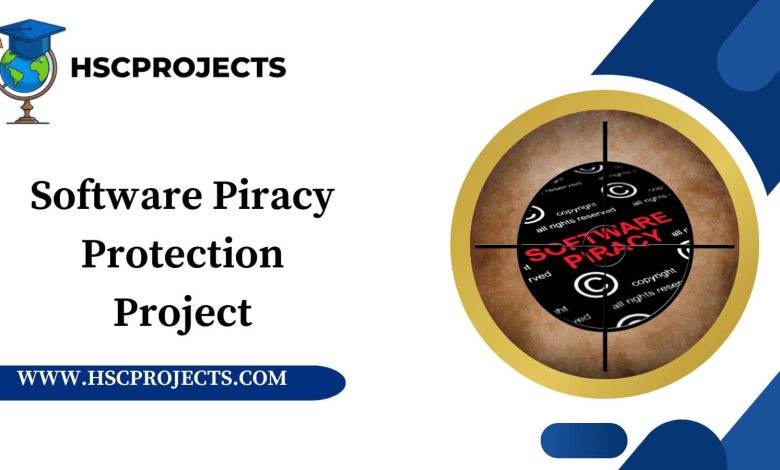
Software Piracy Protection Project
The Software Piracy Protection Project represents a critical initiative in the fight against software piracy, a prevalent issue that significantly impacts the software industry. This project is designed to ensure that only authenticated users can access software, thereby maintaining the integrity and ownership of digital products.
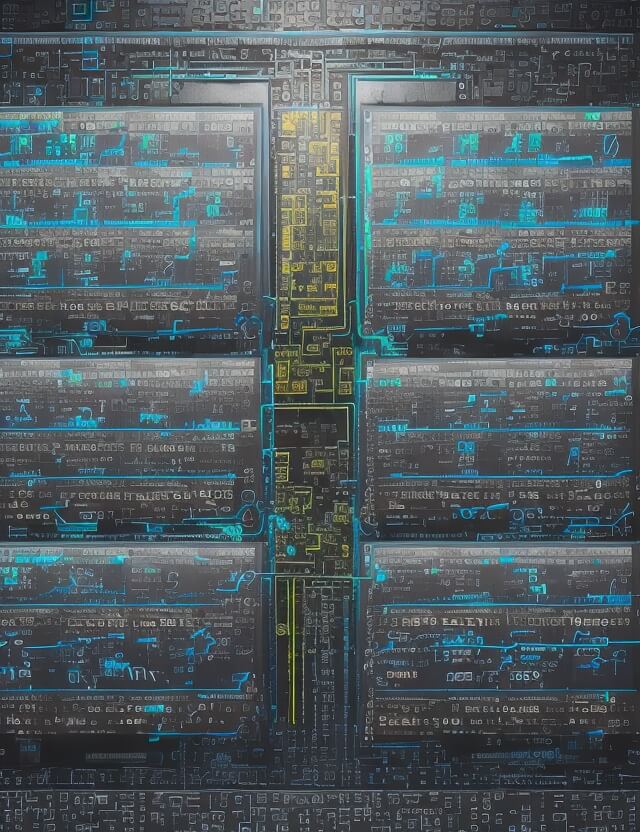
Understanding Software Piracy
Software piracy refers to the unauthorized copying, distribution, or use of software. It includes activities such as producing counterfeit software, distributing software illegally, or using software without proper licenses. This not only harms the software developers by depriving them of rightful earnings but also exposes users to security risks like malware and subpar software performance.
Features of the Software Piracy Protection Project
- Online Registration: Users must register within the system, ensuring that only verified individuals can access the software.
- Secure Payment for Software: Access to the software is granted after a secure online payment, guaranteeing transactions are safe and legitimate.
- Unique Serial Key with Download: Post-payment, users can download the software along with a unique serial key for later authentication.
- PC ID Reader & Product ID Generation: The system reads the user’s PC MAC address and generates a unique user ID using a specific algorithm.
- Key Generation and Authentication: Users request a serial key by sending their unique user ID. An encrypted key is then generated and provided for authentication.
- Data Matching and Authentication Process: The software compares the user-provided key with the system-generated key for authentication. If the keys match, the software functions fully; otherwise, it remains locked.
Advantages of Implementing the System
- Protection of Copyright: Ensures that software ownership is respected and prevents unauthorized distribution.
- Unique Activation Codes: Each software copy requires a unique activation code, preventing unauthorized copying and sharing.
- Robust Security: The system is designed to be secure against hacking and unauthorized access, protecting both the software and the user.
Limitations of the Current Approach
- Time-Consuming Process: The requirement for admin approval and matching details can delay the activation process.
- Device Dependency: The system’s reliance on MAC IDs means the software cannot be easily transferred between devices.
Sample Code
import uuid
import hashlib
def generate_unique_id():
# Generate a unique ID using the MAC address and current time
unique_id = uuid.uuid1() # This simulates generating a unique user/product ID
return unique_id
def generate_serial_key(unique_id):
# Create a serial key using a hashing algorithm
serial_key = hashlib.sha256(unique_id.bytes).hexdigest()
return serial_key[:16] # Truncate key for simplicity
if __name__ == "__main__":
unique_id = generate_unique_id()
print("Unique ID:", unique_id)
serial_key = generate_serial_key(unique_id)
print("Serial Key:", serial_key)
import KeyGen
def verify_serial_key(user_provided_key, unique_id):
# Regenerate the serial key from the unique ID
correct_key = KeyGen.generate_serial_key(unique_id)
# Compare the provided key with the correct key
if user_provided_key == correct_key:
return True
else:
return False
if __name__ == "__main__":
# Simulate user entering serial key
user_provided_key = input("Enter your serial key: ")
# Simulate retrieving the unique ID (in reality, this would be securely stored and retrieved)
unique_id = KeyGen.generate_unique_id()
# Verify the serial key
if verify_serial_key(user_provided_key, unique_id):
print("Serial key is valid. Software activated.")
else:
print("Invalid serial key. Activation failed.")
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Software Piracy Protection Project PDF
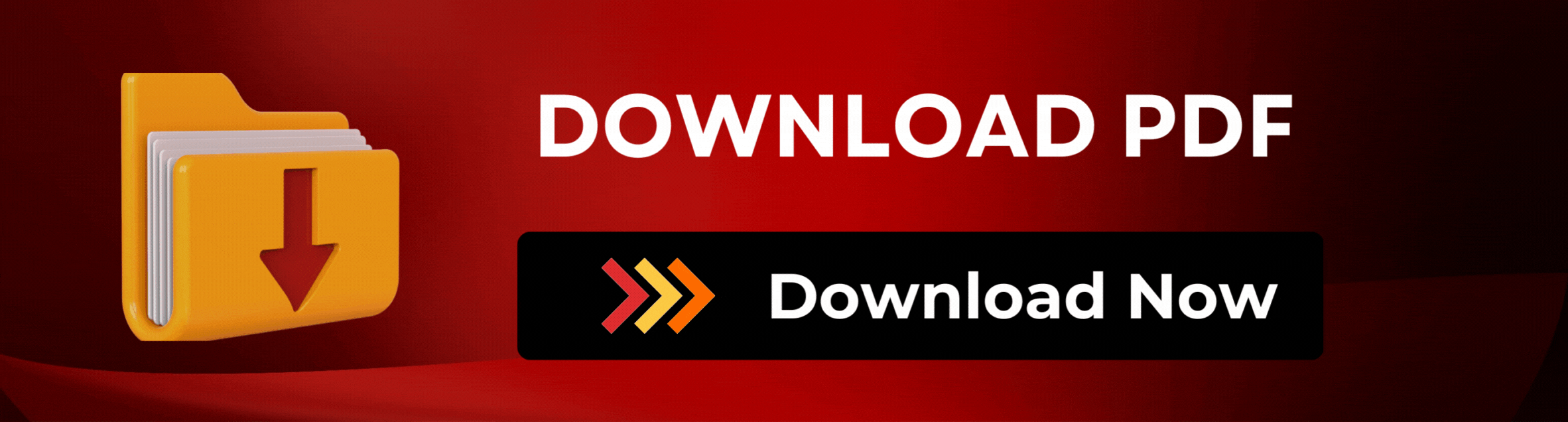