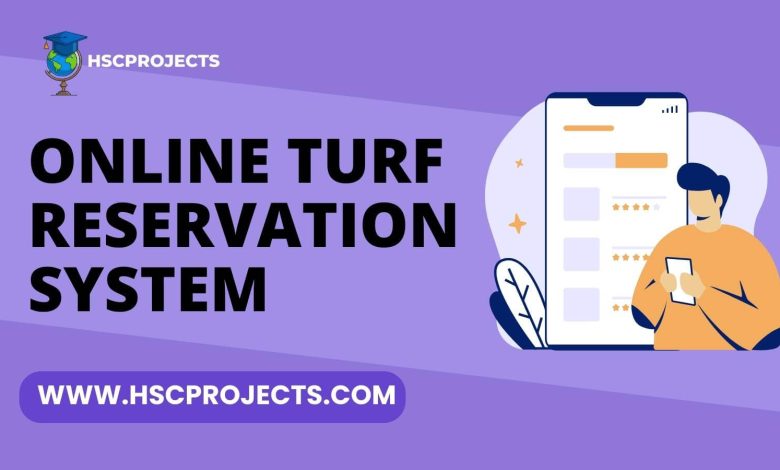
Online Turf Reservation System
Introduction
Sports enthusiasts know the importance of a good turf for activities like football, rugby, tennis, and cricket. However, securing a slot at your preferred turf can be a challenge, especially in high-demand areas like Mumbai. Enter the world of online turf booking, a digital solution that simplifies the reservation process, making it easier for individuals and teams to focus on the game rather than logistics.
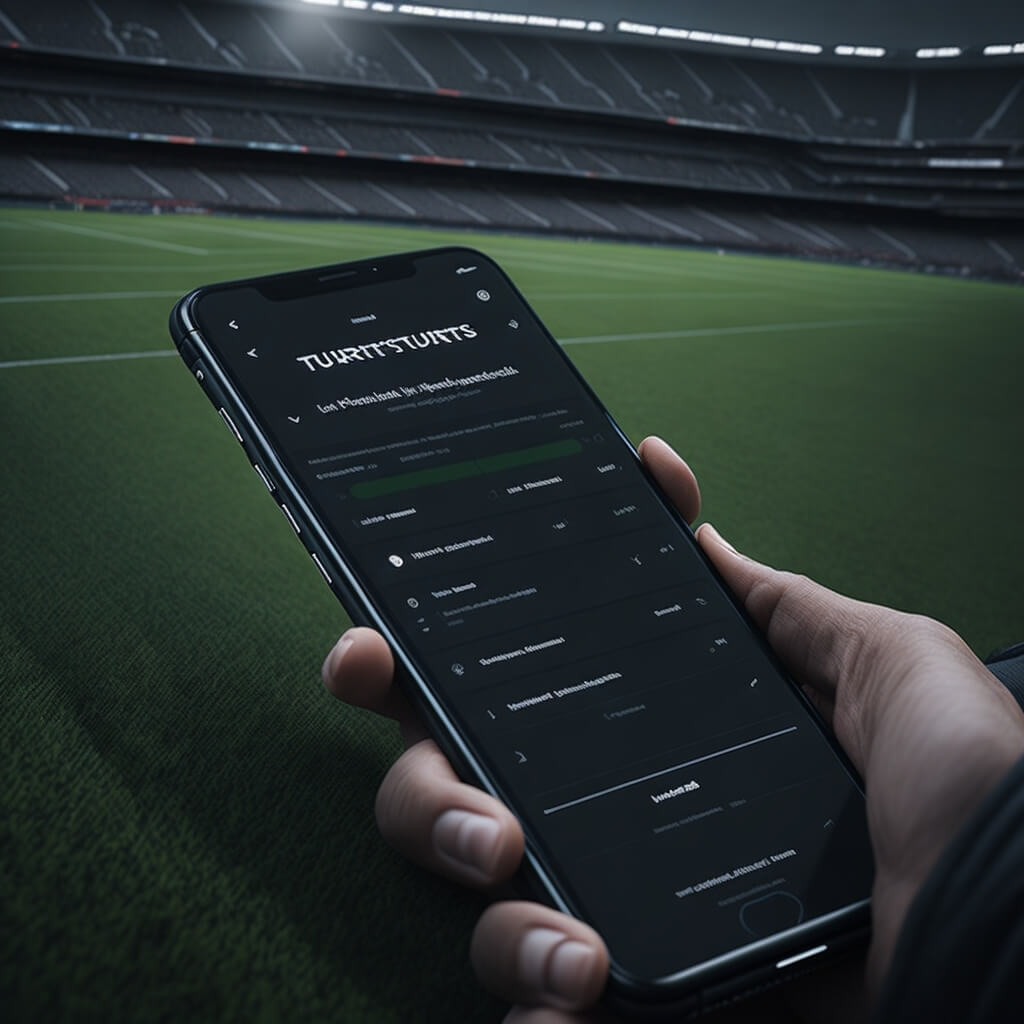
The Need for Online Turf Booking
Traditional methods of booking a sports turf often involve multiple phone calls, visits, and a lot of uncertainty. Online turf booking systems eliminate these hassles, offering a streamlined process that saves both time and effort.
How It Works
The online sports turf booking system comprises three main modules:
- Admin: Responsible for adding new turf locations, setting prices, and assigning managers to specific turfs.
- Manager: Manages specific turf locations, accepts bookings, generates bills, and maintains a history of all transactions.
- User: Can check turf availability, book slots, make payments, and view their booking history.
Features
- Real-time availability checks
- Secure payment options
- User-friendly interface
- Detailed booking history for users and managers
- Rate viewing and bill generation by managers
Advantages
- Convenience of booking from the comfort of your home
- Real-time availability and instant confirmation
- Secure and multiple payment options
- Transparency in pricing and billing
Limitations
- Requires an active internet connection for booking
- Limited to areas where the service is available
Popular Turf Booking Apps
- TurfBookingMumbai
- SportsBookingApp
- GroundBookingMaster
Conclusion
Online turf booking systems are a game-changer in the world of sports and recreation. They offer an efficient, transparent, and user-friendly way to secure your preferred playing ground, allowing you to focus more on the game and less on the booking process.
Sample Code
First, install Flask if you haven’t already:
pip install Flask
Create a new Python file (app.py
):
from flask import Flask, render_template, request, redirect, url_for
app = Flask(__name__)
# Dummy data for demonstration
turfs = [
{'id': 1, 'name': 'Turf A', 'location': 'Mumbai', 'availability': True},
{'id': 2, 'name': 'Turf B', 'location': 'Mumbai', 'availability': False},
{'id': 3, 'name': 'Turf C', 'location': 'Mumbai', 'availability': True},
]
@app.route('/')
def index():
return render_template('index.html', turfs=turfs)
@app.route('/book/<int:turf_id>', methods=['POST'])
def book_turf(turf_id):
turf = next((item for item in turfs if item['id'] == turf_id), None)
if turf and turf['availability']:
turf['availability'] = False
return redirect(url_for('index'))
else:
return "Turf not available", 400
if __name__ == '__main__':
app.run(debug=True)
Create an HTML file (templates/index.html
):
<!DOCTYPE html>
<html>
<head>
<title>Turf Booking</title>
</head>
<body>
<h1>Online Sports Turf Booking</h1>
<table border="1">
<tr>
<th>ID</th>
<th>Name</th>
<th>Location</th>
<th>Availability</th>
<th>Action</th>
</tr>
{% for turf in turfs %}
<tr>
<td>{{ turf.id }}</td>
<td>{{ turf.name }}</td>
<td>{{ turf.location }}</td>
<td>{{ 'Available' if turf.availability else 'Not Available' }}</td>
<td>
{% if turf.availability %}
<form action="{{ url_for('book_turf', turf_id=turf.id) }}" method="post">
<button type="submit">Book</button>
</form>
{% endif %}
</td>
</tr>
{% endfor %}
</table>
</body>
</html>
Run the Flask app:
python app.py
Visit http://127.0.0.1:5000/
in your web browser. You should see a list of turfs with their availability status and a “Book” button for available turfs.
This is a very basic example and lacks many features like user authentication, database storage, and front-end interactivity. For a production application, you would likely use a database to store turf and booking information and add more features like user accounts, payment processing, etc.
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Online Turf Reservation System PDF
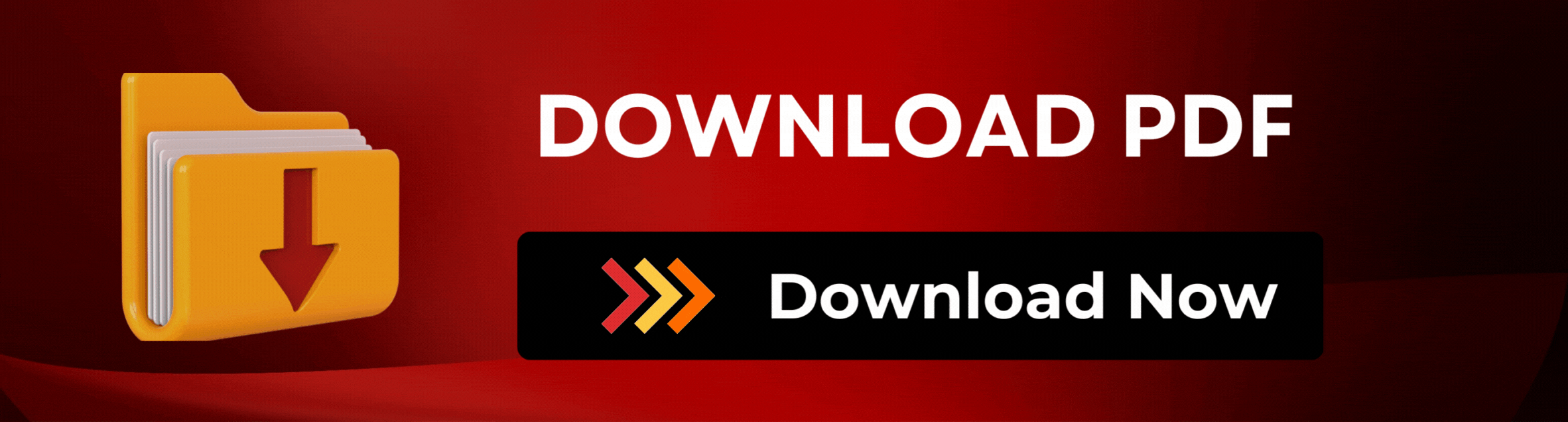