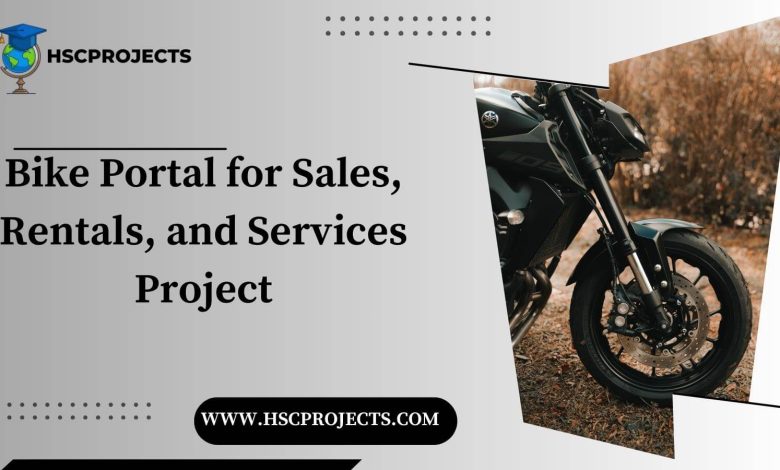
Bike Portal for Sales, Rentals, and Services Project
Introduction
The modern biker seeks more than just a ride; they seek a comprehensive experience that caters to all their biking needs. Welcome to our newly optimized platform, where you can buy, rent, and service bikes, as well as purchase parts and accessories—all under one digital roof.

Features
Comprehensive Listings
Our bike portal hosts an extensive range of bikes from multiple brands. Each listing is accompanied by detailed features, user reviews, and high-definition images, allowing you to make an informed decision.
Personalized Suggestions
Based on your browsing history and preferences, our system offers personalized bike and parts suggestions. It means you spend less time searching and more time riding.
Secure Payments
We understand the importance of secure transactions. Our platform offers a smooth and secure payment process, including credit card facilities, to ensure your peace of mind.
Rent a Bike
Not looking to buy just yet? Our ‘Rent a Bike’ feature lets you experience the thrill without the commitment. All rental bikes are thoroughly inspected to ensure your safety and enjoyment.
Community Articles
Join our vibrant community of biking enthusiasts. Share or read articles on various biking topics, and even comment on them. To contribute, simply register and share your insights with the world.
Efficient Inventory Management
For those in need of parts or accessories, our inventory management system is second to none. With real-time updates, you’ll never miss out on what you need.
Advantages
- Save time and effort with our user-friendly interface.
- Comprehensive comparisons allow you to make the best choice for your needs.
- Interactive graphical representations enhance your shopping experience.
- A transparent selling platform ensures you get the right price for your bike.
Disadvantages
- While our high-definition images aim to replicate the in-person experience, the visual effect of a physical inspection may differ.
Conclusion
Our bike portal is designed to be a comprehensive platform for all your biking needs. From purchasing to renting, from servicing to community interaction, we’ve got it all. So why wait? Get on the road to the ultimate biking experience today.
Sample Code
File Structure
- bikers_portal/
- app.py
- templates/
- index.html
- view_bike.html
- rent_bike.html
app.py
from flask import Flask, render_template, request, redirect
app = Flask(__name__)
# Mock data
bikes = [
{'id': 1, 'name': 'Mountain Bike', 'features': 'Off-road, 21 gears'},
{'id': 2, 'name': 'Road Bike', 'features': 'Lightweight, 18 gears'}
]
@app.route('/')
def index():
return render_template('index.html', bikes=bikes)
@app.route('/view/<int:bike_id>')
def view_bike(bike_id):
bike = next((item for item in bikes if item['id'] == bike_id), None)
return render_template('view_bike.html', bike=bike)
@app.route('/rent/<int:bike_id>', methods=['GET', 'POST'])
def rent_bike(bike_id):
bike = next((item for item in bikes if item['id'] == bike_id), None)
if request.method == 'POST':
# Implement your logic for renting the bike
return redirect('/')
return render_template('rent_bike.html', bike=bike)
if __name__ == '__main__':
app.run(debug=True)
index.html
<!DOCTYPE html>
<html>
<head>
<title>Bikers Portal</title>
</head>
<body>
<h1>Welcome to Bikers Portal</h1>
{% for bike in bikes %}
<a href="{{ url_for('view_bike', bike_id=bike.id) }}">{{ bike.name }}</a><br>
{% endfor %}
</body>
</html>
view_bike.html
<!DOCTYPE html>
<html>
<head>
<title>View Bike</title>
</head>
<body>
<h1>{{ bike.name }}</h1>
<p>Features: {{ bike.features }}</p>
<a href="{{ url_for('rent_bike', bike_id=bike.id) }}">Rent this bike</a>
</body>
</html>
rent_bike.html
<!DOCTYPE html>
<html>
<head>
<title>Rent Bike</title>
</head>
<body>
<h1>Rent {{ bike.name }}</h1>
<form method="post">
<!-- Your form fields here -->
<button type="submit">Rent Bike</button>
</form>
</body>
</html>
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Download Bike Portal for Sales, Rentals, and Services Project PDF
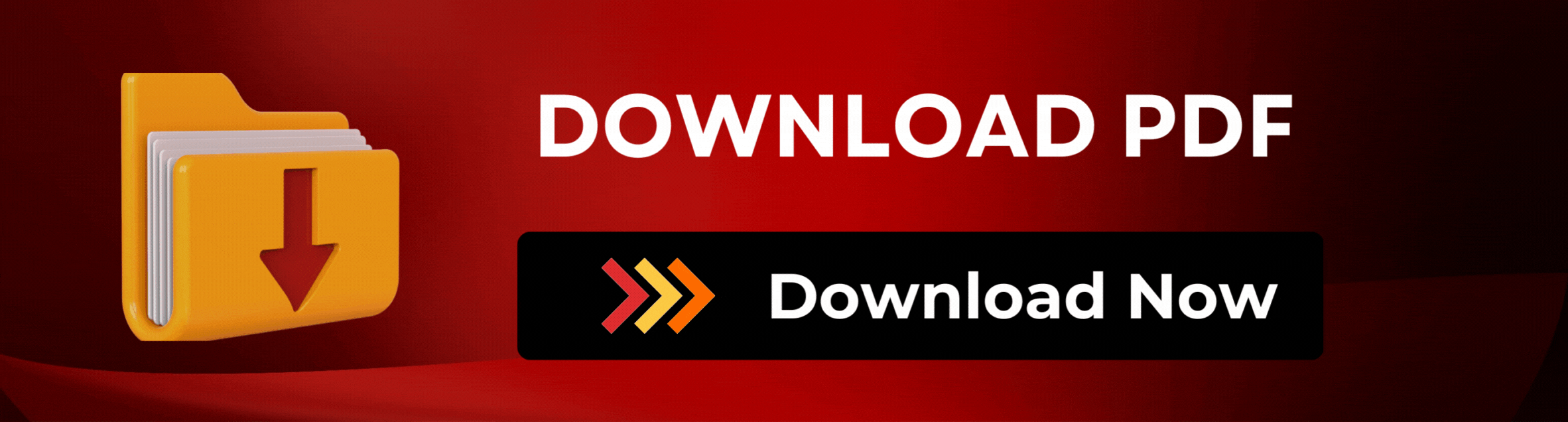