
Google Mobile Vision API – Face Detection on Android Devices
Google Mobile Vision API: Revolutionizing Face Detection on Android
In today’s digital age, the ability to detect faces using mobile devices has become increasingly crucial. One of the standout tools in this domain is the Google Mobile Vision API. This state-of-the-art technology enables Android applications to recognize and process faces in real-time, offering a seamless experience for users.
How Does It Work?
The Google Mobile Vision API is not just any face detection mobile tool; it’s a sophisticated system that offers a plethora of features. Here’s a step-by-step breakdown:
- Initiation: The user points their phone’s camera at a picture or a group of people.
- Detection: Leveraging the power of the Mobile Vision API, the system identifies faces, capturing attributes such as the degree of happiness and the openness of the left and right eyes, represented in percentages.
- Storage: Users have the option to save detected faces in either jpg or png formats. Additionally, each face can be named and stored in a database. For convenience, photos are saved in Base 64 format in the database, allowing users to revisit them later.
Key Features:

- Precision: The system boasts an impressive accuracy rate, thanks to the robustness of the Google Mobile Vision API.
- Emotion Detection: Beyond mere face detection, the system gauges the level of happiness on the face, presenting it as a percentage.
- Eye Analysis: The API provides insights into how much each eye is open, offering a unique perspective on the subject’s expression.
Advantages:
- Efficiency: Say goodbye to the tedious task of cropping pictures manually. The system handles it for you.
- High Accuracy: With the Mobile Vision API at its core, the system ensures top-notch accuracy in face detection.
- Emotion Analysis: The system doesn’t just detect faces; it offers insights into the subject’s emotions, such as the intensity of their smile.
- Detailed Eye Metrics: Users receive data on the openness of each eye, separately, in percentage terms.
Limitations:
- Camera Dependency: The system exclusively uses live camera shots, meaning it cannot tag or process images already stored on the phone.
- Face Detection, Not Recognition: It’s essential to note that while the system is adept at detecting faces, it doesn’t serve as a face recognition tool.
In conclusion, the Google Mobile Vision API is a game-changer in the realm of face detection on mobile devices. While it has its limitations, its advantages far outweigh them, making it a must-have tool for Android developers and enthusiasts alike.
Sample Code
1. Add the necessary dependencies to your build.gradle
file:
implementation 'com.google.android.gms:play-services-vision:20.1.3'
2. Add permissions to your AndroidManifest.xml
:
<uses-permission android:name="android.permission.CAMERA" />
<application>
...
<meta-data
android:name="com.google.android.gms.vision.DEPENDENCIES"
android:value="face"/>
...
</application>
3. Create a simple layout with a SurfaceView
for camera preview in activity_main.xml
:
<SurfaceView
android:id="@+id/surfaceView"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
4. Implement face detection in your MainActivity.java
:
import com.google.android.gms.vision.CameraSource;
import com.google.android.gms.vision.face.FaceDetector;
public class MainActivity extends AppCompatActivity {
private SurfaceView surfaceView;
private CameraSource cameraSource;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
surfaceView = findViewById(R.id.surfaceView);
FaceDetector faceDetector = new FaceDetector.Builder(getApplicationContext())
.setTrackingEnabled(false)
.setLandmarkType(FaceDetector.ALL_LANDMARKS)
.build();
if (!faceDetector.isOperational()) {
Toast.makeText(this, "Face Detector could not be set up on your device!", Toast.LENGTH_SHORT).show();
return;
}
cameraSource = new CameraSource.Builder(getApplicationContext(), faceDetector)
.setRequestedPreviewSize(640, 480)
.setFacing(CameraSource.CAMERA_FACING_FRONT)
.setRequestedFps(30.0f)
.build();
surfaceView.getHolder().addCallback(new SurfaceHolder.Callback() {
@Override
public void surfaceCreated(SurfaceHolder holder) {
try {
cameraSource.start(surfaceView.getHolder());
} catch (IOException e) {
e.printStackTrace();
}
}
@Override
public void surfaceChanged(SurfaceHolder holder, int format, int width, int height) {}
@Override
public void surfaceDestroyed(SurfaceHolder holder) {
cameraSource.stop();
}
});
}
}
Note: This is a basic example, and you might need to handle permissions, camera lifecycle, and other aspects for a production-ready application. Always refer to the official documentation for the most up-to-date and detailed information.
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Google Mobile Vision API – Face Detection on Android Devices PDF
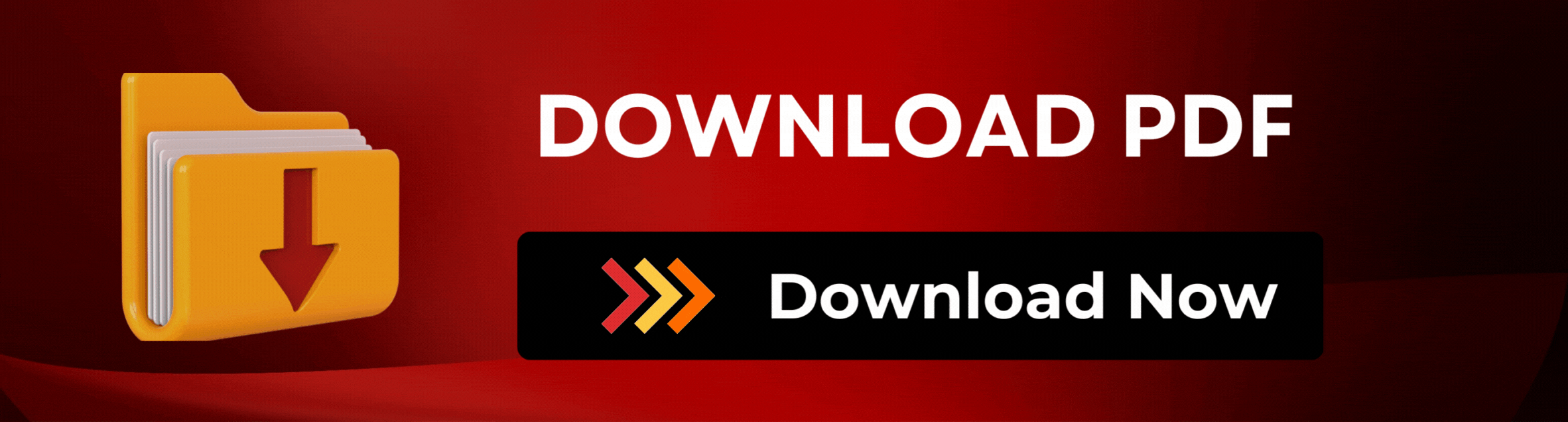