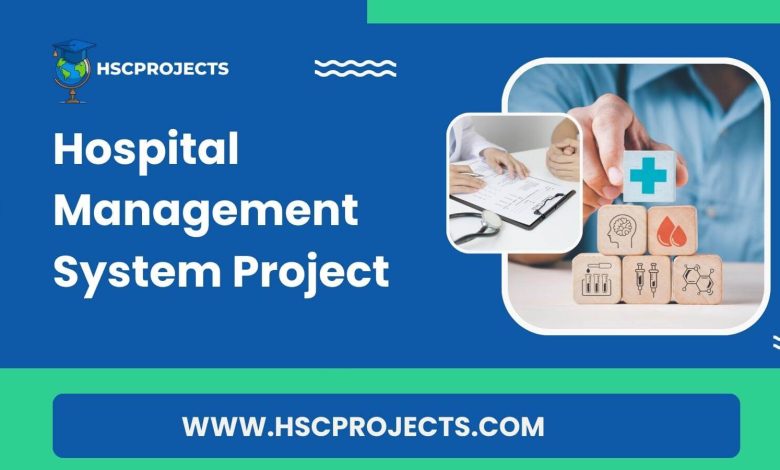
Hospital Management System Project
Introduction
The healthcare industry has seen a significant transformation with the advent of Hospital Management Systems (HMS). These systems serve as a one-stop solution for managing various aspects of hospital administration, from patient care to internal operations. This article aims to provide an in-depth understanding of what a hospital management system is, its types, uses, and much more.

What is a Hospital Management System?
A Hospital Management System is a software solution designed to manage and streamline the complex tasks involved in running a hospital. It serves as a centralized platform where administrators can store and manage various types of data, including patient information, bed availability, and medical records.
Key Features
- Patient Data Management: Stores comprehensive patient details, including name, contact information, and health parameters.
- Bed Allocation: Allows administrators to check bed availability in real-time and allocate them as per patient needs.
- Billing and Records: Automates the billing process and eliminates the need for paper records.
- Doctor’s Dashboard: Enables doctors to access their patients’ treatment records easily.
Types of Hospital Management Systems
- Database Management Systems (DBMS): Primarily focuses on storing large sets of data securely.
- Online Hospital Management Systems: Provides cloud-based solutions for remote access.
- Mini Projects: Smaller systems tailored for clinics or small healthcare facilities.
Advantages
- Automation: Replaces manual methods, making the system more efficient.
- Real-Time Access: Facilitates immediate bed allocation and patient data retrieval.
- Cost-Effective: Saves time, effort, and resources, thereby reducing operational costs.
Disadvantages
- Database Requirements: Needs a robust database system.
- Manual Updates: Administrators must keep the system updated manually.
Future Scope
With advancements in technology, we can expect more integrated and AI-driven hospital management systems that will further revolutionize healthcare.
Conclusion
Hospital Management Systems have become an integral part of modern healthcare facilities. They not only make the management tasks easier but also enhance the quality of healthcare services provided.
Sample Code
install Flask if you haven’t already:
pip install Flask
basic outline of the code:
from flask import Flask, request, jsonify
app = Flask(__name__)
# Sample database
patients = {}
beds = {'ICU': 10, 'General': 20}
@app.route('/add_patient', methods=['POST'])
def add_patient():
patient_data = request.json
patient_id = patient_data['id']
if patient_id in patients:
return jsonify({'message': 'Patient already exists'}), 400
patients[patient_id] = patient_data
return jsonify({'message': 'Patient added successfully'}), 201
@app.route('/allocate_bed', methods=['POST'])
def allocate_bed():
data = request.json
patient_id = data['id']
bed_type = data['bed_type']
if bed_type not in beds:
return jsonify({'message': 'Invalid bed type'}), 400
if beds[bed_type] <= 0:
return jsonify({'message': 'No beds available'}), 400
if patient_id not in patients:
return jsonify({'message': 'Patient does not exist'}), 400
beds[bed_type] -= 1
patients[patient_id]['bed_type'] = bed_type
return jsonify({'message': f'Bed allocated in {bed_type}'}), 200
@app.route('/get_patient/<int:patient_id>', methods=['GET'])
def get_patient(patient_id):
if patient_id not in patients:
return jsonify({'message': 'Patient not found'}), 404
return jsonify(patients[patient_id]), 200
if __name__ == '__main__':
app.run(debug=True)
To add a patient:
curl --request POST \
--url http://127.0.0.1:5000/add_patient \
--header 'Content-Type: application/json' \
--data '{
"id": 1,
"name": "John Doe",
"age": 30,
"address": "123 Main St"
}'
To allocate a bed:
curl --request POST \
--url http://127.0.0.1:5000/allocate_bed \
--header 'Content-Type: application/json' \
--data '{
"id": 1,
"bed_type": "ICU"
}'
To get patient details:
curl --request GET \
--url http://127.0.0.1:5000/get_patient/1
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Hospital Management System Project PDF
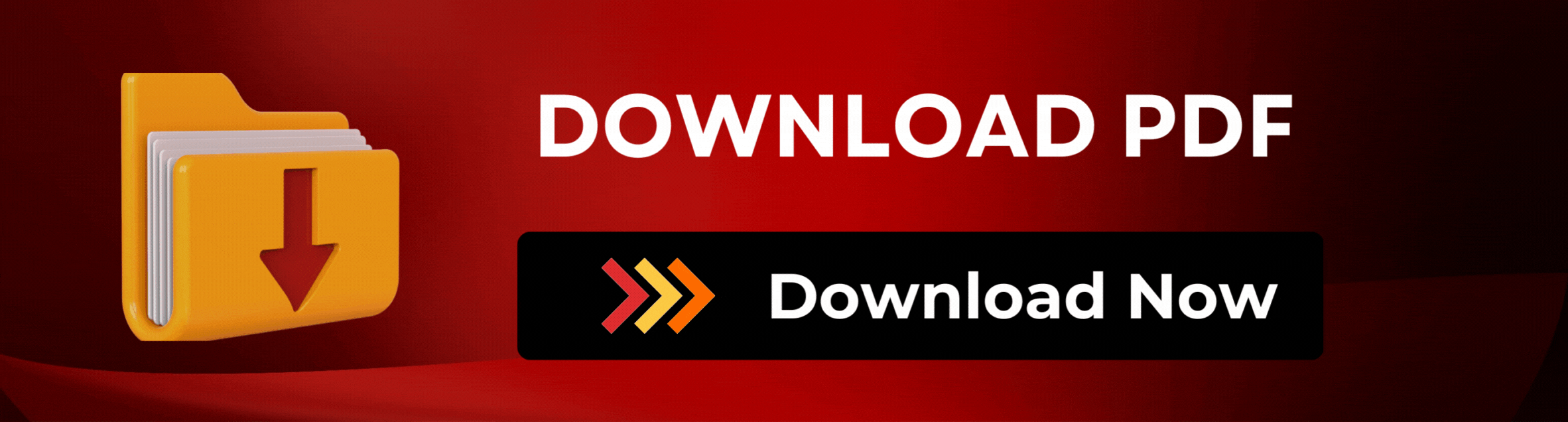