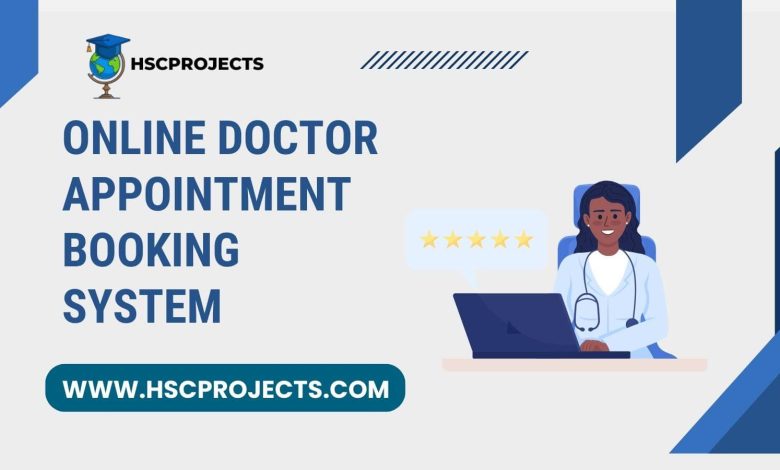
Online Doctor Appointment Booking System
Introduction
The healthcare industry has been undergoing a digital transformation, and one of the most notable changes is the shift towards online doctor appointment systems. Gone are the days when booking a doctor’s appointment meant long waiting times and manual scheduling. Today, doctor appointment booking systems offer a seamless, user-friendly experience for both healthcare providers and patients. This article delves into the intricacies of these systems, their advantages, and how they are making healthcare more accessible.
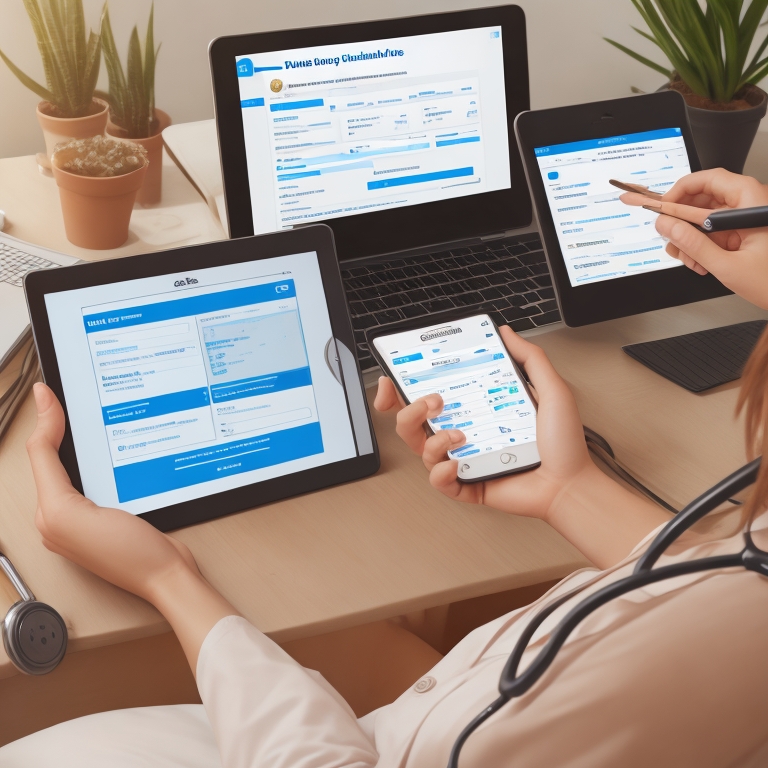
What is an Online Doctor Appointment System?
An online doctor appointment system is a web application or mobile app that allows patients to book, reschedule, or cancel appointments with healthcare providers. These systems often come with additional features like appointment reminders, online payment options, and even telehealth consultations.
Key Features
- User-Friendly Interface: A simple and intuitive interface ensures that even individuals who are not tech-savvy can book appointments easily.
- Flexible Scheduling: Patients can view various booking slots and choose a date and time that suits them best.
- Appointment Management: Doctors and administrative staff can easily manage appointments, reducing the risk of double bookings or scheduling conflicts.
- Monthly Earnings Report: An added feature for healthcare providers is the ability to track monthly earnings through the system.
Advantages of Online Doctor Appointment Systems
- Reduced Waiting Time: One of the most significant benefits is the reduction in the waiting time for patients.
- Convenience: Patients can book appointments at any time, from anywhere, making the process extremely convenient.
- Efficient Use of Resources: Automating the appointment booking process allows healthcare providers to better manage their time and resources.
Disadvantages
- Internet Dependency: The system requires an active internet connection to function.
- Database Requirements: A large database is necessary to manage the appointments effectively, which could be a limitation for smaller practices.
Conclusion
Online doctor appointment booking systems are not just a trend but a necessity in today’s fast-paced world. They offer numerous advantages like convenience, efficiency, and better resource management for healthcare providers. However, it’s essential to choose a system that fits your specific needs and complies with healthcare regulations.
Sample Code
First, install Flask if you haven’t already:
pip install Flask
from flask import Flask, request, jsonify
app = Flask(__name__)
# Sample database of doctors and appointments
doctors = [
{'id': 1, 'name': 'Dr. Smith', 'specialization': 'Cardiology'},
{'id': 2, 'name': 'Dr. Johnson', 'specialization': 'Neurology'}
]
appointments = []
@app.route('/doctors', methods=['GET'])
def get_doctors():
return jsonify({'doctors': doctors})
@app.route('/appointments', methods=['GET'])
def get_appointments():
return jsonify({'appointments': appointments})
@app.route('/book', methods=['POST'])
def book_appointment():
if not request.json or not 'doctor_id' in request.json or not 'time' in request.json:
return jsonify({'error': 'Bad Request'}), 400
appointment = {
'id': len(appointments) + 1,
'doctor_id': request.json['doctor_id'],
'time': request.json['time']
}
appointments.append(appointment)
return jsonify({'appointment': appointment}), 201
if __name__ == '__main__':
app.run(debug=True)
To run the code, save it in a file (e.g., app.py
) and run it. You can then use Postman or curl to test the API endpoints.
- To view doctors:
GET http://127.0.0.1:5000/doctors
- To view appointments:
GET http://127.0.0.1:5000/appointments
- To book an appointment:
POST http://127.0.0.1:5000/book
with JSON data like{"doctor_id": 1, "time": "2021-09-05T14:30:00"}
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Subscribed? Click on Confirm
Download Online Doctor Appointment Booking System PDF
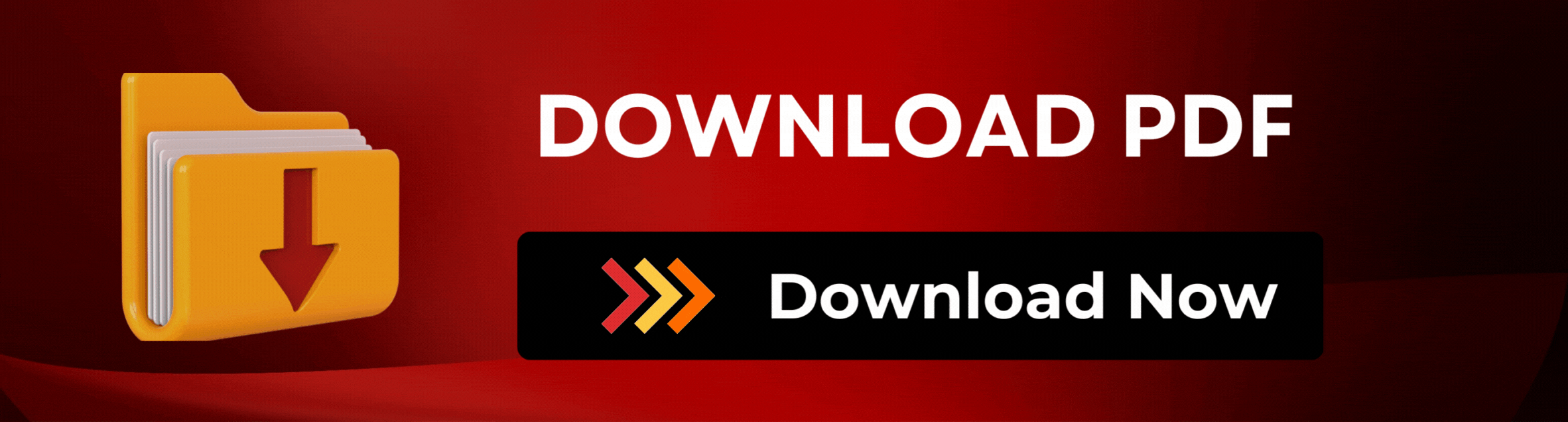