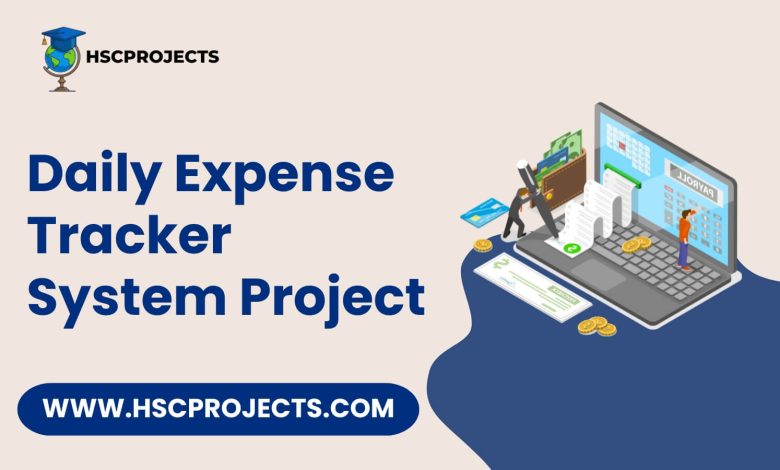
Daily Expense Tracker System Project
Introduction
Managing household finances can be a daunting task, especially when you’re juggling multiple responsibilities. The Daily Expense Tracker System is designed to simplify this process, providing a comprehensive solution for tracking income, expenses, and savings. Whether you’re a housewife, a student, or anyone looking to gain better control over their finances, this system is for you.
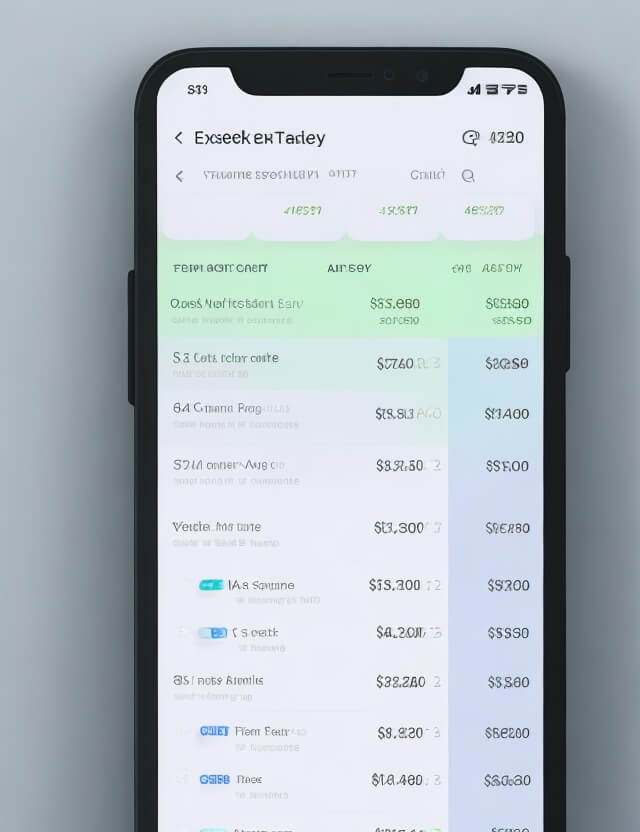
How Does It Work?
The Daily Expense Tracker System is an intuitive platform that allows you to input your income and categorize your expenses. The system then calculates your daily spending limit based on your income. If you exceed the daily limit, the system adjusts your future spending allowance. Conversely, if you spend less than the daily limit, the surplus is added to your savings.
Key Features
- User Login & Registration: Securely access your financial data.
- Add Expense: Input your daily expenses and categorize them for better tracking.
- Add Income: Update your income sources and amounts.
- View Income-Expense Curve: Visualize your financial trends with a monthly report.
- Savings: Track your savings and allocate them for special occasions.
- Special Expense: Add expenses that are expected to be refunded, like deposits or reimbursements.
Advantages
- Financial Awareness: Gain a better understanding of your spending habits.
- Savings Growth: Encourages you to save by showing the potential growth of your savings.
- Budgeting: Helps you stick to a budget by adjusting your daily spending limit.
- Special Occasions: Allows you to allocate savings for special events like birthdays or anniversaries.
Who Can Benefit?
While the system was initially designed with housewives in mind, its features make it versatile enough for anyone looking to improve their financial management skills. Whether you’re a freelancer juggling multiple income streams or a student managing a tight budget, the Daily Expense Tracker System can help you gain financial stability.
Conclusion
The Daily Expense Tracker System offers a comprehensive solution for managing your daily finances. With features like income and expense tracking, savings management, and monthly reporting, you can take control of your financial future. Start using our daily expense tracker app or website today and see the difference it can make in your life.
Sample Code
First, install the required packages if you haven’t:
pip install Flask
pip install Flask-SQLAlchemy
from flask import Flask, request, jsonify
from flask_sqlalchemy import SQLAlchemy
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///expenses.db'
db = SQLAlchemy(app)
class Expense(db.Model):
id = db.Column(db.Integer, primary_key=True)
description = db.Column(db.String(50))
amount = db.Column(db.Float)
class Income(db.Model):
id = db.Column(db.Integer, primary_key=True)
source = db.Column(db.String(50))
amount = db.Column(db.Float)
db.create_all()
@app.route('/add_expense', methods=['POST'])
def add_expense():
data = request.get_json()
new_expense = Expense(description=data['description'], amount=data['amount'])
db.session.add(new_expense)
db.session.commit()
return jsonify({"message": "Expense added"}), 201
@app.route('/add_income', methods=['POST'])
def add_income():
data = request.get_json()
new_income = Income(source=data['source'], amount=data['amount'])
db.session.add(new_income)
db.session.commit()
return jsonify({"message": "Income added"}), 201
@app.route('/get_expenses', methods=['GET'])
def get_expenses():
expenses_list = Expense.query.all()
expenses = [{"id": x.id, "description": x.description, "amount": x.amount} for x in expenses_list]
return jsonify({"expenses": expenses})
@app.route('/get_income', methods=['GET'])
def get_income():
income_list = Income.query.all()
incomes = [{"id": x.id, "source": x.source, "amount": x.amount} for x in income_list]
return jsonify({"incomes": incomes})
if __name__ == '__main__':
app.run(debug=True)
To use this code:
- Save it in a file, for example,
app.py
. - Run the file:
python app.py
. - Use Postman or curl to test the API endpoints.
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Daily Expense Tracker System Project PDF
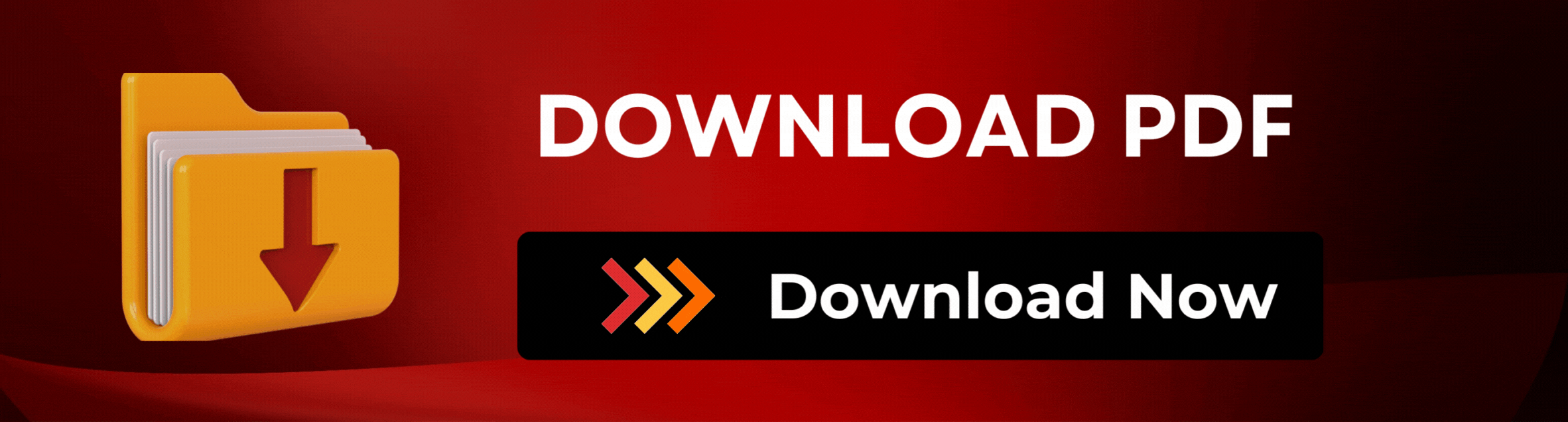