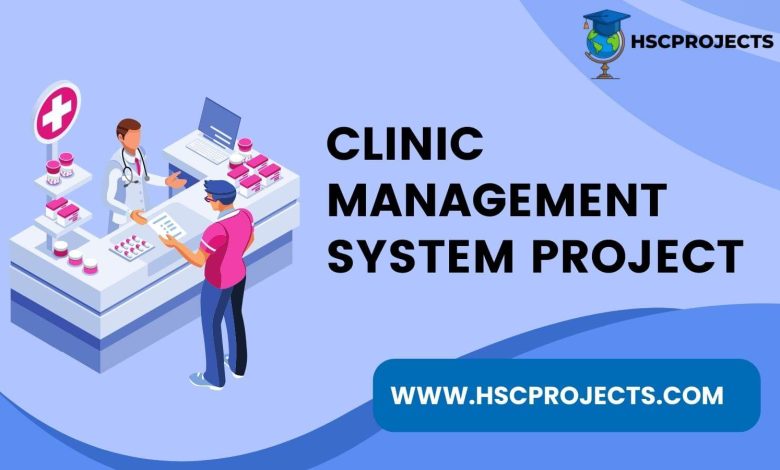
Clinic Management System Project
Introduction
The healthcare industry is undergoing a digital transformation, and one of the key drivers is the adoption of clinic management systems. These systems not only simplify the workflow for healthcare providers but also enhance the patient experience. In this article, we delve into the intricacies of clinic management systems and how they are revolutionizing healthcare.
How It Works
A clinic management system is a software solution designed to facilitate seamless communication between healthcare providers and administrative staff. Typically, there are two primary users: the doctor and the receptionist. The receptionist assigns token numbers to patients and saves their details in the database. This information is then sent to the doctor, who can review it before the consultation. Post-consultation, the doctor inputs the prescribed medicines into the system, which the receptionist can access to generate bills and update records.

Key Features
- Patient Records: Stores comprehensive patient histories, making it easier for doctors to review past treatments.
- Billing: Automates the billing process, eliminating the need for manual calculations.
- Database Management: Robust SQL database support for storing user-specific details.
- User-Friendly Interface: Developed on the C#.net platform, the system is intuitive and easy to navigate.
Advantages
- Automation: Replaces manual procedures, making clinic management more efficient.
- Accessibility: Doctors can easily access patient records and treatment histories.
- Cost-Effectiveness: Reduces the need for paper records, saving time and resources.
- Convenience: The system is flexible and can be customized to suit the specific needs of a clinic.
Limitations
- Database Requirements: The system requires a large database, which could be a limitation for smaller clinics.
- Manual Updates: Users must manually input new information, which could lead to errors if not done carefully.
Conclusion
Clinic management systems are an invaluable tool for modern healthcare facilities, offering a range of features that streamline administrative tasks and improve patient care. While there are some limitations, the advantages far outweigh the drawbacks, making it a must-have for any clinic looking to optimize its operations.
Sample Code
# Clinic Management System
# Simulated database
patients_db = {}
def add_patient(token, name, age, symptoms):
patients_db[token] = {
'Name': name,
'Age': age,
'Symptoms': symptoms,
'Prescription': None,
'Bill': None
}
def view_patient(token):
return patients_db.get(token, "Patient not found")
def add_prescription(token, prescription):
if token in patients_db:
patients_db[token]['Prescription'] = prescription
else:
return "Patient not found"
def generate_bill(token, amount):
if token in patients_db:
patients_db[token]['Bill'] = amount
else:
return "Patient not found"
def main():
while True:
print("\nClinic Management System")
print("1. Add Patient")
print("2. View Patient")
print("3. Add Prescription")
print("4. Generate Bill")
print("5. Exit")
choice = input("Enter your choice: ")
if choice == '1':
token = input("Enter Token Number: ")
name = input("Enter Patient Name: ")
age = input("Enter Patient Age: ")
symptoms = input("Enter Symptoms: ")
add_patient(token, name, age, symptoms)
elif choice == '2':
token = input("Enter Token Number: ")
print(view_patient(token))
elif choice == '3':
token = input("Enter Token Number: ")
prescription = input("Enter Prescription: ")
add_prescription(token, prescription)
elif choice == '4':
token = input("Enter Token Number: ")
amount = input("Enter Bill Amount: ")
generate_bill(token, amount)
elif choice == '5':
break
else:
print("Invalid choice. Please try again.")
if __name__ == "__main__":
main()
To run the code, copy it into a Python editor and execute it. Follow the on-screen instructions to add patients, view patient details, add prescriptions, and generate bills.
Remember, this is a simplified example and lacks many features like error handling, data persistence, and security measures that a real-world application would require.
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Subscribed? Click on Confirm
Download Clinic Management System Project PDF
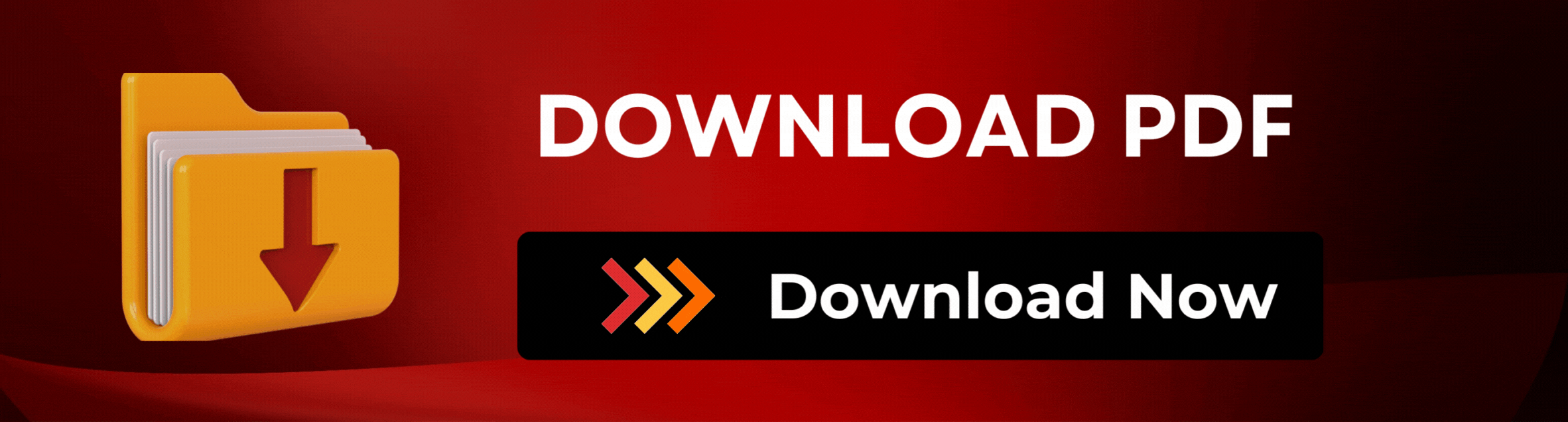