
Advanced Android Step Counter App
Introduction
In a world where health consciousness is rising, keeping track of physical activity is more important than ever. The Android Step Counter App is designed to be your health companion, offering precise step counting, sleep monitoring, and water intake recommendations tailored to your personal health metrics.
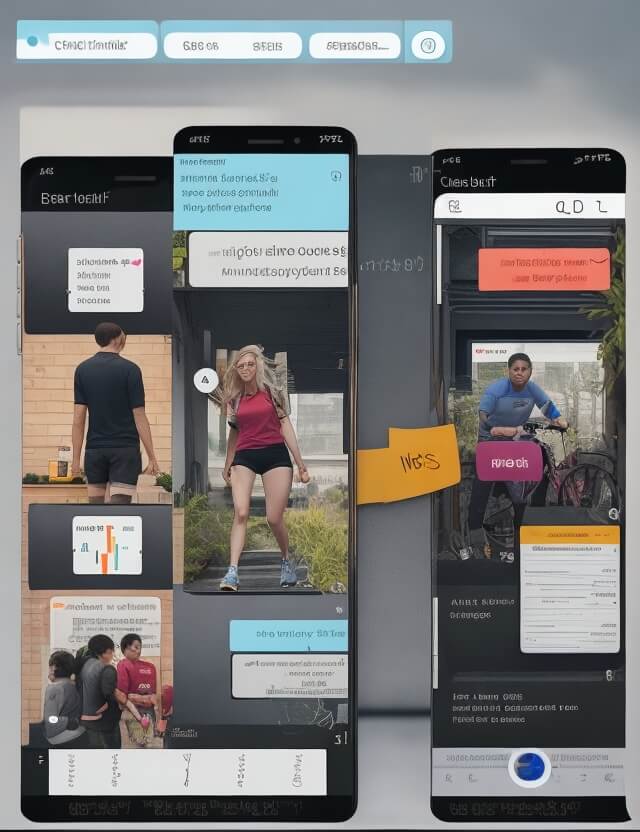
How the App Works
- Step Counting: Utilizing the smartphone’s built-in accelerometer, the app accurately counts your steps as you move throughout the day.
- Personalized Health Metrics: Input your age, height, weight, and gender to calculate your Body Mass Index (BMI) and tailor daily step, sleep, and water intake goals.
- Graphical Representations: Visualize your progress with daily and historical data graphs, motivating you to reach your fitness goals.
Key Features
- Real-Time Step Tracking: Monitors every step you take, providing instant feedback on your daily activity levels.
- Sleep and Hydration Monitoring: In addition to step count, the app calculates and suggests optimal sleep duration and water intake based on your personal health data.
- Data Visualization: Offers a graphical representation of your step count history, allowing you to analyze trends and improvements over time.
Advantages
- Health Monitoring: Encourages a healthy lifestyle by tracking physical activity along with sleep and hydration.
- User-Friendly Interface: Intuitive and easy-to-use, ensuring a seamless experience for all users.
- Motivational Tool: Sets personal goals and visually tracks progress, providing a motivational boost to users.
Limitations
- Accuracy Concern: The step count may vary depending on the sensitivity and specifications of the accelerometer sensor.
- Data Persistence: Local data storage means that if the app is uninstalled, all historical data will be lost unless backed up externally.
Conclusion
The Android Step Counter App is more than just a pedometer; it’s a comprehensive health monitoring system that fits right into your pocket. By combining user-friendly design with powerful tracking and analysis tools, it empowers users to take charge of their health and stride confidently towards their fitness goals.
Sample Code
Components Needed:
- Android Studio and SDK
- A physical Android device or emulator with an accelerometer sensor
Permissions and Dependencies:
<uses-permission android:name="android.permission.ACTIVITY_RECOGNITION"/>
import android.app.Activity;
import android.hardware.Sensor;
import android.hardware.SensorEvent;
import android.hardware.SensorEventListener;
import android.hardware.SensorManager;
import android.os.Bundle;
import android.widget.TextView;
public class MainActivity extends Activity implements SensorEventListener {
private TextView count;
boolean activityRunning;
private SensorManager sensorManager;
private Sensor stepSensor;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
count = (TextView) findViewById(R.id.count);
sensorManager = (SensorManager) getSystemService(Context.SENSOR_SERVICE);
if (sensorManager.getDefaultSensor(Sensor.TYPE_STEP_COUNTER) != null) {
stepSensor = sensorManager.getDefaultSensor(Sensor.TYPE_STEP_COUNTER);
}
}
@Override
protected void onResume() {
super.onResume();
activityRunning = true;
sensorManager.registerListener(this, stepSensor, SensorManager.SENSOR_DELAY_UI);
}
@Override
protected void onPause() {
super.onPause();
activityRunning = false;
sensorManager.unregisterListener(this);
}
@Override
public void onSensorChanged(SensorEvent event) {
if (activityRunning) {
count.setText(String.valueOf(event.values[0]));
}
}
@Override
public void onAccuracyChanged(Sensor sensor, int accuracy) {
}
}
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="16dp">
<TextView
android:id="@+id/count"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Steps: 0"
android:textSize="30sp" />
</RelativeLayout>
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Advanced Android Step Counter App PDF
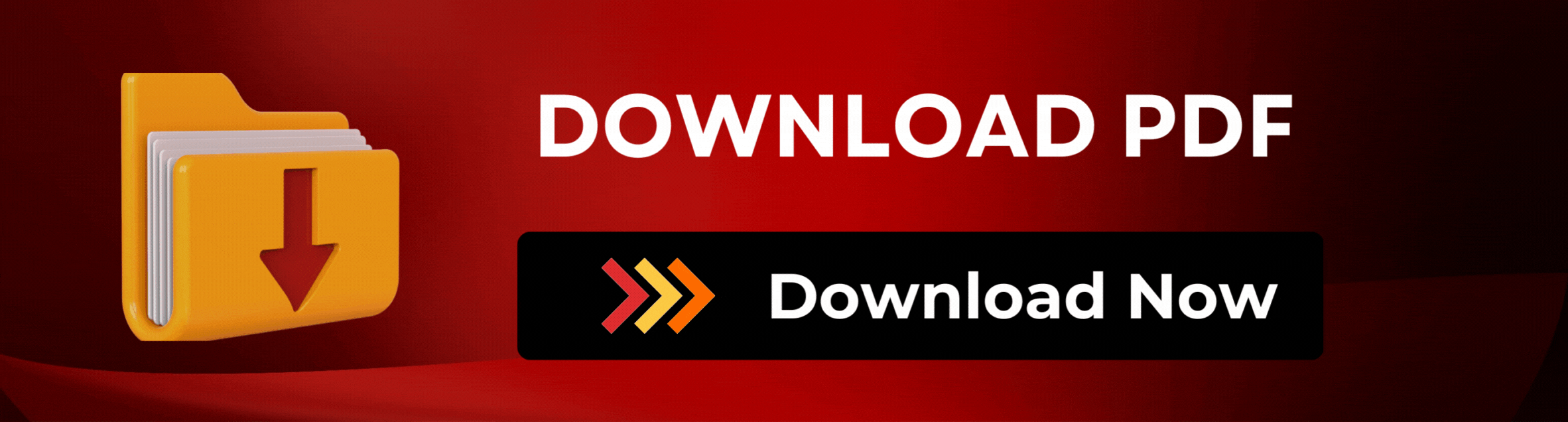