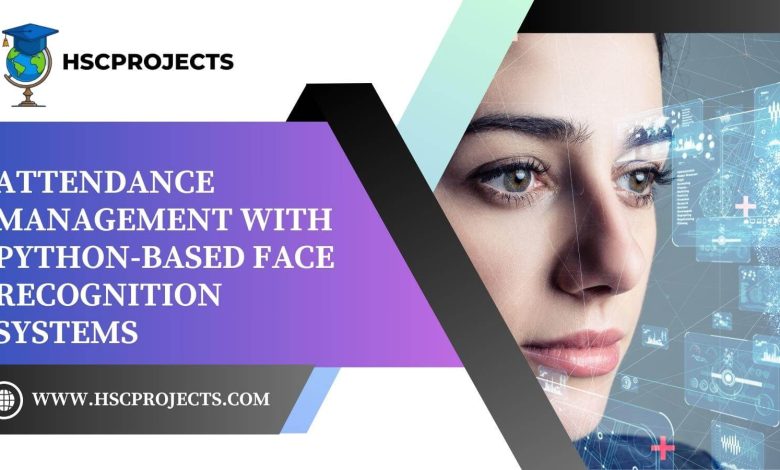
Attendance Management with Python-based Face Recognition Systems
Introduction
Attendance management has always been a challenging task for organizations. Traditional methods like manual entry are prone to errors and fraud. However, the face recognition attendance system using Python offers a compelling, efficient, and user-friendly solution. This technology leverages advanced algorithms to identify employees uniquely and accurately.

The Mechanism Behind Face Recognition Attendance
Face recognition serves as a biometric technique that relies on facial characteristics to verify identity. The technology embedded in the attendance system uses Python-based libraries such as OpenCV, Dlib, and Face-Recognition to accurately detect and record an employee’s presence. These libraries provide a robust foundation for implementing the face recognition features effectively.
System Architecture
The front-end of this face recognition-based attendance system is developed using HTML, CSS, and JavaScript. Meanwhile, the back-end uses Python and runs on the Django framework. MySQL serves as the database for storing attendance records and other essential data.
Advantages of Face Recognition Attendance System
- Ease of Maintenance: The system is designed to be straightforward, thus requiring minimal maintenance.
- User-Friendly Interface: A simple interface ensures that employees can interact with the system without any complications.
- Accuracy: The biometric face recognition method eliminates the risk of proxy attendance and errors.
- Automated Process: No human intervention is required, making the system entirely automatic and error-free.
How To Implement
The face recognition attendance system python source code can be tailored to suit an organization’s specific needs. To obtain the face recognition based attendance system using Python project report, one can consult documentation and online tutorials that explain each stage of development comprehensively.
Conclusion
Implementing a face recognition attendance system using Python can significantly improve the efficiency of attendance management in an organization. By leveraging Python’s powerful libraries and frameworks, organizations can modernize their attendance systems, thereby saving resources and eliminating human error.
Sample Code
import face_recognition
import cv2
import csv
import datetime
# Initialize webcam
video_capture = cv2.VideoCapture(0)
# Load a sample picture and learn how to recognize it.
known_face_encoding = face_recognition.face_encodings(face_recognition.load_image_file("employee.jpg"))[0]
known_face_names = ["Employee Name"]
# Initialize variables
face_locations = []
face_encodings = []
face_names = []
process_this_frame = True
# Open a CSV file to save attendance data
with open('attendance.csv', 'w', newline='') as csvfile:
fieldnames = ['Name', 'Time']
writer = csv.DictWriter(csvfile, fieldnames=fieldnames)
writer.writeheader()
while True:
ret, frame = video_capture.read()
# Resize frame for faster processing
small_frame = cv2.resize(frame, (0, 0), fx=0.25, fy=0.25)
# Find all the faces and face encodings in the frame
face_locations = face_recognition.face_locations(small_frame)
face_encodings = face_recognition.face_encodings(small_frame, face_locations)
face_names = []
for face_encoding in face_encodings:
matches = face_recognition.compare_faces([known_face_encoding], face_encoding)
name = "Unknown"
if True in matches:
name = known_face_names[matches.index(True)]
face_names.append(name)
writer.writerow({'Name': name, 'Time': str(datetime.datetime.now())})
# Display the results
for (top, right, bottom, left), name in zip(face_locations, face_names):
top *= 4
right *= 4
bottom *= 4
left *= 4
cv2.rectangle(frame, (left, top), (right, bottom), (0, 0, 255), 2)
font = cv2.FONT_HERSHEY_DUPLEX
cv2.putText(frame, name, (left + 6, bottom - 6), font, 0.5, (255, 255, 255), 1)
cv2.imshow('Video', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
video_capture.release()
cv2.destroyAllWindows()
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Subscribed? Click on Confirm
Download Attendance Management with Python-based Face Recognition Systems PDF
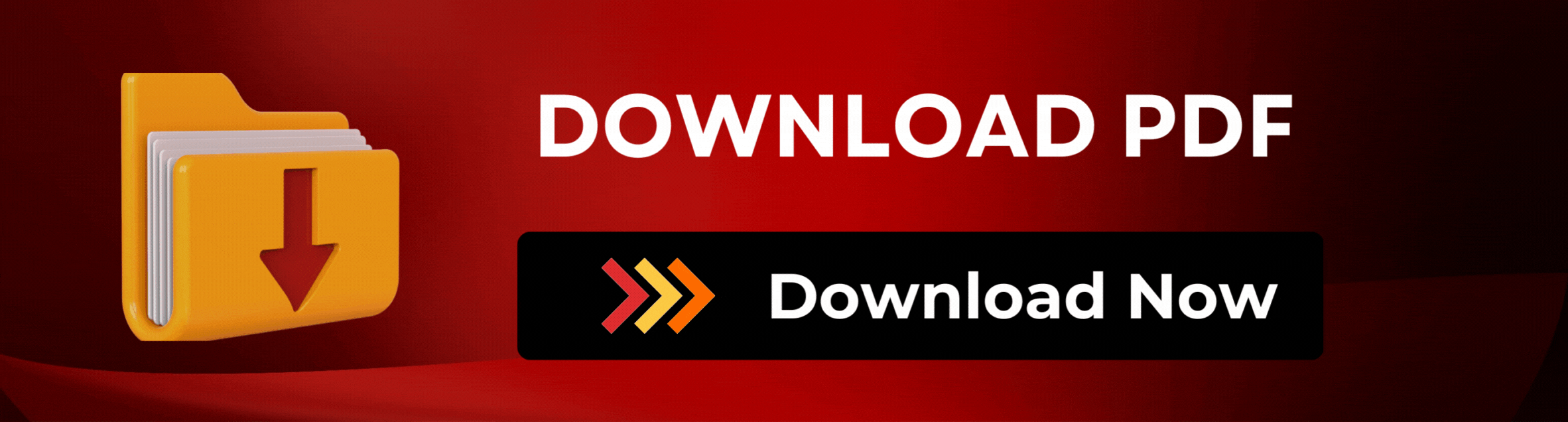