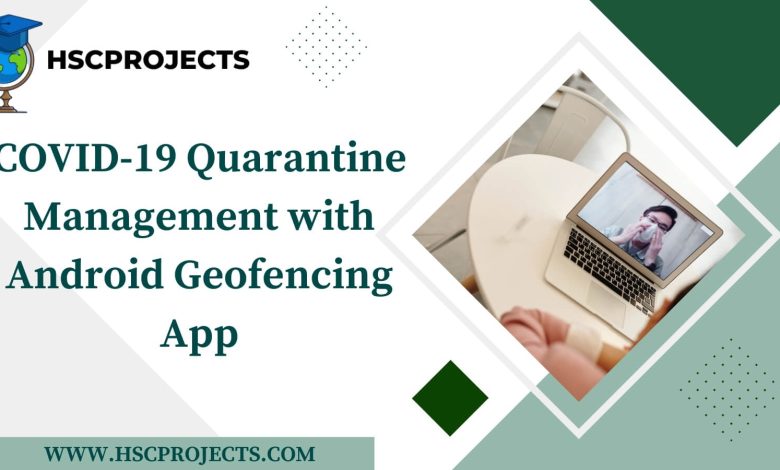
COVID-19 Quarantine Management with Android Geofencing App
Introduction
Enforcing quarantine during the ongoing COVID-19 pandemic has presented unprecedented challenges for authorities worldwide. Our Android Geofencing App for quarantine management offers an innovative solution to monitor and regulate the movement of quarantined individuals.

Features
- Two Users Interface: The app is designed for two types of users—Admin and Patient.
- Boundary Setting: Admins can set geographical boundaries, measured in meters, to confine the quarantined individual.
- Movement Tracking: The app records and displays the movement of the patient within the set geographical boundary.
- Google Sign-In: Users can easily sign in through their Google account.
- Account Freezing: Users have the option to freeze their accounts temporarily if necessary.
How it Works
The application utilizes geofencing technology to create a virtual perimeter around the quarantine location. When a user, flagged as ‘Positive,’ steps out of this boundary, an alert is sent to the Admin. Admins can not only set these boundaries but can also monitor how many times a person has moved in or out of the fenced area.
Security Measures
The app is secure, with login and logout options managed by the Admin. Only those with the password can logout or disable location updates, making the system tamper-proof.
Advantages
- Enhanced Compliance: The app ensures that quarantined individuals are staying within designated areas, thereby improving overall compliance.
- Efficient Monitoring: Allows authorities to monitor multiple patients simultaneously, reducing the need for physical surveillance.
- User Convenience: Google Sign-In and the ability to freeze accounts provide user flexibility while maintaining stringent monitoring.
Conclusion
The Android Geofencing App is a vital tool in the battle against COVID-19. It balances the need for stringent monitoring with user convenience and administrative efficiency, making it an indispensable asset for effective quarantine management.
Sample Code
AndroidManifest.xml
<manifest ... >
<!-- Permissions -->
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
...
<!-- Services -->
<service android:name=".GeofenceTransitionsIntentService" />
...
</manifest>
MainActivity.java
import android.app.PendingIntent;
import android.content.Intent;
import com.google.android.gms.location.GeofencingClient;
import com.google.android.gms.location.GeofencingRequest;
import com.google.android.gms.location.Geofence;
public class MainActivity extends AppCompatActivity {
private GeofencingClient geofencingClient;
private PendingIntent geofencePendingIntent;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
geofencingClient = LocationServices.getGeofencingClient(this);
// Create a Geofence
Geofence geofence = new Geofence.Builder()
.setRequestId("GEOFENCE_ID")
.setCircularRegion(37.422, -122.084, 100) // lat, long, radius in meters
.setExpirationDuration(Geofence.NEVER_EXPIRE)
.setTransitionTypes(Geofence.GEOFENCE_TRANSITION_ENTER | Geofence.GEOFENCE_TRANSITION_EXIT)
.build();
// Create a GeofencingRequest
GeofencingRequest geofencingRequest = new GeofencingRequest.Builder()
.setInitialTrigger(GeofencingRequest.INITIAL_TRIGGER_ENTER)
.addGeofence(geofence)
.build();
// Create a PendingIntent
Intent intent = new Intent(this, GeofenceTransitionsIntentService.class);
geofencePendingIntent = PendingIntent.getService(this, 0, intent, PendingIntent.FLAG_UPDATE_CURRENT);
// Add Geofence
geofencingClient.addGeofences(geofencingRequest, geofencePendingIntent);
}
}
GeofenceTransitionsIntentService.java
import android.app.IntentService;
import android.content.Intent;
import com.google.android.gms.location.Geofence;
import com.google.android.gms.location.GeofencingEvent;
public class GeofenceTransitionsIntentService extends IntentService {
public GeofenceTransitionsIntentService() {
super("GeofenceTransitionsIntentService");
}
@Override
protected void onHandleIntent(Intent intent) {
GeofencingEvent geofencingEvent = GeofencingEvent.fromIntent(intent);
if (geofencingEvent.hasError()) {
// Handle error
return;
}
int geofenceTransition = geofencingEvent.getGeofenceTransition();
if (geofenceTransition == Geofence.GEOFENCE_TRANSITION_ENTER) {
// Send notification or alert to Admin about the quarantine violation
} else if (geofenceTransition == Geofence.GEOFENCE_TRANSITION_EXIT) {
// Send different notification or alert
}
}
}
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download COVID-19 Quarantine Management with Android Geofencing App PDF
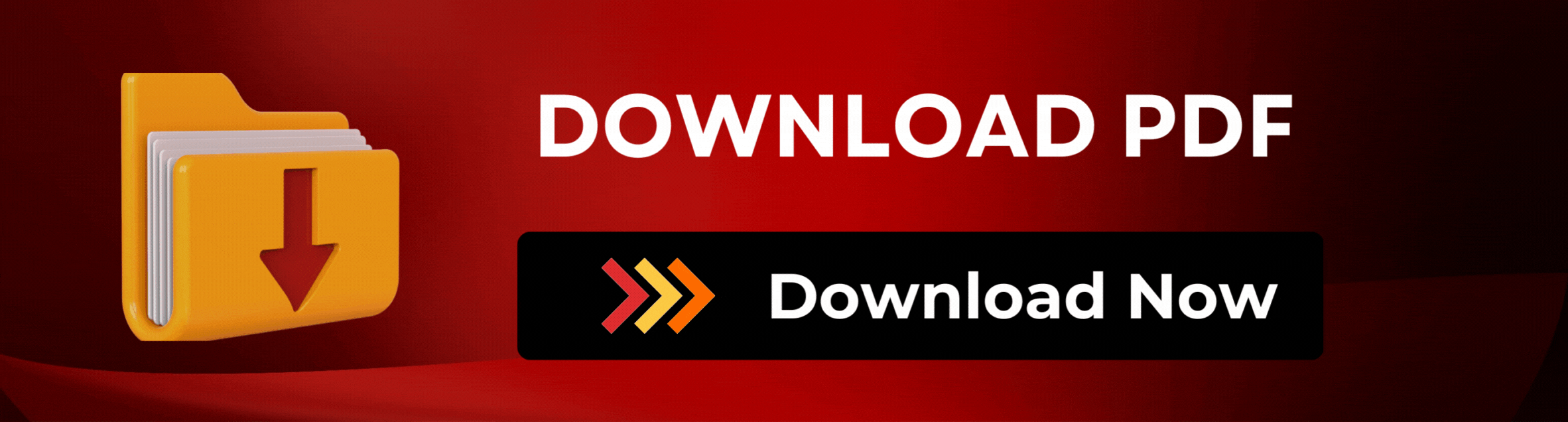