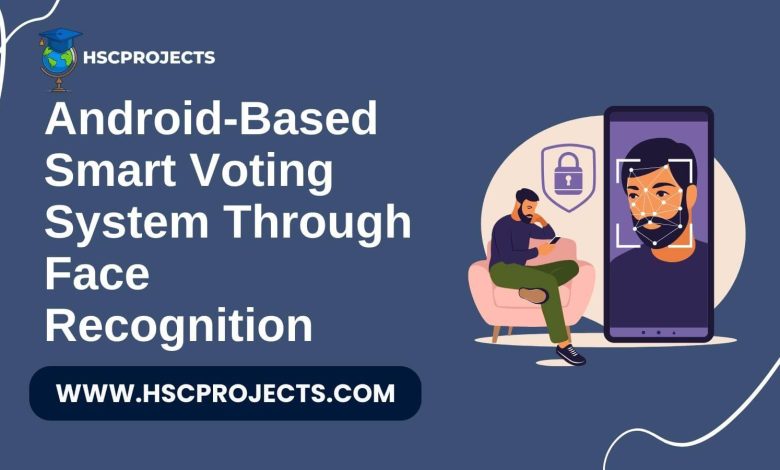
Android-Based Smart Voting System Through Face Recognition
Introduction
The digital age has brought about many changes, and voting systems are no exception. While traditional methods have their merits, the demand for a more secure and convenient voting process has never been higher. Enter the Android-based smart voting system through face recognition—a groundbreaking approach to modernizing democracy.

How It Works
The system is built on Android and utilizes Microsoft Cognitive Services Face API for face recognition. This technology not only verifies the identity of voters but also ensures the security of the voting process. The system comprises two modules: Admin and User.
Admin Module:
- Approves or disapproves user accounts
- Creates public or private polls
- Monitors voting activity and results
User Module:
- Registers and manages profiles
- Participates in public or private polls
- Reviews past voting activity and results
Features and Advantages
- Ease of Use: The system is user-friendly, allowing voters to participate from the comfort of their homes.
- Security: Face recognition technology ensures the authenticity of votes and voters.
- Private Polls: Admins can create private polls accessible only through a security code.
- Transparency: Both admins and users can view detailed voting results, enhancing the system’s transparency.
Technical Aspects
The system leverages Azure Face service, which provides AI algorithms that detect, recognize, and analyze human faces in images. This ensures a high level of accuracy in voter identification.
Conclusion
The Android-based smart voting system through face recognition is a significant step forward in making voting more secure, transparent, and convenient. It holds the potential to increase voter turnout and provide more accurate results compared to traditional methods.
Sample Code
First, add the following dependencies to your ‘build.gradle
‘
implementation 'com.microsoft.projectoxford:face:1.4.0'
implementation 'com.google.code.gson:gson:2.8.6'
import android.graphics.Bitmap;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.ImageView;
import androidx.appcompat.app.AppCompatActivity;
import com.microsoft.projectoxford.face.FaceServiceClient;
import com.microsoft.projectoxford.face.FaceServiceRestClient;
import com.microsoft.projectoxford.face.contract.Face;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.InputStream;
public class MainActivity extends AppCompatActivity {
private final String apiEndpoint = "YOUR_API_ENDPOINT";
private final String subscriptionKey = "YOUR_SUBSCRIPTION_KEY";
private final FaceServiceClient faceServiceClient = new FaceServiceRestClient(apiEndpoint, subscriptionKey);
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
final Button btnCapture = findViewById(R.id.btnCapture);
final ImageView imgView = findViewById(R.id.imgView);
// Assume you have a Bitmap image (imgBitmap) captured from the camera or loaded from storage
final Bitmap imgBitmap = /* your bitmap image */;
imgView.setImageBitmap(imgBitmap);
btnCapture.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
imgBitmap.compress(Bitmap.CompressFormat.JPEG, 100, outputStream);
ByteArrayInputStream inputStream = new ByteArrayInputStream(outputStream.toByteArray());
new Thread(new Runnable() {
public void run() {
try {
Face[] result = faceServiceClient.detect(inputStream, true, false, null);
if (result.length == 0) {
Log.d("RESULT", "No face detected");
} else {
Log.d("RESULT", "Face detected: " + result[0].faceId);
}
} catch (Exception e) {
Log.d("ERROR", e.getMessage());
}
}
}).start();
}
});
}
}
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Subscribed? Click on Confirm
Download Android-Based Smart Voting System Through Face Recognition PDF
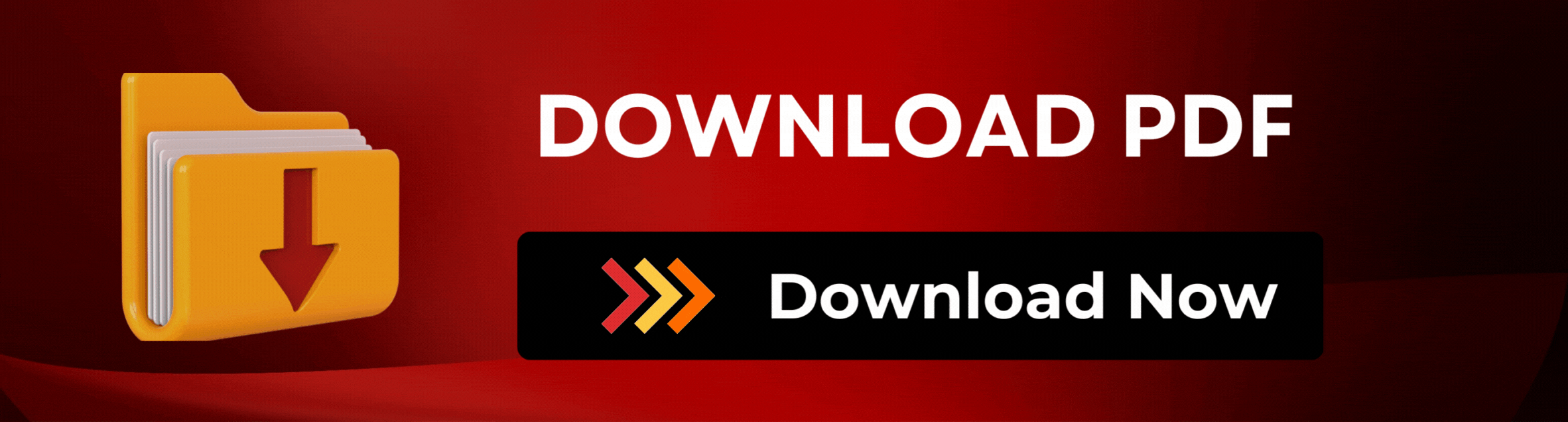