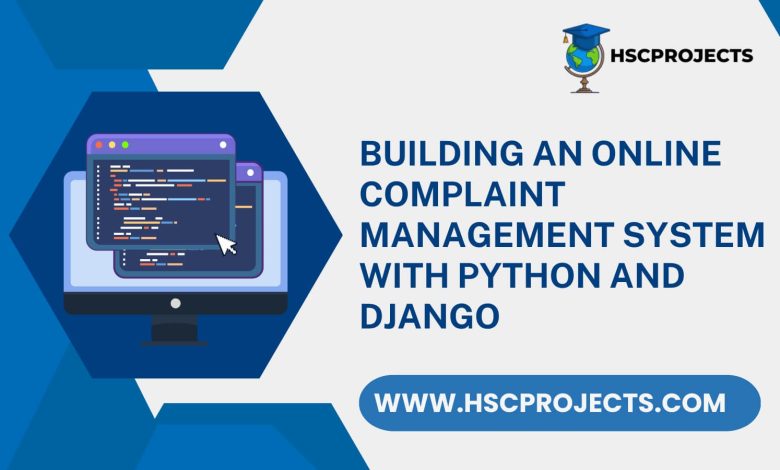
Building an Online Complaint Management System with Python and Django
Introduction
In today’s digital age, the need for an efficient and transparent complaint management system is more critical than ever. Traditional methods often involve tedious paperwork and long waiting times, leading to dissatisfaction among the public. This article aims to guide you through the process of building an online complaint management system using Python and Django, which not only speeds up the complaint resolution process but also minimizes corruption.
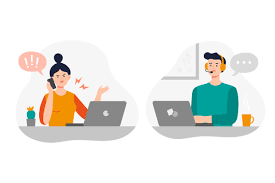
Why Choose Python and Django?
Python is known for its simplicity and readability, making it an excellent choice for web development projects. Django, a high-level Python web framework, further simplifies the process by providing reusable code “app” structures, which can save a lot of time.
Core Features
- User Registration: Allows users to create an account where they can log in to file complaints.
- Complaint Registration: Users can easily register their complaints online.
- Complaint Tracking: Enables users to track the status of their complaints.
- Admin Panel: Officers can view, manage, and resolve complaints.
Technical Stack
- Front-end: HTML, CSS, and JavaScript
- Back-end: Python
- Framework: Django
- Database: MySQL
Advantages of the Online Complaint Management System
- User-Friendly Interface: The system is designed to be intuitive and easy to navigate.
- Efficiency: The digital platform speeds up the complaint resolution process.
- Transparency: Users can track the status of their complaints, leading to greater accountability.
Steps to Build the System
- Set up Django Project: Initialize a new Django project and create a new app for the complaint management system.
- Database Configuration: Use MySQL to set up your database and connect it to your Django project.
- User Interface: Design the front-end using HTML, CSS, and JavaScript.
- Complaint Registration and Tracking: Implement these features using Python and Django’s built-in functionalities.
- Admin Panel: Create an admin panel where officers can manage complaints.
- Testing: Thoroughly test the system for any bugs or issues.
Conclusion
An online complaint management system not only streamlines the complaint resolution process but also brings about transparency and accountability. Python and Django make it easier to implement such a system, offering a robust and user-friendly platform for both the public and the officers.
Sample Code
Prerequisites
- Python installed
- Django installed
- MySQL installed
Steps
Create a new Django project and app
django-admin startproject ComplaintManagement
cd ComplaintManagement
python manage.py startapp complaint_app
Configure Database in settings.py
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.mysql',
'NAME': 'complaint_db',
'USER': 'root',
'PASSWORD': 'password',
'HOST': 'localhost',
'PORT': '3306',
}
}
Create a model for Complaints in models.py
from django.db import models
class Complaint(models.Model):
title = models.CharField(max_length=200)
description = models.TextField()
status = models.CharField(max_length=10, default='Pending')
Register the model in admin.py
from django.contrib import admin
from .models import Complaint
admin.site.register(Complaint)
Create a form for Complaint Registration in forms.py
from django import forms
from .models import Complaint
class ComplaintForm(forms.ModelForm):
class Meta:
model = Complaint
fields = ['title', 'description']
Create views for Users and Admins in views.py
from django.shortcuts import render, redirect
from .forms import ComplaintForm
from .models import Complaint
def register_complaint(request):
if request.method == 'POST':
form = ComplaintForm(request.POST)
if form.is_valid():
form.save()
return redirect('admin_panel')
else:
form = ComplaintForm()
return render(request, 'register_complaint.html', {'form': form})
def admin_panel(request):
complaints = Complaint.objects.all()
return render(request, 'admin_panel.html', {'complaints': complaints})
Create templates for User and Admin
- Create a folder named
templates
inside your app directory and add HTML files for user and admin panels.
Run Migrations
python manage.py makemigrations
python manage.py migrate
Run Server
python manage.py runserver
This is a very basic example to get you started. You can extend this by adding user authentication, complaint tracking, and other advanced features.
Remember to create the necessary HTML templates (register_complaint.html
and admin_panel.html
) to make the views work.
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Building an Online Complaint Management System with Python and Django PDF
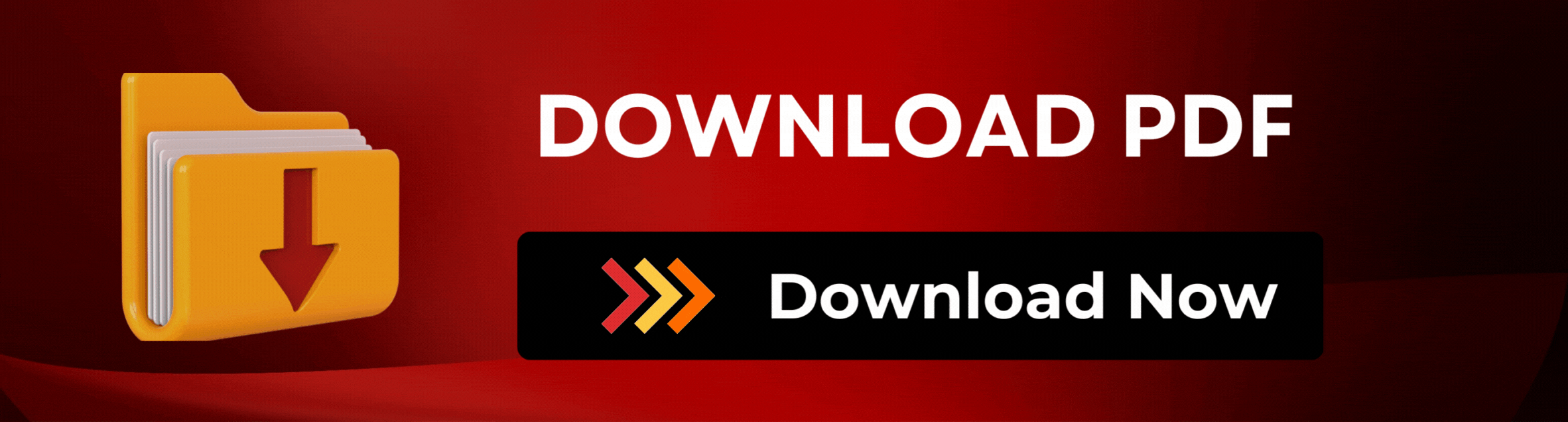