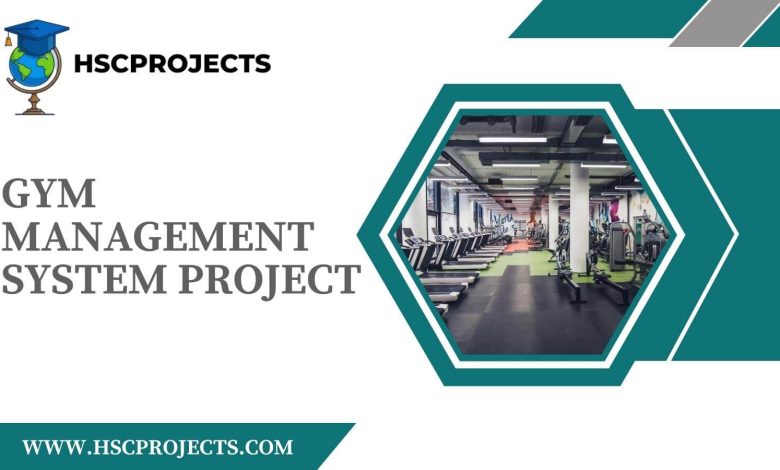
Gym Management System Project
Introduction
Maintaining a healthy lifestyle is imperative in today’s fast-paced world. However, getting the most out of a fitness club or gym often involves complex administrative processes. Our modern Gym Management System project offers a seamless solution for both gyms and members. This robust health and fitness management software allows for easy booking, electronic payments, and efficient trainer-member interactions.

Key Features of Our Gym Management System Project
The Gym Management System has three key entities: Admin, Member, and Trainer.
- Admin Functions
- Package Management: The admin can add, update, or delete fitness packages, specifying costs and discounts.
- Member Management: Admins can view, add, or delete member details and packages.
- Attendance Tracking: Admins have access to attendance data taken by trainers.
- Member Functions
- Profile Management: Members can log in to view their profiles and see a list of available trainers.
- Payment: Payments for gym packages and trainer sessions can be made through card details.
- Feedback: Members can offer feedback on their trainers to improve the quality of the service.
- Trainer Functions
- Profile Setup: Trainers can set up their profiles with credentials.
- Attendance: Trainers are responsible for tracking daily attendance of their assigned members.
- Password Security: Trainers can update their passwords to maintain account security.
Advantages of the Gym Management System
- Simplifies scheduling and booking of personal training sessions.
- Accessible anytime, anywhere with an internet connection.
- Increases the gym’s revenue and personal training sessions.
Disadvantages of the Gym Management System
- Requires a constant internet connection for optimal functionality.
- Users must enter data carefully to avoid errors.
Gym Management System Project in PHP
For those interested in PHP-based solutions, our gym management system project in PHP offers a flexible, easy-to-implement framework. You can download the gym management system project report for a detailed guide on the setup and operation.
Conclusion
Whether you’re a gym owner or a fitness enthusiast, our Gym Management System is designed to streamline operations and provide a more satisfactory experience for everyone involved. The platform balances user-friendliness with advanced features, making it a reliable choice for any health club or fitness center.
Sample Code
class GymSystem:
def __init__(self):
self.members = {}
self.trainers = {}
self.packages = {}
def add_member(self, id, name):
self.members[id] = {'name': name, 'package': None}
def add_trainer(self, id, name):
self.trainers[id] = {'name': name}
def add_package(self, id, cost):
self.packages[id] = {'cost': cost}
def assign_package_to_member(self, member_id, package_id):
if member_id in self.members and package_id in self.packages:
self.members[member_id]['package'] = package_id
def show_members(self):
for id, details in self.members.items():
print(f"ID: {id}, Name: {details['name']}, Package: {details['package']}")
def show_trainers(self):
for id, details in self.trainers.items():
print(f"ID: {id}, Name: {details['name']}")
def show_packages(self):
for id, details in self.packages.items():
print(f"Package ID: {id}, Cost: {details['cost']}")
if __name__ == "__main__":
gym = GymSystem()
while True:
print("\n1. Add Member")
print("2. Add Trainer")
print("3. Add Package")
print("4. Assign Package to Member")
print("5. Show Members")
print("6. Show Trainers")
print("7. Show Packages")
print("8. Quit")
choice = int(input("\nEnter your choice: "))
if choice == 1:
id = input("Enter Member ID: ")
name = input("Enter Member Name: ")
gym.add_member(id, name)
elif choice == 2:
id = input("Enter Trainer ID: ")
name = input("Enter Trainer Name: ")
gym.add_trainer(id, name)
elif choice == 3:
id = input("Enter Package ID: ")
cost = input("Enter Package Cost: ")
gym.add_package(id, cost)
elif choice == 4:
member_id = input("Enter Member ID: ")
package_id = input("Enter Package ID: ")
gym.assign_package_to_member(member_id, package_id)
elif choice == 5:
gym.show_members()
elif choice == 6:
gym.show_trainers()
elif choice == 7:
gym.show_packages()
elif choice == 8:
break
else:
print("Invalid choice. Please try again.")
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Gym Management System Project PDF
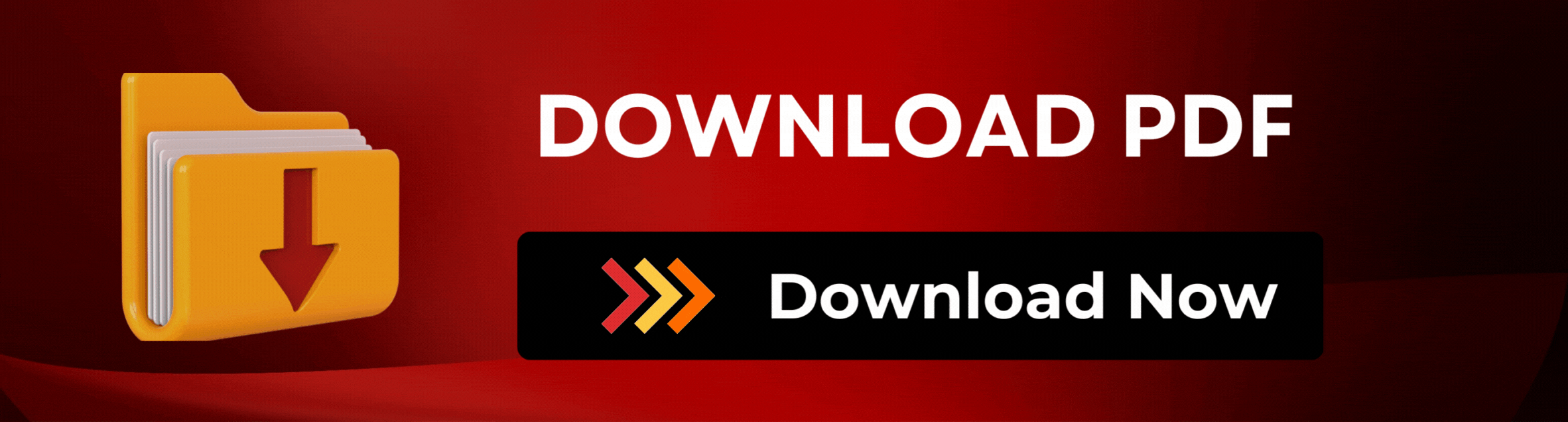