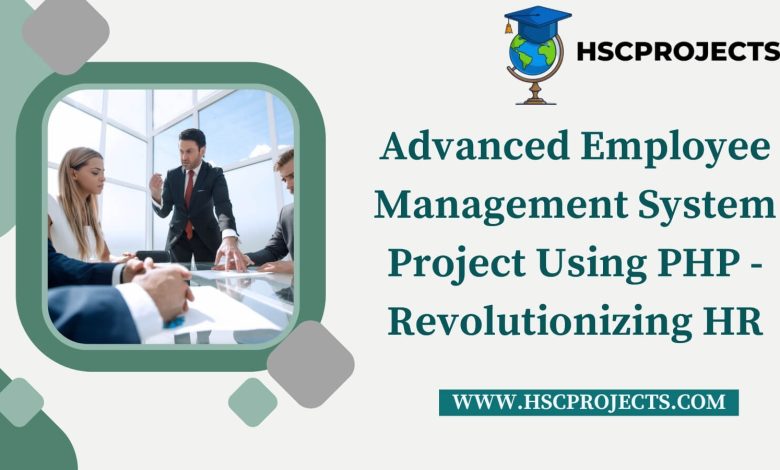
Advanced Employee Management System Project Using PHP – Revolutionizing HR
Introduction
Managing human resources is a complex task that involves various responsibilities, including employee management, leave management, and payroll processing. Traditional methods can be cumbersome and inefficient, especially for larger organizations. This is where an advanced Employee Management System Project in PHP comes into play. This comprehensive system not only simplifies HR tasks but also adds a layer of efficiency and accuracy.
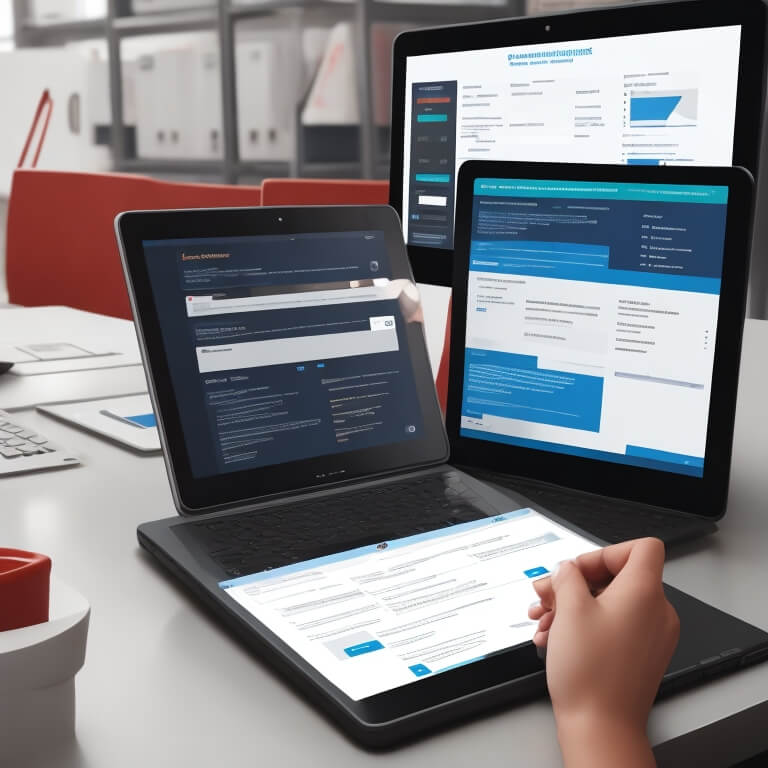
System Entities
The system primarily consists of two entities: Admin and Employee.
- Admin: The admin has the authority to add company information, manage employee details, oversee leaves, manage payroll, and add holidays.
- Employee: Employees can check their leave status, view salary details, see the list of holidays, and even submit their resignation if needed.
Features
- Real-Time Employee Data: The system provides real-time data on employees, making it easier for HR to make informed decisions.
- Leave Management: With a few clicks, both employees and HR can manage leaves efficiently, reducing paperwork and manual errors.
- Payroll Management: The system automates the complex task of payroll management, ensuring that employees are paid accurately and on time.
- Holiday Management: Admin can easily add or modify the list of official holidays, which can be viewed by all employees.
- Document Management: Important documents like employment contracts and confidentiality agreements can be securely stored and easily accessed.
Advantages
- Efficiency: The system significantly reduces the time spent on HR tasks, allowing the department to focus on more strategic activities.
- Accuracy: Automated calculations and data updates minimize the risk of errors.
- Accessibility: Being a web-based system, it can be accessed from anywhere, offering flexibility to both employees and HR staff.
Disadvantages
- Internet Dependency: The system requires an active internet connection, which could be a limitation in areas with poor connectivity.
- Data Integrity: The system is only as good as the data fed into it. Incorrect data entry can lead to errors in payroll or leave calculations.
Conclusion
An Advanced Employee Management System Project in PHP is not just a want but a need for companies aiming for operational excellence. It streamlines HR operations and offers a centralized platform for employee management.
Sample Code
First, let’s create a MySQL database named employee_management
.
CREATE DATABASE employee_management;
USE employee_management;
CREATE TABLE employees (
id INT PRIMARY KEY AUTO_INCREMENT,
name VARCHAR(50),
email VARCHAR(50),
position VARCHAR(50)
);
create the PHP files.
config.php
This file will contain the database connection details.
<?php
$servername = "localhost";
$username = "root";
$password = "";
$dbname = "employee_management";
$conn = new mysqli($servername, $username, $password, $dbname);
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
?>
admin.php
This file will contain the form for the admin to add an employee.
<?php include 'config.php'; ?>
<!DOCTYPE html>
<html>
<head>
<title>Admin Dashboard</title>
</head>
<body>
<h1>Add Employee</h1>
<form action="admin.php" method="post">
Name: <input type="text" name="name"><br>
Email: <input type="email" name="email"><br>
Position: <input type="text" name="position"><br>
<input type="submit" name="add_employee" value="Add Employee">
</form>
<?php
if (isset($_POST['add_employee'])) {
$name = $_POST['name'];
$email = $_POST['email'];
$position = $_POST['position'];
$sql = "INSERT INTO employees (name, email, position) VALUES ('$name', '$email', '$position')";
if ($conn->query($sql) === TRUE) {
echo "New employee added successfully";
} else {
echo "Error: " . $sql . "<br>" . $conn->error;
}
}
?>
</body>
</html>
employee.php
This file will display the list of employees.
<?php include 'config.php'; ?>
<!DOCTYPE html>
<html>
<head>
<title>Employee Dashboard</title>
</head>
<body>
<h1>Employee List</h1>
<?php
$sql = "SELECT id, name, email, position FROM employees";
$result = $conn->query($sql);
if ($result->num_rows > 0) {
while($row = $result->fetch_assoc()) {
echo "ID: " . $row["id"]. " - Name: " . $row["name"]. " - Email: " . $row["email"]. " - Position: " . $row["position"]. "<br>";
}
} else {
echo "0 results";
}
$conn->close();
?>
</body>
</html>
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Advanced Employee Management System Project Using PHP – Revolutionizing HR PDF
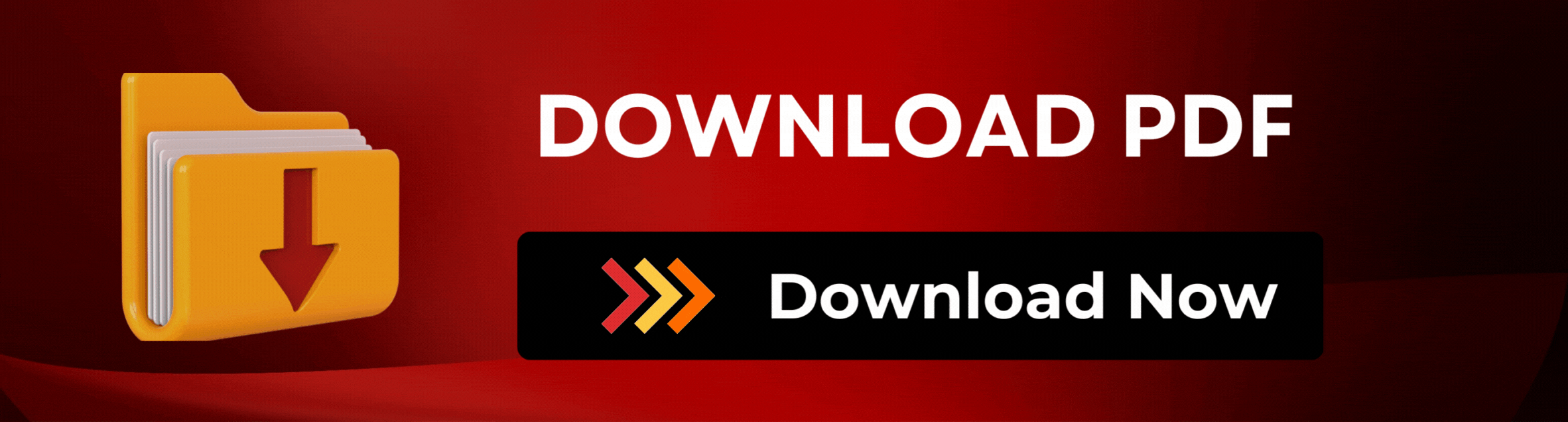