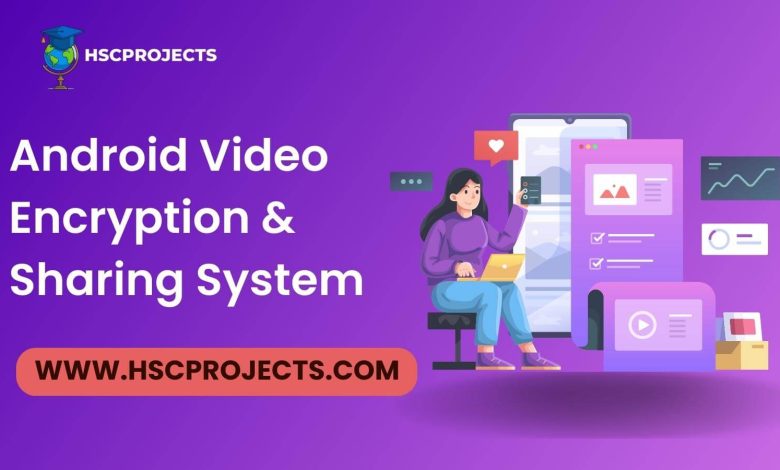
Android Video Encryption & Sharing System
Introduction
In the age of digital media, video sharing has become a ubiquitous part of our daily lives. However, the security of these videos often remains a significant concern. This article delves into an Android-based solution that employs AES and Blowfish encryption algorithms to secure videos, making it a unique offering in the realm of secure video sharing.
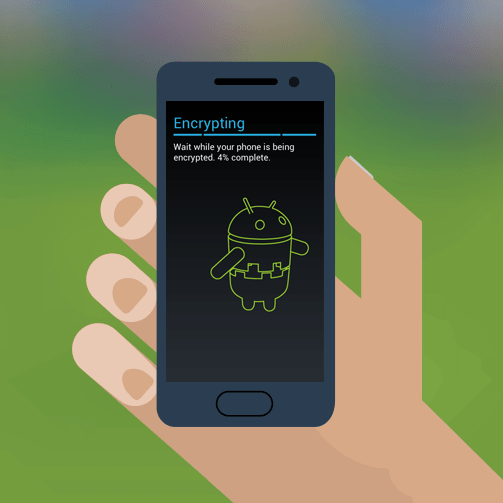
How It Works
The system allows users to upload videos, which are then encrypted using a two-tier encryption process. The video is divided into segments; one segment is encrypted using the AES algorithm, and the other using the Blowfish algorithm. Alternatively, the entire video can be encrypted first with AES and then with Blowfish. This dual encryption ensures a robust security layer, making it extremely difficult for unauthorized users to decrypt the video.
Key Features
- AES and Blowfish Encryption: The system uses two of the most secure encryption algorithms to ensure the confidentiality of your videos.
- Selective Sharing: Users have the option to share videos with specific users, enhancing privacy.
- Access Control: The uploader can grant or revoke access to the shared video, providing dynamic control over who can view the content.
- Download Option: Users can download encrypted videos, which can be decrypted and viewed within the application.
Advantages
- Enhanced Security: The dual encryption ensures that your videos are secure and protected from unauthorized access.
- User-Specific Sharing: The ability to share videos with specific users adds an extra layer of privacy.
- Dynamic Access Control: Granting and revoking access provides flexibility and control over video distribution.
- On-Demand Access: Users can download and view videos at their convenience.
Disadvantages
- Internet Dependency: An active internet connection is required for uploading and downloading videos.
- Processing Time: Larger video files may take more time to encrypt and decrypt, affecting user experience.
Conclusion
The Android Video Encryption & Sharing system offers a secure platform for video sharing by leveraging AES and Blowfish encryption algorithms. While it does have some limitations, such as the need for an internet connection and longer processing times for larger files, its advantages in terms of security and user-specific sharing make it a compelling option for those concerned about the privacy and security of their videos.
Sample Code
Add the necessary permissions to your AndroidManifest.xml
:
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/>
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
Utility class for AES encryption and decryption:
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
public class AESUtil {
private static final String ALGORITHM = "AES";
private static final byte[] keyValue = new byte[]{'T', 'h', 'i', 's', 'I', 's', 'A', 'S', 'e', 'c', 'r', 'e', 't', 'K', 'e', 'y'};
public static byte[] encrypt(byte[] data) throws Exception {
SecretKeySpec key = new SecretKeySpec(keyValue, ALGORITHM);
Cipher c = Cipher.getInstance(ALGORITHM);
c.init(Cipher.ENCRYPT_MODE, key);
return c.doFinal(data);
}
public static byte[] decrypt(byte[] encryptedData) throws Exception {
SecretKeySpec key = new SecretKeySpec(keyValue, ALGORITHM);
Cipher c = Cipher.getInstance(ALGORITHM);
c.init(Cipher.DECRYPT_MODE, key);
return c.doFinal(encryptedData);
}
}
Example of how you might use this utility class to encrypt a video file and then decrypt it:
import android.os.Environment;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
File sdCard = Environment.getExternalStorageDirectory();
File dir = new File(sdCard.getAbsolutePath() + "/videoDir");
dir.mkdirs();
// Assuming videoFile is the File object of existing video file to be encrypted
File videoFile = new File(dir, "myVideo.mp4");
// Encrypt the video
try {
FileInputStream fis = new FileInputStream(videoFile);
byte[] videoBytes = new byte[(int) videoFile.length()];
fis.read(videoBytes);
byte[] encryptedBytes = AESUtil.encrypt(videoBytes);
File encryptedFile = new File(dir, "myEncryptedVideo.enc");
FileOutputStream fos = new FileOutputStream(encryptedFile);
fos.write(encryptedBytes);
fos.close();
} catch (Exception e) {
e.printStackTrace();
}
// Decrypt the video
try {
File encryptedFile = new File(dir, "myEncryptedVideo.enc");
FileInputStream fis = new FileInputStream(encryptedFile);
byte[] encryptedBytes = new byte[(int) encryptedFile.length()];
fis.read(encryptedBytes);
byte[] decryptedBytes = AESUtil.decrypt(encryptedBytes);
File decryptedFile = new File(dir, "myDecryptedVideo.mp4");
FileOutputStream fos = new FileOutputStream(decryptedFile);
fos.write(decryptedBytes);
fos.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Android Video Encryption & Sharing System PDF
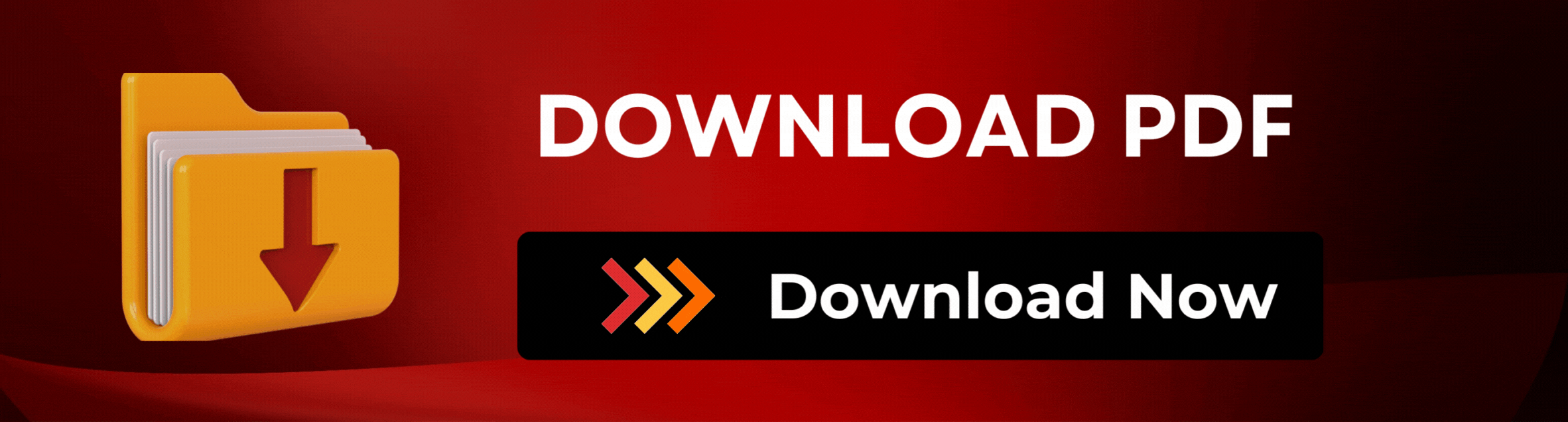