
Exam Cell Automation Systems
Introduction
In the era of digital transformation, traditional manual methods in educational settings are gradually giving way to automated solutions. The exam cell automation system serves as a paradigm shift in how exams are conducted, making the process more organized, efficient, and less prone to errors.

Features
- Secure Registration: The exam cell automation system offers a secure platform where students can register for their exams, providing vital details such as name, semester, and subjects.
- Automated Hall Ticket Generation: Gone are the days of standing in long queues for hall tickets. The system automatically generates hall tickets which are sent directly to students’ emails.
- Mark Sheet Management: Admins can effortlessly populate marks for each student across different semesters and subjects, directly through a Graphical User Interface (GUI).
Advantages
- Security: With secure login credentials, unauthorized access is effectively curbed.
- Convenience: Students can print hall tickets at their leisure and can also update personal details online.
- Speed and Efficiency: The system automates various functions like hall ticket generation and marks entry, saving valuable time.
- Data Accuracy: Reduces the chances of errors that are common in manual methods.
Disadvantages
- Data Integrity: A small mistake in data entry can lead to inaccuracies across the system.
- Dependency on Internet: Being an online system, network issues can sometimes cause delays or missed notifications.
Conclusion
The exam cell automation system abstracts away much of the tedious work involved in conducting examinations. From generating hall tickets to entering marks, every aspect is managed online, making the process easier for both students and administrators. However, like any system, it’s not without its shortcomings. Accurate data entry is crucial, and a reliable internet connection is a must. Despite these challenges, the advantages far outweigh the disadvantages, making the exam cell automation system a cornerstone in modern education technology.
Sample Code
from flask import Flask, render_template, request, redirect, url_for
import sqlite3
app = Flask(__name__)
# Initialize SQLite Database
def init_db():
with sqlite3.connect("exam_cell.db") as conn:
cur = conn.cursor()
cur.execute("""
CREATE TABLE IF NOT EXISTS students (
id INTEGER PRIMARY KEY AUTOINCREMENT,
name TEXT,
email TEXT,
semester INTEGER
);
""")
cur.execute("""
CREATE TABLE IF NOT EXISTS marks (
id INTEGER PRIMARY KEY AUTOINCREMENT,
student_id INTEGER,
subject TEXT,
mark INTEGER,
FOREIGN KEY (student_id) REFERENCES students(id)
);
""")
conn.commit()
init_db()
@app.route("/")
def index():
return render_template("index.html")
@app.route("/register", methods=["POST"])
def register():
name = request.form["name"]
email = request.form["email"]
semester = request.form["semester"]
with sqlite3.connect("exam_cell.db") as conn:
cur = conn.cursor()
cur.execute("INSERT INTO students (name, email, semester) VALUES (?, ?, ?)", (name, email, semester))
conn.commit()
# Normally, you'd send the hall ticket by email here.
return redirect(url_for("index"))
@app.route("/admin", methods=["GET", "POST"])
def admin():
if request.method == "POST":
student_id = request.form["student_id"]
subject = request.form["subject"]
mark = request.form["mark"]
with sqlite3.connect("exam_cell.db") as conn:
cur = conn.cursor()
cur.execute("INSERT INTO marks (student_id, subject, mark) VALUES (?, ?, ?)", (student_id, subject, mark))
conn.commit()
with sqlite3.connect("exam_cell.db") as conn:
cur = conn.cursor()
cur.execute("SELECT * FROM students")
students = cur.fetchall()
return render_template("admin.html", students=students)
if __name__ == "__main__":
app.run(debug=True)
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Exam Cell Automation Systems PDF
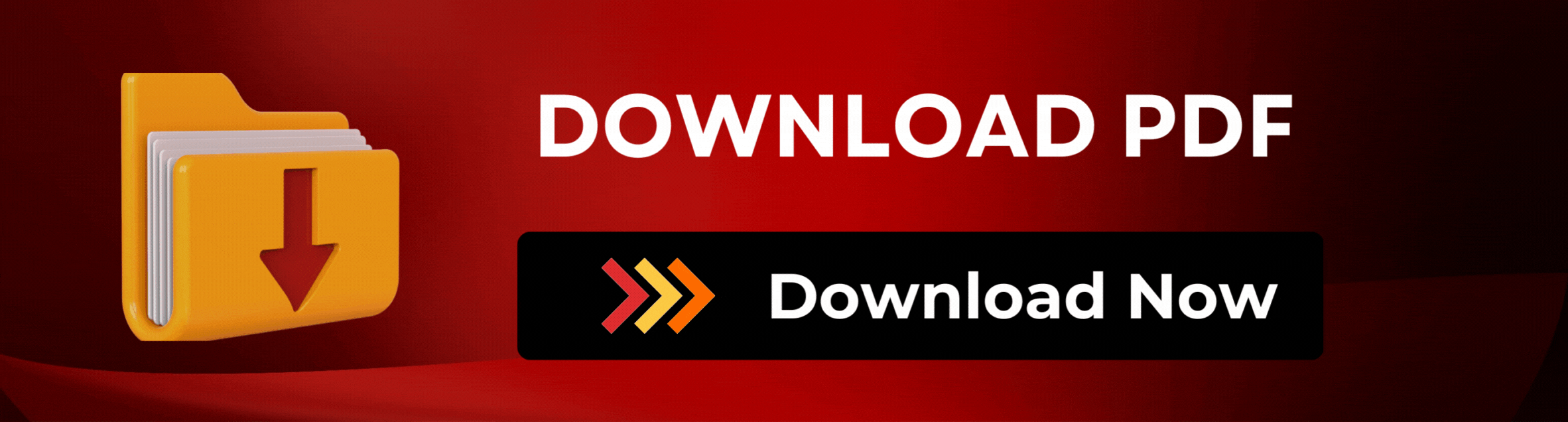