
Engineering ProjectsBsc-ITDiplomaIT ProjectsMsc-IT Projects
Advanced Warehouse Management System
Introduction
The Advanced Warehouse Management System (WMS) is an innovative real-time database solution designed to efficiently manage large inventories for organizations. From single stores to multiple warehouses, this system is scalable to meet the needs of any size business, ensuring a consistent supply of products and materials.
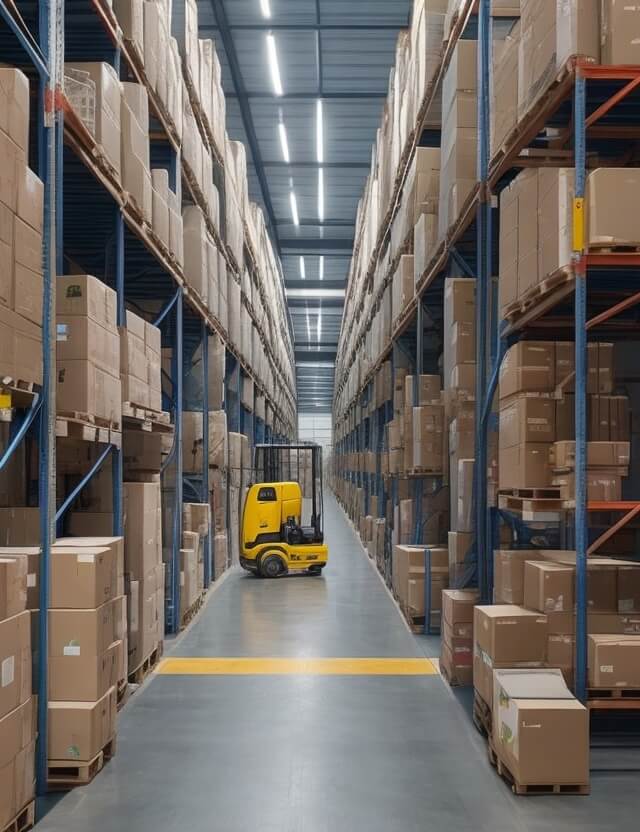
System Overview
- Capabilities: The WMS tracks sales, restocking data, and provides low-stock notifications. It supports the distribution of stock among various locations within a franchise.
- Design: Aimed at small to large store owners and stock managers, the system focuses on maintaining sufficient goods on hand in both retail and manufacturing settings. It can operate as a standalone desktop application or scale to a networked environment across multiple locations.
- Functionality: Besides tracking In & Out transactions and generating billing details, it produces comprehensive monthly sales reports, enabling managers to understand and analyze transaction trends.
Advantages
- Efficiency: The system increases operational efficiency, making information input and inventory tracking simpler and more accurate.
- Cost Reduction: With efficient management, the system helps in lowering operational and inventory costs.
- Reporting: Capable of generating detailed monthly reports and billing for every transaction, aiding in strategic planning and accountability.
Disadvantages
- Data Accuracy: The effectiveness of the WMS depends on the accuracy of data entry, requiring diligent monitoring and verification.
- Complexity: Managing the various functions of inventory can be complex and requires a shared responsibility approach among team members.
Conclusion
The Advanced Warehouse Management System is a dynamic and essential tool for any business seeking to improve its inventory and godown management. By automating and streamlining processes, it ensures a smooth flow of operations, from stocking to distribution, while minimizing costs and maximizing efficiency.
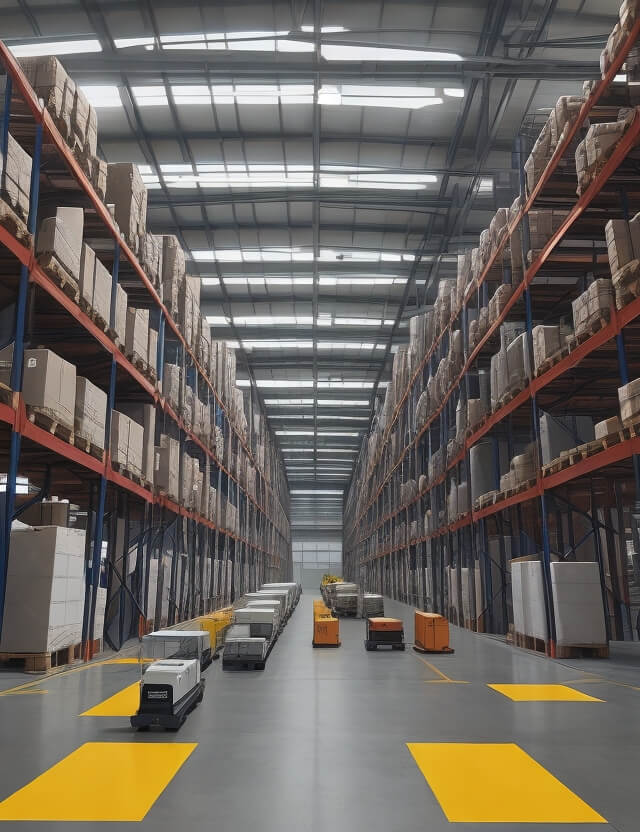
Sample Code
import java.util.HashMap;
import java.util.Map;
// Class representing an item in the warehouse
class Item {
String id;
String name;
double price;
public Item(String id, String name, double price) {
this.id = id;
this.name = name;
this.price = price;
}
// Getters and setters not shown for brevity
}
// Main Warehouse Management System class
public class WarehouseManagementSystem {
// Inventory map to hold items and quantities
private Map<Item, Integer> inventory;
public WarehouseManagementSystem() {
this.inventory = new HashMap<>();
}
// Add items to the inventory
public void addItem(Item item, int quantity) {
inventory.put(item, inventory.getOrDefault(item, 0) + quantity);
}
// Remove items from the inventory
public boolean removeItem(Item item, int quantity) {
if (!inventory.containsKey(item) || inventory.get(item) < quantity) {
System.out.println("Not enough inventory or item does not exist.");
return false;
}
inventory.put(item, inventory.get(item) - quantity);
return true;
}
// Display inventory
public void displayInventory() {
System.out.println("Inventory:");
for (Map.Entry<Item, Integer> entry : inventory.entrySet()) {
Item item = entry.getKey();
Integer quantity = entry.getValue();
System.out.println("Item ID: " + item.id + ", Name: " + item.name + ", Quantity: " + quantity);
}
}
// Main method for demonstration
public static void main(String[] args) {
WarehouseManagementSystem wms = new WarehouseManagementSystem();
// Adding some items
wms.addItem(new Item("1", "Widget", 19.99), 150);
wms.addItem(new Item("2", "Gadget", 29.99), 90);
// Displaying current inventory
wms.displayInventory();
// Removing some items
wms.removeItem(new Item("1", "Widget", 19.99), 50);
// Displaying inventory after removal
wms.displayInventory();
}
}
Click to rate this post!
[Total: 0 Average: 0]
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Advanced Warehouse Management System PDF
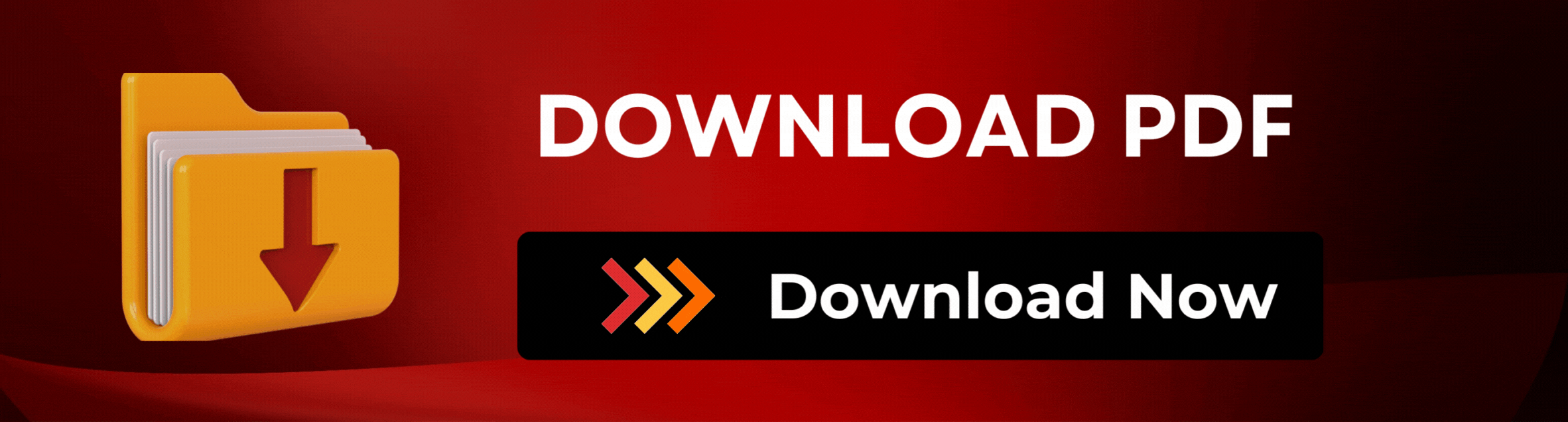