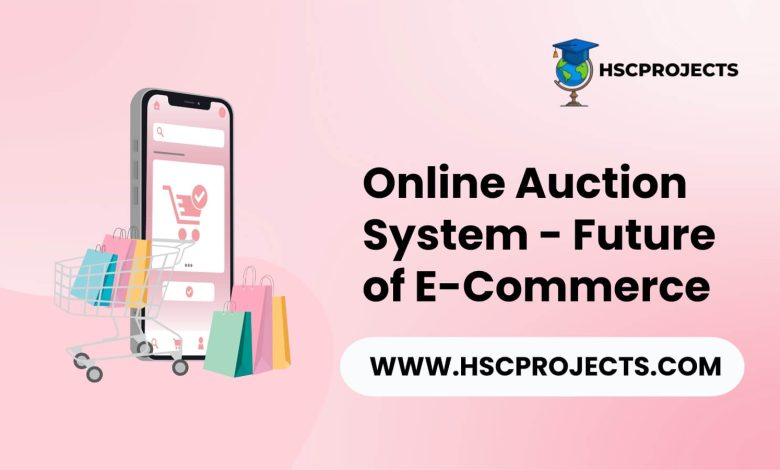
Online Auction System – Future of E-Commerce
Introduction
The digital age has brought a plethora of changes to various industries, and the auction world is no exception. Online auction systems have revolutionized the way we buy and sell items, offering a convenient and efficient alternative to traditional auction houses. This article delves into the various components of an online auction system project, its advantages, and some of its limitations.
Core Components of an Online Auction System
An online auction system typically consists of three main modules:
- Bidder’s Dashboard: This is where the magic happens for buyers. After logging in, bidders can browse through a list of products available for auction. They can place their bids and track the status of their ongoing bids.
- Seller’s Portal: Sellers can list their products for auction through this module. They can set a starting price, upload product images, and provide detailed descriptions to attract potential bidders.
- Admin Control: The admin module is designed to oversee the entire auction process. Administrators can remove fake or inappropriate listings and generate reports to analyze user activity and auction trends.
- Notification System: Both winners and losers of the auction receive SMS notifications, updating them on the outcome of their bids.
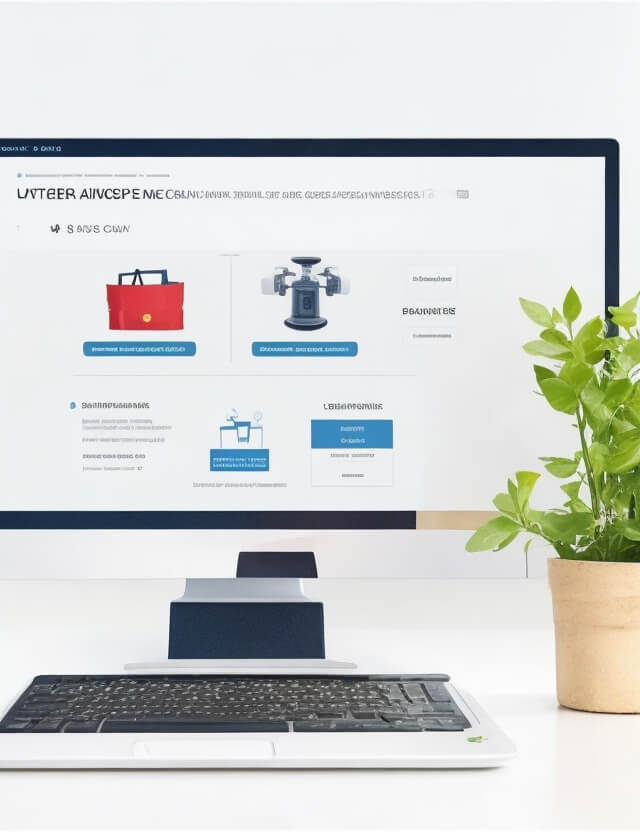
Advantages of Online Auction Systems
- Global Reach: One of the most significant benefits is the ability to attract bidders from around the world.
- Convenience: Bidders can participate at any time, from anywhere, without the constraints of a physical location.
- Transparency: Online systems often provide detailed histories of bids, making the process transparent and fair.
Limitations
- Lack of Physical Inspection: One of the main drawbacks is that bidders cannot physically inspect the items before bidding.
Future of Online Auctions in E-Commerce
With advancements in technology, online auction platforms are likely to become even more secure, user-friendly, and feature-rich. Features like virtual reality could even allow bidders to inspect items “virtually” before making a bid.
Conclusion
Online auction systems have significantly impacted the way we think about buying and selling goods. They offer a level of convenience and global reach that traditional auction systems can’t match. However, like any system, they come with their own set of challenges and limitations. As technology advances, we can only expect these platforms to evolve and better serve both buyers and sellers in the e-commerce landscape.
Sample Code
First, install Flask if you haven’t:
pip install Flask
Here’s a basic Flask app to get you started:
from flask import Flask, request, jsonify
app = Flask(__name__)
# In-memory database
products = {}
bids = {}
@app.route('/add_product', methods=['POST'])
def add_product():
data = request.json
product_id = data.get('product_id')
product_name = data.get('product_name')
starting_price = data.get('starting_price')
products[product_id] = {
'product_name': product_name,
'starting_price': starting_price,
'highest_bid': starting_price
}
return jsonify({"message": "Product added successfully"}), 200
@app.route('/place_bid', methods=['POST'])
def place_bid():
data = request.json
product_id = data.get('product_id')
bidder_id = data.get('bidder_id')
bid_amount = data.get('bid_amount')
product = products.get(product_id)
if not product:
return jsonify({"message": "Product not found"}), 404
if bid_amount <= product['highest_bid']:
return jsonify({"message": "Bid must be higher than the current highest bid"}), 400
product['highest_bid'] = bid_amount
bids[bidder_id] = {'product_id': product_id, 'bid_amount': bid_amount}
return jsonify({"message": "Bid placed successfully"}), 200
@app.route('/get_highest_bid/<product_id>', methods=['GET'])
def get_highest_bid(product_id):
product = products.get(product_id)
if not product:
return jsonify({"message": "Product not found"}), 404
return jsonify({"highest_bid": product['highest_bid']}), 200
if __name__ == '__main__':
app.run(debug=True)
To run the code, save it in a file (e.g., app.py
) and run it. You can then use Postman or curl to test the API endpoints.
Here’s how you can add a product:
curl --request POST \
--url http://127.0.0.1:5000/add_product \
--header 'Content-Type: application/json' \
--data '{
"product_id": "1",
"product_name": "Laptop",
"starting_price": 500
}'
Place a bid:
curl --request POST \
--url http://127.0.0.1:5000/place_bid \
--header 'Content-Type: application/json' \
--data '{
"product_id": "1",
"bidder_id": "user1",
"bid_amount": 600
}'
Get the highest bid for a product:
curl --request GET \
--url http://127.0.0.1:5000/get_highest_bid/1
This is a very basic example and lacks many features like authentication, real-time updates, and database storage. You would typically use a frontend framework like React or Angular along with a real database for a production application.
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Online Auction System – Future of E-Commerce PDF
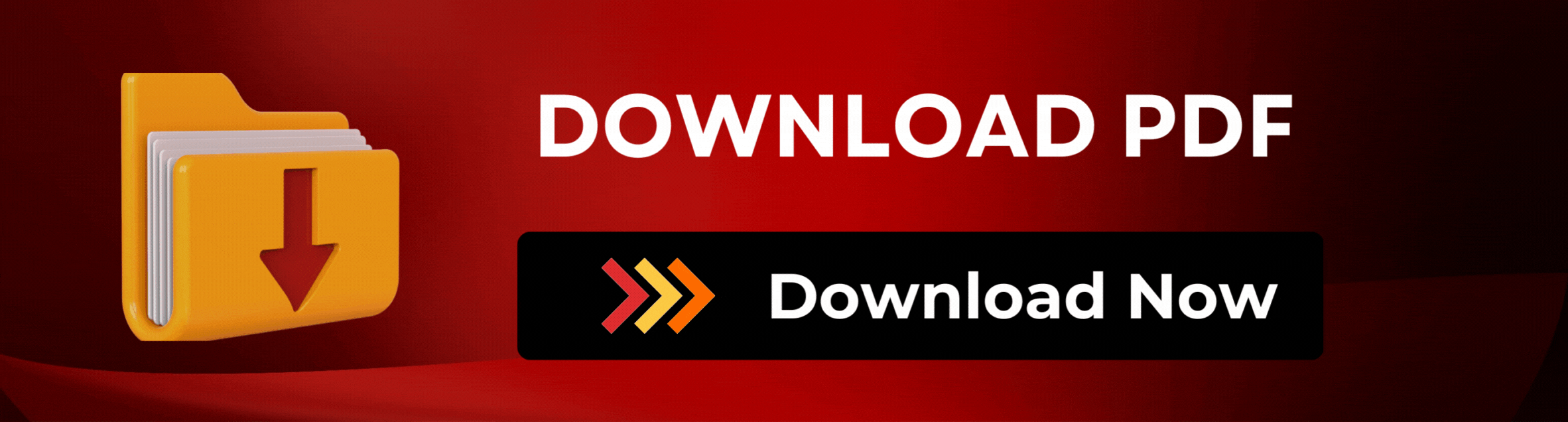