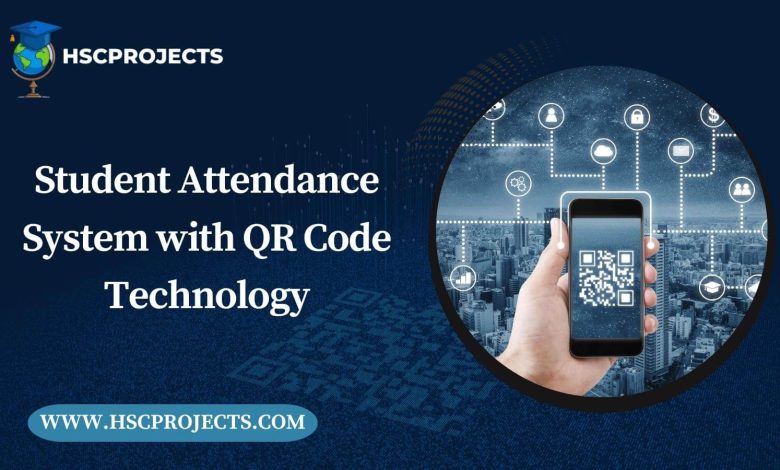
Student Attendance System with QR Code Technology
In today’s digital age, educational institutions are perpetually on the lookout for smart solutions to streamline their operational processes. One such innovative solution is the QR Code Attendance System, a contemporary approach to managing student attendance with a touch of modern technology. This system not only simplifies the attendance taking procedure but also paves the way for a paperless, efficient, and eco-friendly environment within schools and colleges.
At the core of this attendance management system is the QR code technology. Each student is issued a unique card embedded with a QR code, which serves as their digital identity. This QR code attendance system operates on a straightforward principle; students flash their cards before a webcam, which scans the QR code and registers their attendance for the day. The system is designed to recognize each QR code’s unique ID, corresponding to the individual student, thus ensuring accuracy and preventing any attendance fraud.

The operation of the QR code based attendance system is seamless. As students step into the classroom and scan their cards, their attendance data is instantly captured and logged against the respective dates. This eradication of manual attendance marking not only saves precious time but also nullifies the chances of human error, which is often a concern in conventional attendance systems.
One of the standout features of this system is the automatic generation of insightful reports. The system diligently stores all the attendance records, enabling the administration to track attendance trends over time. Should there be any attendance discrepancies or habitual defaulters, the system is adept at flagging such instances and generating a defaulter list. Furthermore, it extends its functionality to provide an overall attendance report, exported in an Excel sheet format for the administrative personnel. This feature is particularly beneficial for analyzing attendance data over a term or academic year, aiding in informed decision-making.
The application of the QR code attendance system goes beyond just marking attendance. It embodies a progressive step towards integrating technology in educational management, making daily operations more streamlined, data-driven, and transparent. By adopting such smart attendance solutions, schools and colleges are not just keeping up with the technological trends, but also fostering a conducive environment for both administrative efficiency and academic excellence.
Sample Code
pip install opencv-python opencv-python-headless numpy pandas
import cv2
import numpy as np
import pandas as pd
from datetime import date
# Function to scan QR code and return the decoded text
def scan_qr_code(cap):
ret, frame = cap.read()
detector = cv2.QRCodeDetector()
value, pts, qr_code = detector(frame)
return value
def main():
cap = cv2.VideoCapture(0)
attendance_file = 'attendance.csv'
# Check if attendance file exists, if not create one with headers
try:
attendance_data = pd.read_csv(attendance_file)
except FileNotFoundError:
attendance_data = pd.DataFrame(columns=['ID', 'Date'])
attendance_data.to_csv(attendance_file, index=False)
while True:
print("Ask the student to show the QR code to the webcam.")
input("Press Enter once ready...")
student_id = scan_qr_code(cap)
if student_id:
print(f"Student ID: {student_id}")
# Check if the student has already been marked present for the day
today = date.today()
if not ((attendance_data['ID'] == student_id) & (attendance_data['Date'] == str(today))).any():
# Mark attendance
new_record = pd.DataFrame({'ID': [student_id], 'Date': [str(today)]})
attendance_data = attendance_data.append(new_record, ignore_index=True)
attendance_data.to_csv(attendance_file, index=False)
print(f"Attendance marked for student {student_id}.")
else:
print(f"Attendance already marked for student {student_id}.")
else:
print("No QR code detected. Try again.")
# Exit on 'q' key press
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
if __name__ == "__main__":
main()
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Student Attendance System with QR Code Technology PDF
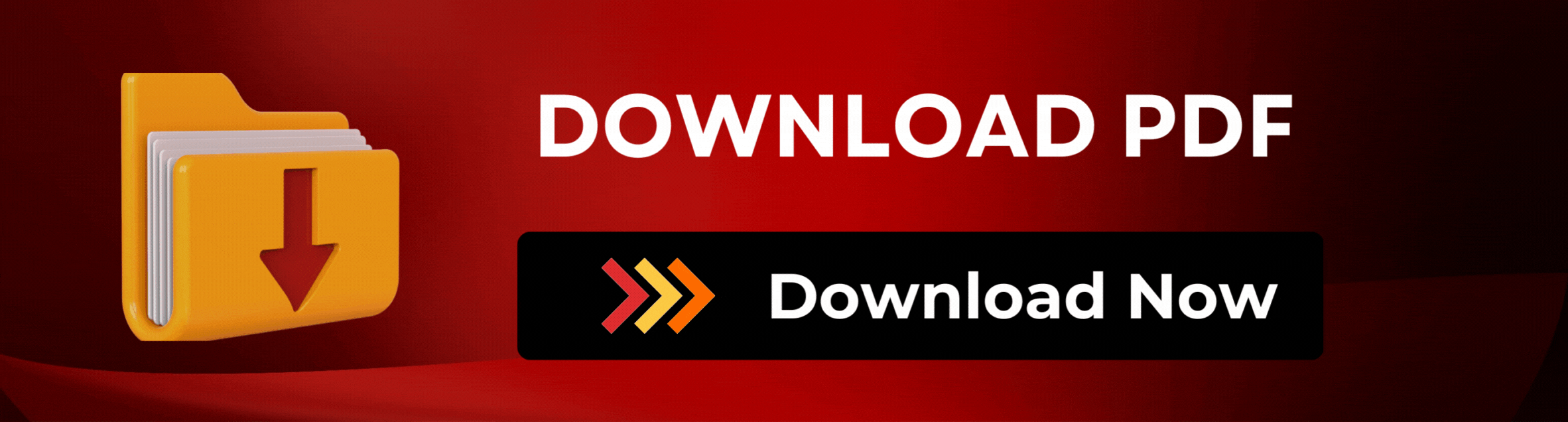