
Vehicle Number Plate Recognition Using Android Apps
Introduction
Vehicle Number Plate Recognition is an indispensable technology for modern law enforcement and traffic management systems. Whether it’s identifying traffic violators or controlling access to high-security areas, the role of number plate identification has never been more vital. This article delves into how Android apps, equipped with number plate reader capabilities, are leading the charge in this domain.

Core Components of Vehicle Number Plate Recognition
The technology behind vehicle number plate recognition can be broken down into two main components:
- Vehicle Number Plate Extraction: In this stage, the system scans the incoming image or video stream and identifies the part of the image where the number plate is located. It then isolates the number plate for further processing.
- Optical Character Recognition (OCR): Once the number plate is extracted, the individual characters on the plate are segmented and processed using OCR algorithms. This converts the visual data into an encoded text string for identification.
Applications and Use-Cases
- Toll Collection: Automated toll plazas use number plate scanner technology to speed up the toll collection process, significantly reducing human error.
- Parking Management: Number plate recognition aids in automatic slot assignment and billing, making the process more efficient.
- High-security Areas: In military installations or other secure areas, plate recognizer technology ensures that only authorized vehicles gain access.
- Traffic Law Enforcement: The number plate recognition system can flag or alert authorities about vehicles that are on the blacklist for any legal or traffic violations.
- GPS Number Plate Tracking: With real-time GPS data, the system can track vehicle movement, aiding in theft recovery and monitoring.
Advantages
- Provides real-time user and vehicle details through vehicle number app.
- Enables authorities to flag or blacklist vehicles not adhering to traffic rules.
Disadvantages
- An active internet connection is required for real-time data processing.
- Inaccurate results may occur if the data is not input correctly.
Future Trends: AR in Number Plate Recognition
Augmented Reality (AR) is set to play a transformative role in number plate recognition. By overlaying data on the real-world view, it can provide instantaneous, valuable information for law enforcement agencies.
Conclusion
As Android continues to dominate the mobile landscape, apps equipped with number plate recognition capabilities are poised to become ever more crucial. From simplifying toll collection to enhancing national security, the potential applications are endless.
Sample Code
‘build.gradle
‘:
dependencies {
implementation 'org.opencv:opencv-android:4.5.1'
implementation 'com.rmtheis:tess-two:9.1.0'
}
Initialize OpenCV in your Main Activity
import org.opencv.android.OpenCVLoader;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
if (!OpenCVLoader.initDebug()) {
Log.e(this.getClass().getSimpleName(), "OpenCV initialization failed.");
} else {
Log.d(this.getClass().getSimpleName(), "OpenCV initialization succeeded.");
}
}
Capture Image and Detect Number Plate
import org.opencv.core.Mat;
import org.opencv.core.MatOfRect;
import org.opencv.core.Rect;
import org.opencv.core.Size;
import org.opencv.imgproc.Imgproc;
import org.opencv.objdetect.CascadeClassifier;
public Mat detectNumberPlate(Mat inputFrame) {
Mat grayFrame = new Mat();
Imgproc.cvtColor(inputFrame, grayFrame, Imgproc.COLOR_BGR2GRAY);
CascadeClassifier numberPlateDetector = new CascadeClassifier("path/to/haarcascade_russian_plate_number.xml");
MatOfRect numberPlates = new MatOfRect();
numberPlateDetector.detectMultiScale(grayFrame, numberPlates);
for (Rect rect : numberPlates.toArray()) {
Imgproc.rectangle(inputFrame, rect.tl(), rect.br(), new Scalar(0, 255, 0), 3);
}
return inputFrame;
}
Use Tesseract for OCR
import com.googlecode.tesseract.android.TessBaseAPI;
public String recognizeText(Mat inputFrame, Rect numberPlateRect) {
TessBaseAPI tessBaseAPI = new TessBaseAPI();
tessBaseAPI.init("path/to/tessdata/", "eng");
Mat plateMat = inputFrame.submat(numberPlateRect);
Bitmap plateBitmap = Bitmap.createBitmap(plateMat.cols(), plateMat.rows(), Bitmap.Config.ARGB_8888);
Utils.matToBitmap(plateMat, plateBitmap);
tessBaseAPI.setImage(plateBitmap);
String recognizedText = tessBaseAPI.getUTF8Text();
tessBaseAPI.end();
return recognizedText;
}
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Vehicle Number Plate Recognition Using Android Apps PDF
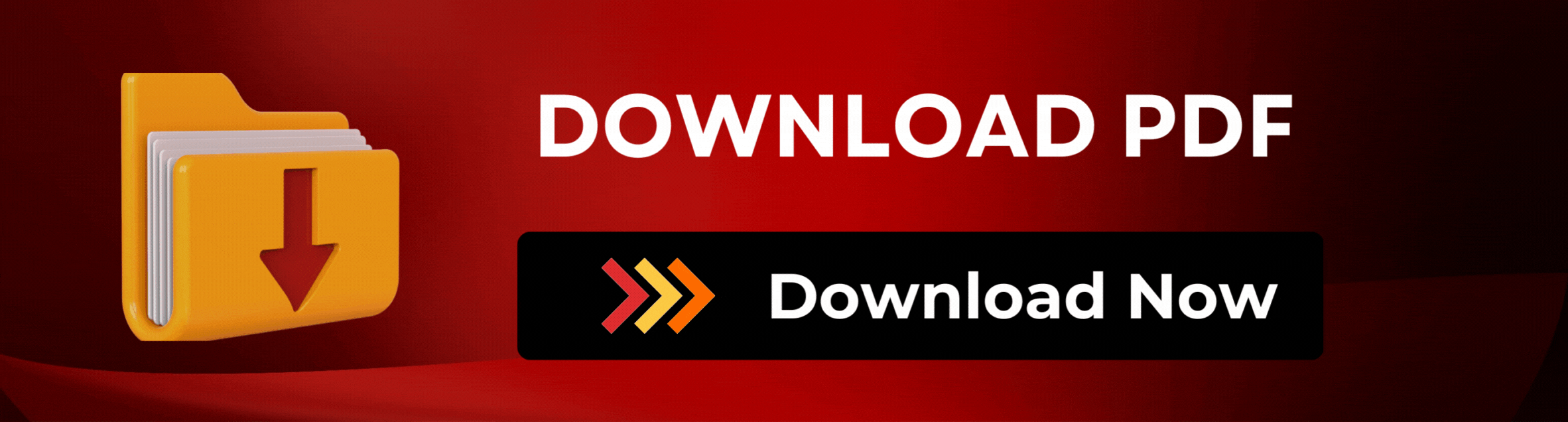