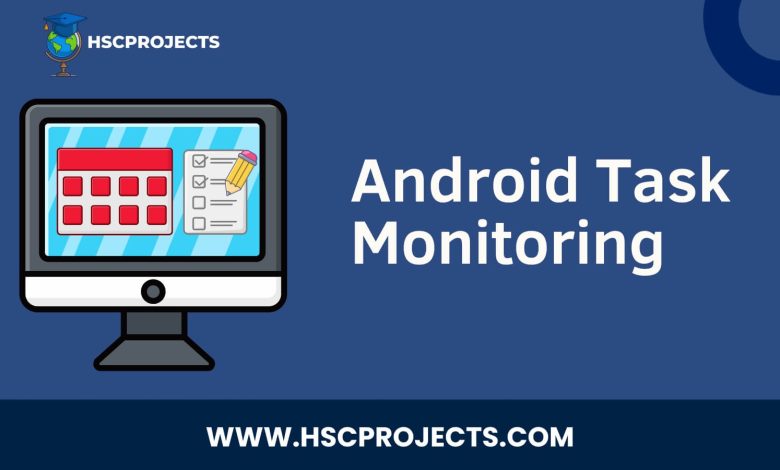
Android Task Monitoring
Introduction
In today’s fast-paced world, managing multiple tasks efficiently is more crucial than ever. Whether it’s attending meetings, paying bills, or taking medications, missing out on important tasks can disrupt your life. Enter Android Task Monitoring—a solution designed to keep you on top of your game. This article delves into the features, advantages, and limitations of Android Task Monitoring projects and apps.
The Need for Android Task Monitoring
The human mind is not built for multitasking; it performs best when focused on a single task. This is where Android Task Monitoring apps come into play. These apps serve as your digital assistant, sending you timely reminders so that you can focus on what truly matters.
AI-Powered Efficiency
The latest Android Task Monitoring apps are integrated with AI-powered chatbots. These bots not only remind you of your tasks but also engage with you to ensure you’re making the most of your day. They analyze your phone usage patterns to send notifications at times when you’re most likely to see them, thereby increasing the chances of task completion.

Key Features
- Real-Time Notifications: Receive instant alerts for upcoming tasks.
- User Preference-Based Reminders: Customize reminders based on your lifestyle and preferences.
- Auto-Answering Queries: The AI assistant can automatically answer frequently asked questions about your schedule.
Advantages
- Stay Informed: Never miss an important task with real-time notifications.
- Customization: Receive reminders that align with your personal preferences.
- AI Assistance: Benefit from an AI assistant that helps you become more productive.
Limitations
- Device Compatibility: These apps are primarily designed for Android devices.
- Internet Dependency: An active internet connection is required for optimal functionality.
- Data Accuracy: The effectiveness of the app depends on the accuracy of the data entered.
Conclusion
Android Task Monitoring is not just a trend; it’s a necessity for anyone looking to improve their productivity and time management. With features like AI-powered reminders and real-time notifications, these apps are the future of task management.
Sample Code
Firstly, add the following permissions to your ‘AndroidManifest.xml
‘:
<uses-permission android:name="android.permission.INTERNET"/>
<uses-permission android:name="android.permission.FOREGROUND_SERVICE"/>
Also, add the service and activity to your ‘AndroidManifest.xml
‘:
<service android:name=".NotificationService"/>
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
create the ‘NotificationService.java
‘:
import android.app.Notification;
import android.app.NotificationChannel;
import android.app.NotificationManager;
import android.app.Service;
import android.content.Intent;
import android.os.Build;
import android.os.IBinder;
import androidx.annotation.Nullable;
import androidx.core.app.NotificationCompat;
public class NotificationService extends Service {
@Override
public void onCreate() {
super.onCreate();
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
NotificationChannel channel = new NotificationChannel("taskChannel", "Task Notifications", NotificationManager.IMPORTANCE_DEFAULT);
NotificationManager manager = getSystemService(NotificationManager.class);
manager.createNotificationChannel(channel);
}
}
@Override
public int onStartCommand(Intent intent, int flags, int startId) {
Notification notification = new NotificationCompat.Builder(this, "taskChannel")
.setContentTitle("Task Alert")
.setContentText("You have a task to complete!")
.setSmallIcon(R.drawable.ic_launcher_foreground)
.build();
startForeground(1, notification);
// Stop the service as soon as the notification is sent
stopSelf();
return START_NOT_STICKY;
}
@Nullable
@Override
public IBinder onBind(Intent intent) {
return null;
}
}
MainActivity.java
:
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button notifyButton = findViewById(R.id.notifyButton);
notifyButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent serviceIntent = new Intent(MainActivity.this, NotificationService.class);
startService(serviceIntent);
}
});
}
}
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Android Task Monitoring PDF
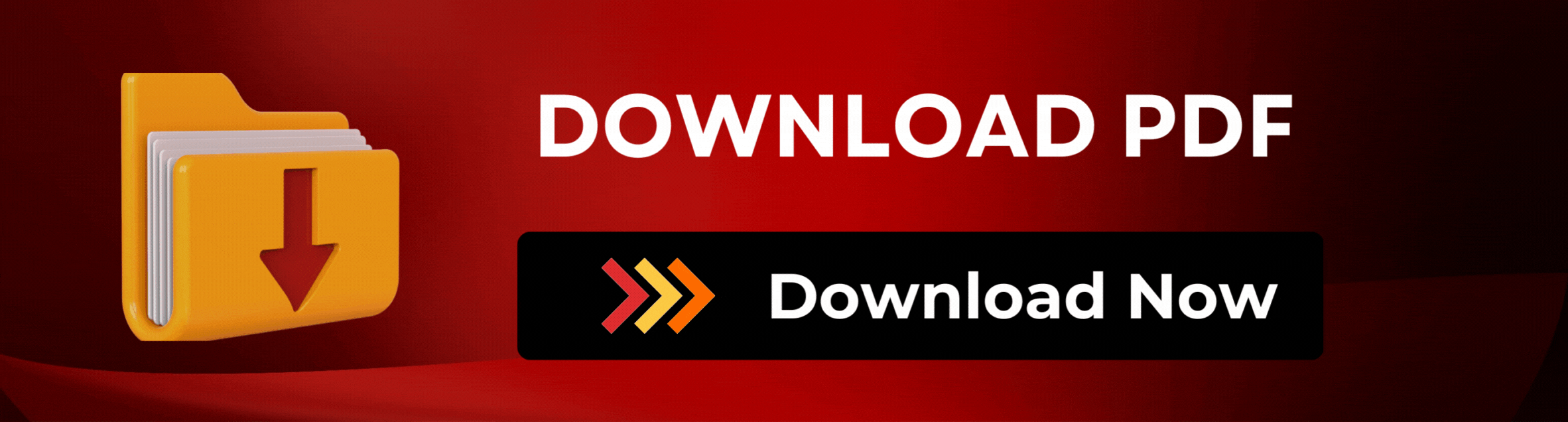