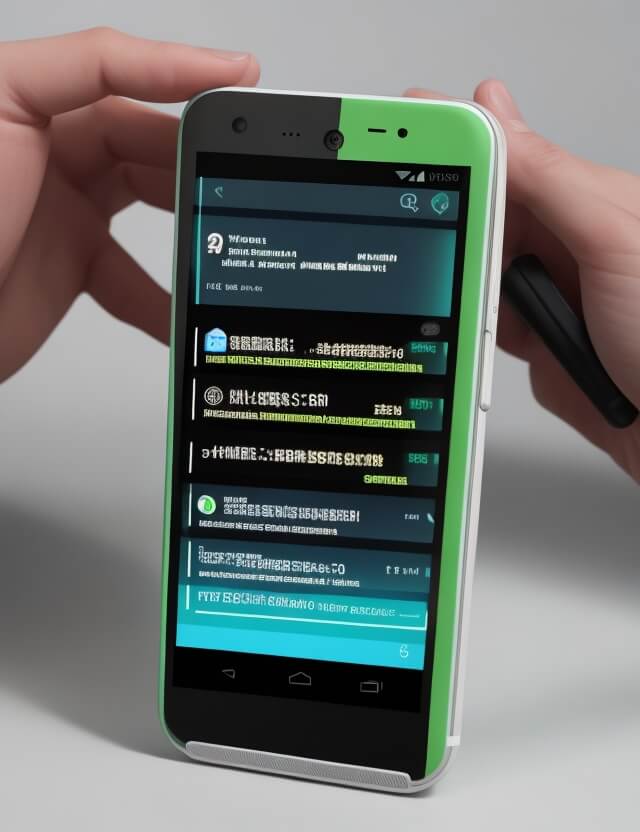
Features
Easy Data Entry
With just a few taps, doctors can enter a wide range of patient details, including the patient’s name, disease, medication prescribed, date of visit, and even associated costs. The user interface is designed for seamless navigation, making data entry effortless.
Instant Access
No more flipping through paper files or navigating cumbersome desktop software. Your patient data is readily accessible right from your Android device. You can search by patient name or by date, allowing you to retrieve patient information swiftly.
Security
Privacy and security are of utmost importance when it comes to healthcare data. The Android Patient Tracker Project ensures that all the data saved on your device is secure.
Flexibility
The system is highly adaptable, allowing doctors to customize data fields according to their specific needs. This feature makes it a flexible tool that can cater to a wide range of healthcare providers.
Advantages and Disadvantages
Advantages
- Portable and easily accessible, providing doctors the freedom to use it anytime, anywhere.
- Eliminates the need for paper-based records, thus making it an eco-friendly option.
- The application is both time-efficient and secure, streamlining the management of patient data.
Disadvantages
- Doctors must own an Android device to utilize this system.
- Data can only be accessed through this specific application and is not transferable to other apps or devices.
- The system does not provide information on dispensary stock details.
- It operates as a standalone system, limiting integration with other software.
Conclusion
The Android Patient Tracker Project is an invaluable asset for modern healthcare management. Although it has some limitations, its advantages overwhelmingly make it an essential tool for doctors seeking to manage their patient data effectively.
For those interested in getting hands-on, the Android Patient Tracker Project Source Code is readily available, enabling healthcare providers to tailor the system according to their needs.
Sample Code
MainActivity.java
package com.example.patienttracker;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
private Button addPatientBtn, viewPatientsBtn;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
addPatientBtn = findViewById(R.id.btnAddPatient);
viewPatientsBtn = findViewById(R.id.btnViewPatients);
addPatientBtn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
startActivity(new Intent(MainActivity.this, AddPatientActivity.class));
}
});
viewPatientsBtn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
startActivity(new Intent(MainActivity.this, ViewPatientsActivity.class));
}
});
}
}
AddPatientActivity.java
package com.example.patienttracker;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import androidx.appcompat.app.AppCompatActivity;
public class AddPatientActivity extends AppCompatActivity {
private EditText nameField, diseaseField, medicationField;
private Button saveBtn;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_add_patient);
nameField = findViewById(R.id.edtName);
diseaseField = findViewById(R.id.edtDisease);
medicationField = findViewById(R.id.edtMedication);
saveBtn = findViewById(R.id.btnSave);
saveBtn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Insert the data into the SQLite database here
// Example:
// DatabaseHelper dbHelper = new DatabaseHelper(AddPatientActivity.this);
// dbHelper.addPatient(nameField.getText().toString(),
// diseaseField.getText().toString(), medicationField.getText().toString());
}
});
}
}
ViewPatientsActivity.java
package com.example.patienttracker;
import android.os.Bundle;
import android.widget.ListView;
import androidx.appcompat.app.AppCompatActivity;
public class ViewPatientsActivity extends AppCompatActivity {
private ListView patientsListView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_view_patients);
patientsListView = findViewById(R.id.patientsListView);
// Load the data from the SQLite database and populate the ListView here
// Example:
// DatabaseHelper dbHelper = new DatabaseHelper(ViewPatientsActivity.this);
// List<Patient> patients = dbHelper.getAllPatients();
// ArrayAdapter<Patient> adapter = new ArrayAdapter<>(this, android.R.layout.simple_list_item_1, patients);
// patientsListView.setAdapter(adapter);
}
}
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Android Patient Tracker Project PDF
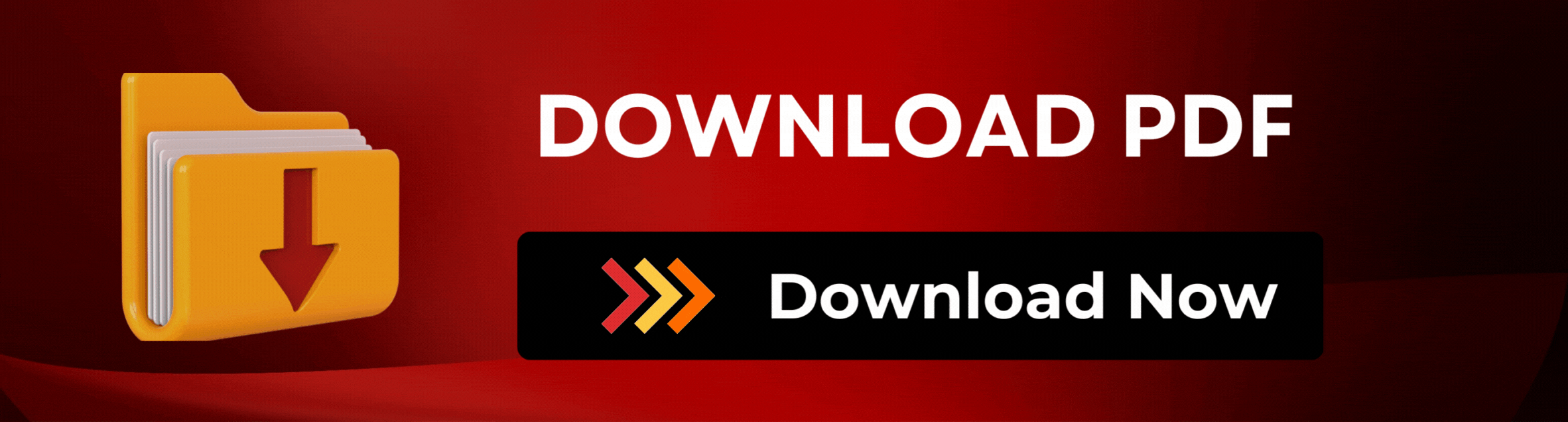