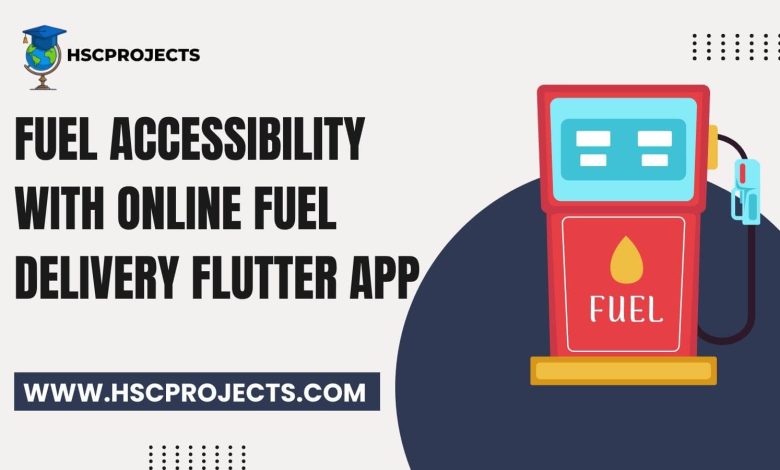
Fuel Accessibility with Online Fuel Delivery Flutter App
Introduction
The traditional method of refueling vehicles by driving them to the nearest gas station is becoming increasingly inconvenient and inefficient, especially for companies operating large fleets. Our Online Fuel Delivery Flutter App aims to revolutionize this process by delivering fuel directly to your location, thereby saving time and reducing operational costs.
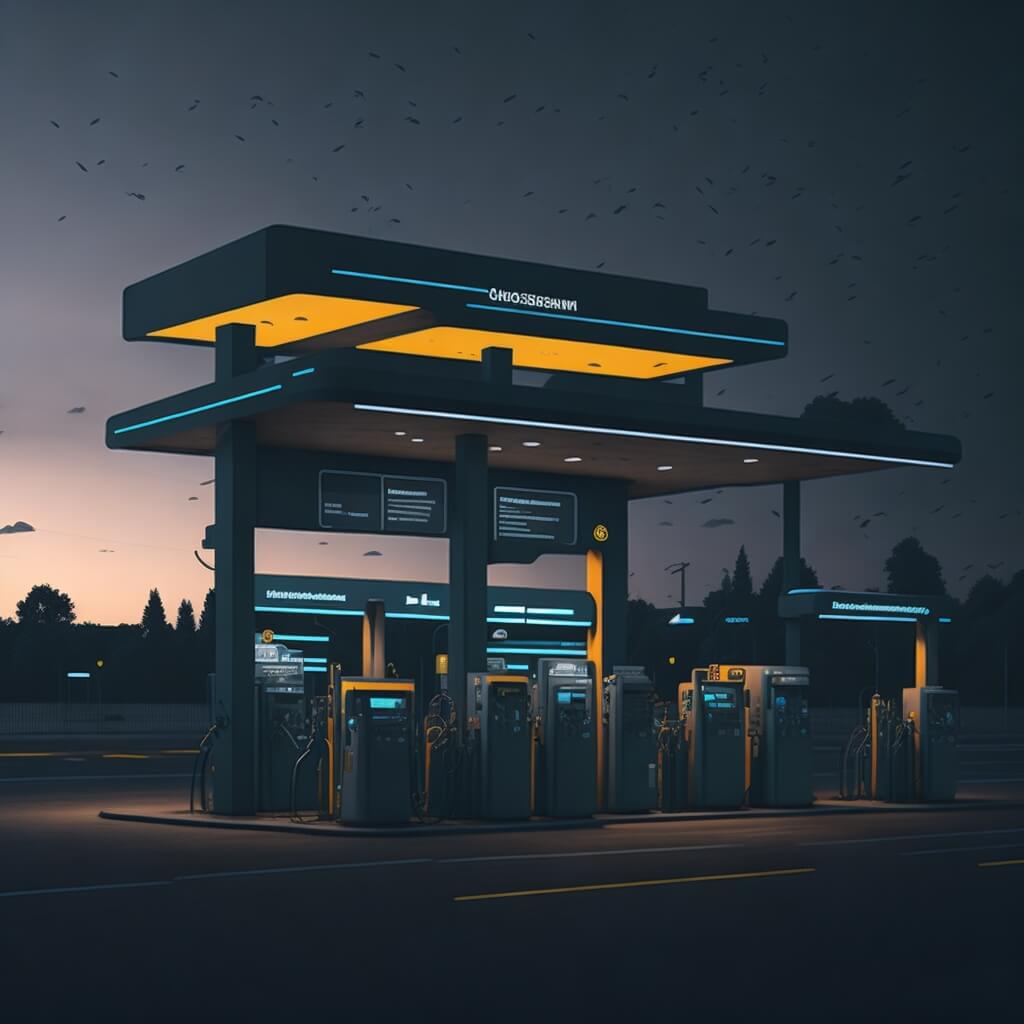
How It Works
The app consists of three main entities: the Admin, the Gas Station, and the User. Developed using Flutter, the app provides a seamless experience across various platforms.
- User Experience: Users can easily specify their location, choose the type of fuel they need—be it petrol or diesel—and the quantity required. Payment can be made securely within the app, making the entire process hassle-free.
- Gas Station Interface: Once an order is confirmed, the details are sent to the nearest participating gas station. The gas station then prepares the order for delivery.
- Admin Control: The admin has complete control over the gas stations, including setting fuel types and prices. This ensures that gas stations cannot manipulate prices, providing a fair system for all.
Key Features
- Online Payment: Users can pay for their fuel online, ensuring a contactless and convenient transaction.
- Real-Time Tracking: Users can track their fuel delivery in real-time, offering transparency and peace of mind.
- Inventory Management: Gas stations can update their fuel inventory, ensuring they only accept orders they can fulfill.
Advantages
- Convenience: Fuel is delivered directly to the user’s location, eliminating the need to drive to a gas station.
- Transparency: With the admin setting fuel prices, users can be assured of fair pricing.
- Efficiency: The app streamlines the fuel ordering process, making it efficient for both users and gas stations.
Conclusion
Our Online Fuel Delivery Flutter App is not just an innovation but a revolution in fuel delivery app development. It offers a win-win situation for all parties involved, making the refueling process more efficient, transparent, and user-friendly.
Sample Code
First, make sure you have Flutter and Dart installed on your machine.
Create a new Flutter project by running flutter create fuel_delivery_app
in your terminal.
Navigate to the lib
directory and replace the contents of main.dart
with the following code:
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: FuelOrderPage(),
);
}
}
class FuelOrderPage extends StatefulWidget {
@override
_FuelOrderPageState createState() => _FuelOrderPageState();
}
class _FuelOrderPageState extends State<FuelOrderPage> {
String _selectedFuelType;
double _selectedQuantity;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Online Fuel Delivery'),
),
body: Padding(
padding: const EdgeInsets.all(16.0),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text('Select Fuel Type:'),
DropdownButton<String>(
value: _selectedFuelType,
items: ['Petrol', 'Diesel'].map((String value) {
return DropdownMenuItem<String>(
value: value,
child: Text(value),
);
}).toList(),
onChanged: (newValue) {
setState(() {
_selectedFuelType = newValue;
});
},
),
SizedBox(height: 20),
Text('Select Quantity (Liters):'),
Slider(
value: _selectedQuantity ?? 0,
min: 0,
max: 100,
divisions: 20,
label: _selectedQuantity?.round()?.toString(),
onChanged: (double value) {
setState(() {
_selectedQuantity = value;
});
},
),
ElevatedButton(
onPressed: () {
// Implement your order logic here
print('Ordered $_selectedQuantity liters of $_selectedFuelType');
},
child: Text('Order Now'),
),
],
),
),
);
}
}
This is a very basic example and doesn’t include features like real-time tracking, admin control, or online payment. However, it should give you a starting point for developing a more comprehensive fuel delivery app.
To run the app, navigate to the project directory in your terminal and run flutter run
.
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Fuel Accessibility with Online Fuel Delivery Flutter App PDF
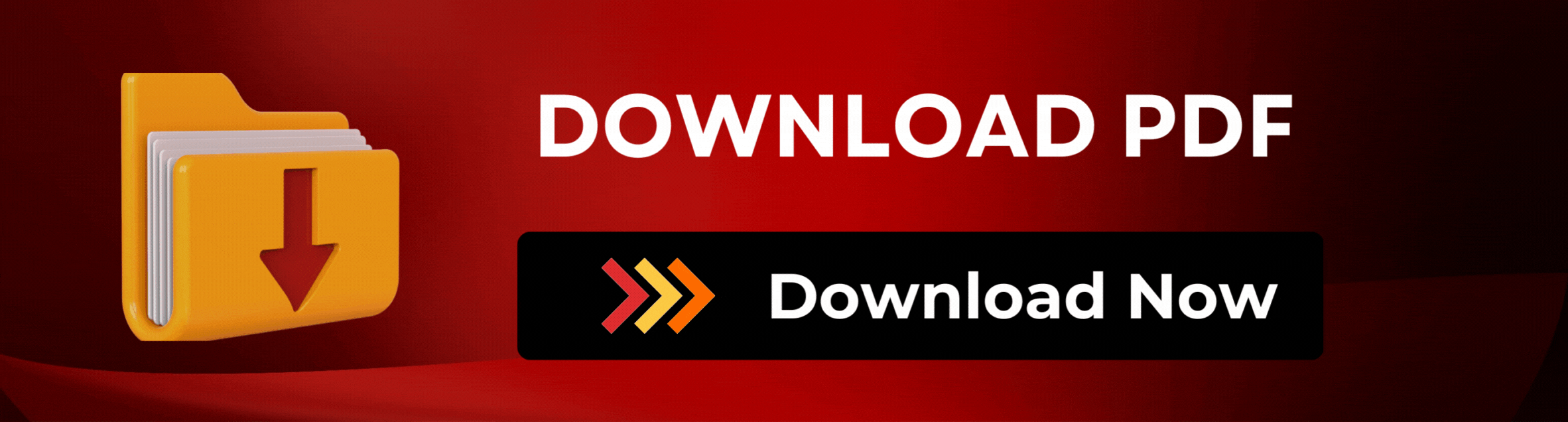