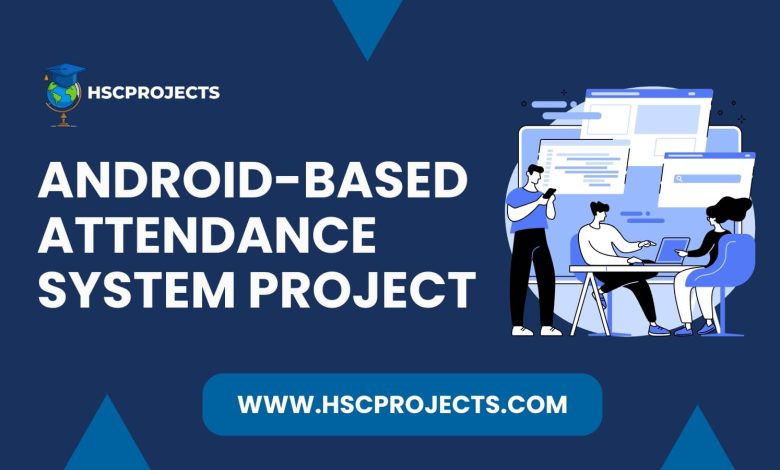
Android Based Attendance System Project
Introduction
Attendance tracking in educational institutions has traditionally been a manual and time-consuming task. However, the advent of Android-based attendance management systems is transforming this crucial aspect of classroom management. This article delves into the features, benefits, and limitations of using an attendance management app on Android devices.
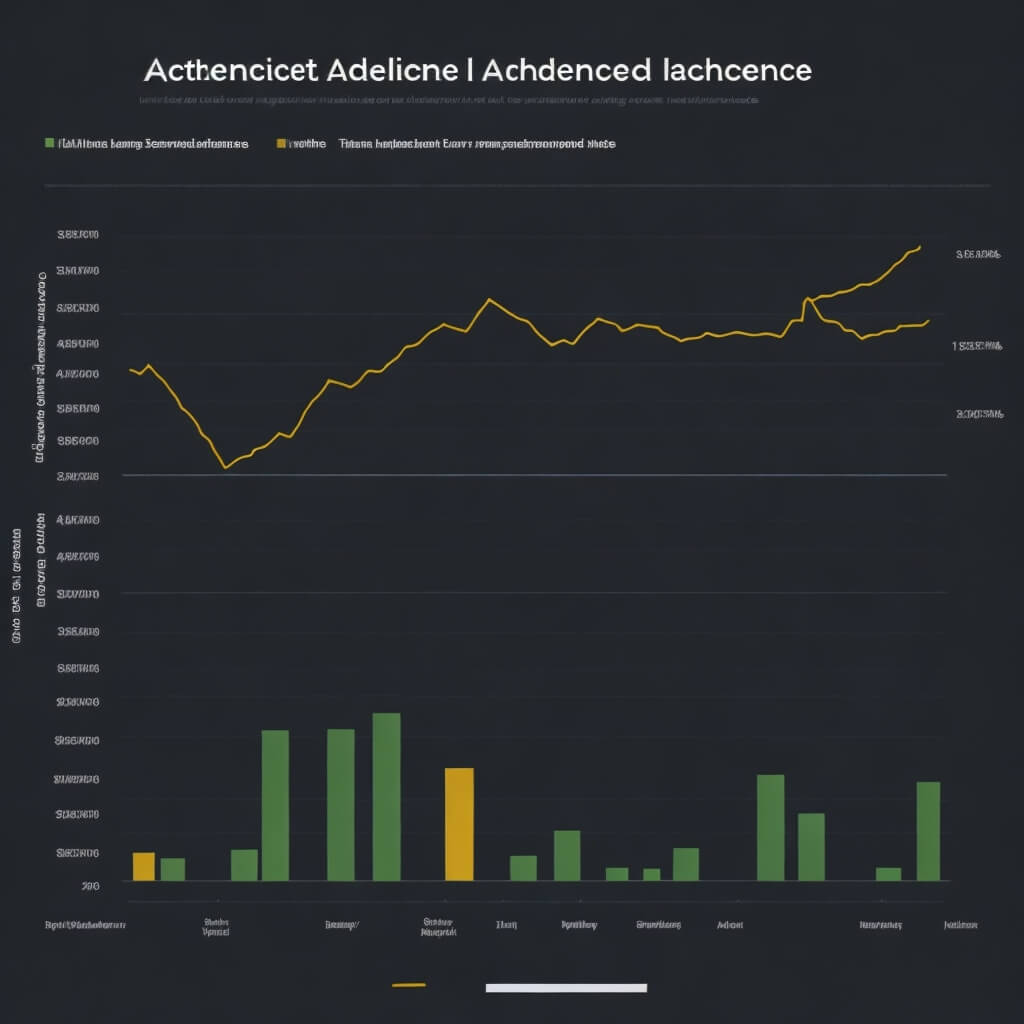
Key Features
- Student Attendance List Creation: The app allows faculty to create a digital attendance sheet, including student names, roll numbers, and subjects.
- Attendance Marking: Faculty can easily mark students as present or absent directly through the app.
- Attendance Storage: All attendance data is securely stored on the faculty’s Android device for easy access and review.
- Attendance Sheet Transfer: Faculty can transfer the attendance data to a server or computer via Bluetooth, allowing for centralized storage and analysis.
Advantages
- Paperless System: The Android-based system eliminates the need for physical paperwork, making it an eco-friendly option.
- Ease of Transfer: The Bluetooth feature allows for easy transfer of attendance data to a central server.
- Class Performance Metrics: The app provides insights into overall class attendance, aiding in performance evaluations.
- Resource Efficiency: The app runs on Android mobile devices, eliminating the need for a computer or laptop in every classroom.
Limitations
- Platform Dependency: The system is limited to Android devices. However, given the widespread use of Android smartphones, this is generally not a significant issue.
Conclusion
The Android-based attendance management system offers a streamlined, efficient, and eco-friendly solution for tracking student attendance. While it does have some limitations, such as platform dependency, the benefits far outweigh the drawbacks. Faculty can now focus more on teaching and less on administrative tasks, making it a win-win for both educators and students.
Sample Code
Add permissions for Bluetooth in your AndroidManifest.xml
:
<uses-permission android:name="android.permission.BLUETOOTH"/>
<uses-permission android:name="android.permission.BLUETOOTH_ADMIN"/>
MainActivity.java
import android.app.Activity;
import android.bluetooth.BluetoothAdapter;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.Toast;
public class MainActivity extends Activity {
private static final int REQUEST_ENABLE_BT = 1;
BluetoothAdapter bluetoothAdapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
bluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
Button markAttendanceButton = findViewById(R.id.markAttendanceButton);
if (bluetoothAdapter == null) {
Toast.makeText(getApplicationContext(), "Bluetooth not supported", Toast.LENGTH_LONG).show();
} else {
markAttendanceButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (!bluetoothAdapter.isEnabled()) {
Intent enableBtIntent = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE);
startActivityForResult(enableBtIntent, REQUEST_ENABLE_BT);
} else {
// Mark attendance logic here
}
}
});
}
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
if (requestCode == REQUEST_ENABLE_BT) {
if (resultCode == RESULT_OK) {
Toast.makeText(getApplicationContext(), "Bluetooth enabled", Toast.LENGTH_LONG).show();
} else {
Toast.makeText(getApplicationContext(), "Bluetooth not enabled", Toast.LENGTH_LONG).show();
}
}
}
}
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<Button
android:id="@+id/markAttendanceButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Mark Attendance" />
</RelativeLayout>
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Subscribed? Click on Confirm
Download Android Based Attendance System Project PDF
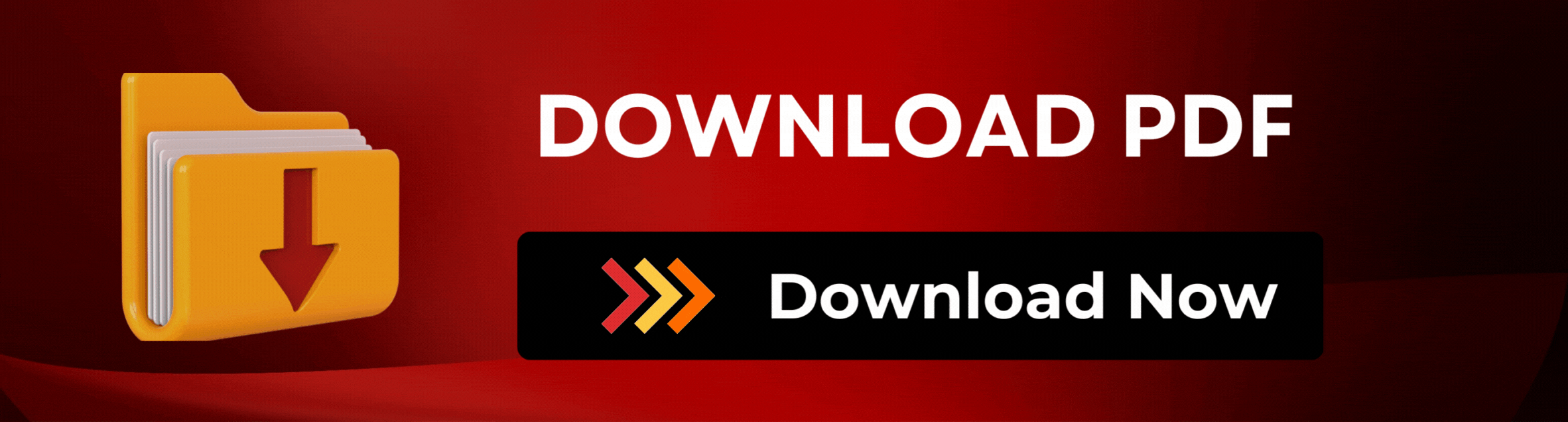