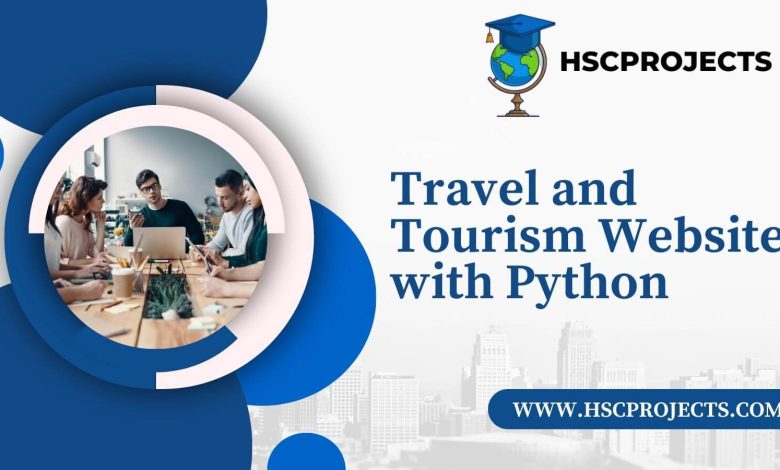
Travel and Tourism Website with Python
Introduction
The travel and tourism industry has been revolutionized by the advent of technology. Gone are the days of tedious planning, as today’s travelers demand convenience and efficiency. In this context, Python-based tourism website projects are reshaping how we think about travel planning.
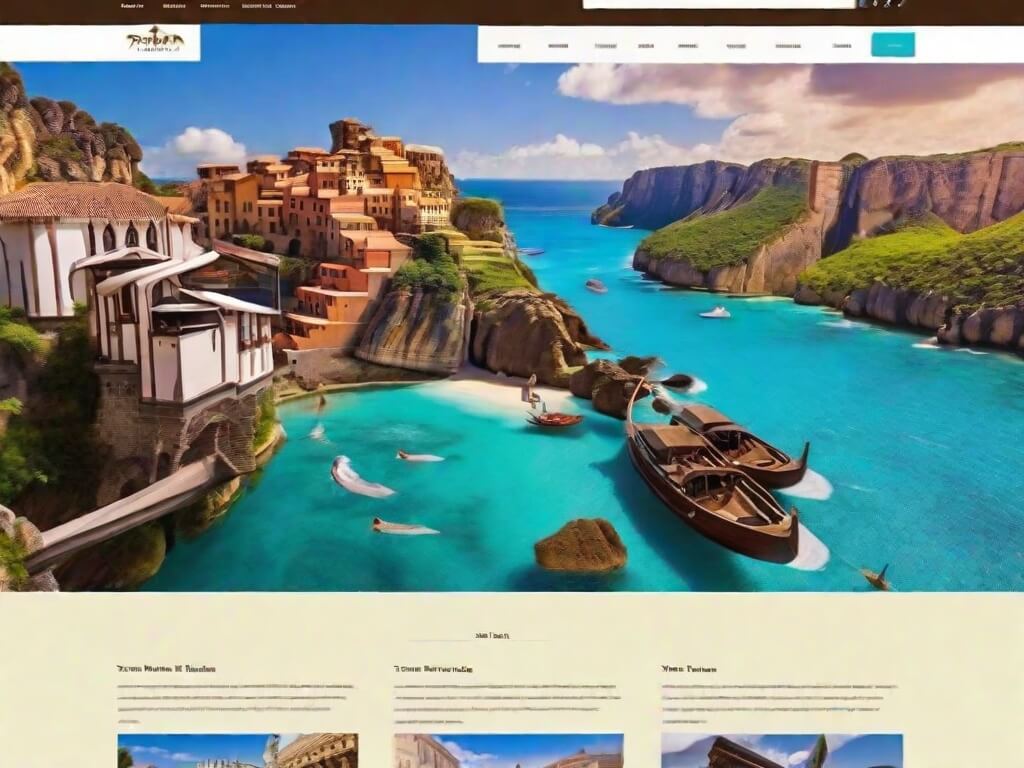
Why Python for a Travel and Tourism Website?
Python, when coupled with Django, offers a robust foundation for creating a versatile travel and tourism project. Not only does it enable quick development, but its libraries and frameworks also provide a multitude of functionalities to facilitate real-time communication and centralized data handling.
User and Admin Roles
The system is designed with two primary entities: the User and the Admin.
- Admin: After successful login, the admin gains complete control over the system. They can manage flights, hotels, and even view user activity, ensuring optimal operational efficiency.
- User: A user-friendly interface with a built-in chatbot guides users in booking hotels, flights, and travel packages. The system simplifies the process, making it easy even for people who are not tech-savvy.
Features and Advantages
- Interstation Communication: Real-time communication between different segments of the website.
- Centralized Data: All data is stored centrally, ensuring consistency and reliability.
- Time and Cost-Efficiency: The system reduces the time consumed in booking and lowers the cost by eliminating middlemen.
- Computerized Record-Keeping: Digital record-keeping minimizes manual errors and enhances efficiency.
- User-Friendly Chatbot: Provides instant assistance, making the user experience pleasant and efficient.
Building Blocks of the System
Creating such an advanced system requires a comprehensive set of tools and technologies. Django serves as the backbone, while Python’s various libraries offer functionalities like real-time chat, data storage, and more. These are complemented by HTML, CSS, and JavaScript, which take care of the frontend.
Conclusion
Travel and tourism are sectors ripe for disruption through technology. A Python-based website not only meets the demands of modern users but also offers scalable solutions for the future. From reducing costs to enhancing operational efficiency, the advantages are numerous.
Sample Code
pip install django
Create a new Django project and a new app.
django-admin startproject TravelTourism
cd TravelTourism
django-admin startapp main
Register the app in ‘settings.py
‘
# settings.py
INSTALLED_APPS = [
# ... other apps
'main',
]
Create models
# main/models.py
from django.db import models
class User(models.Model):
username = models.CharField(max_length=50)
email = models.EmailField()
class Flight(models.Model):
flight_no = models.CharField(max_length=20)
source = models.CharField(max_length=50)
destination = models.CharField(max_length=50)
price = models.FloatField()
class Hotel(models.Model):
name = models.CharField(max_length=50)
location = models.CharField(max_length=50)
price_per_night = models.FloatField()
Run the following command to apply these changes to the database:
python manage.py makemigrations
python manage.py migrate
Create Views
# main/views.py
from django.shortcuts import render
from .models import Flight, Hotel
def home(request):
return render(request, 'home.html')
def flight_list(request):
flights = Flight.objects.all()
return render(request, 'flights.html', {'flights': flights})
def hotel_list(request):
hotels = Hotel.objects.all()
return render(request, 'hotels.html', {'hotels': hotels})
URL Mapping
# main/urls.py
from django.urls import path
from . import views
urlpatterns = [
path('', views.home, name='home'),
path('flights/', views.flight_list, name='flight_list'),
path('hotels/', views.hotel_list, name='hotel_list'),
]
Make sure to include these in the project’s ‘urls.py
‘.
# TravelTourism/urls.py
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('', include('main.urls')),
]
HTML Templates
Create HTML templates (‘home.html
‘, ‘flights.html
‘, ‘hotels.html
‘) under a ‘templates
‘ folder in your ‘main
‘ app to display the data.
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Travel and Tourism Website with Python PDF
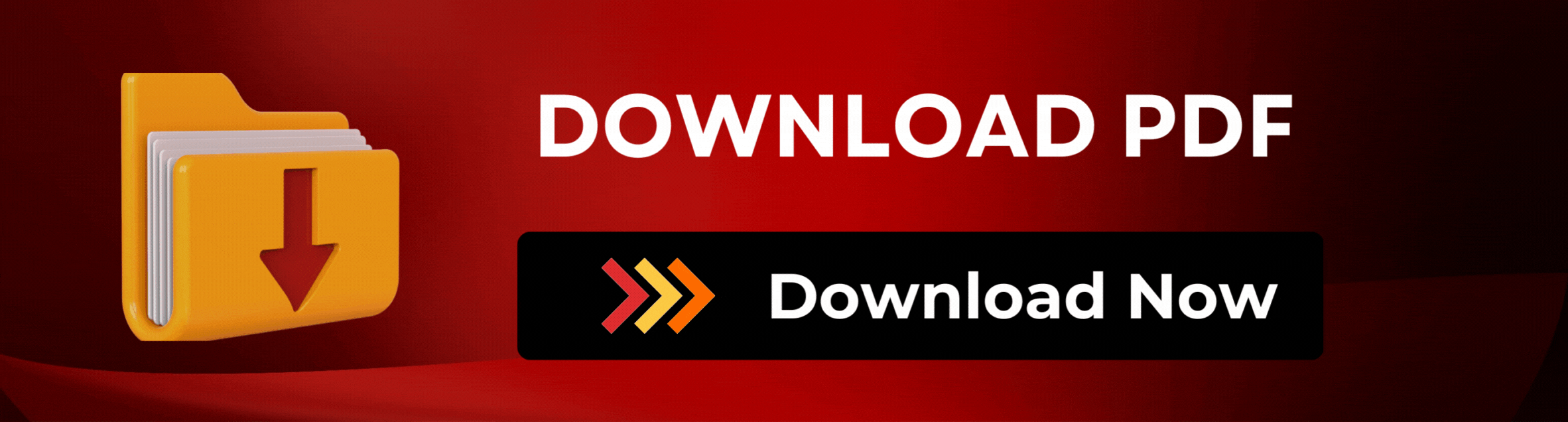