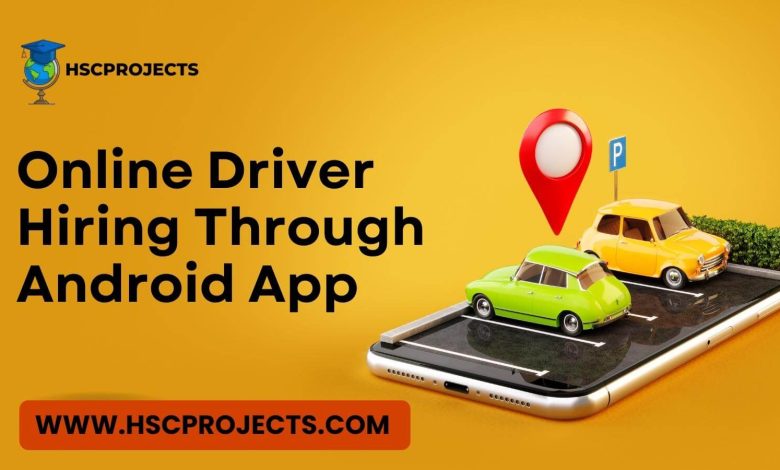
Online Driver Hiring Through Android App
Introduction
Owning a vehicle is one thing, but driving it is another. For those who prefer the comfort of being driven, hiring a skilled and experienced driver is essential. This article explores the features and benefits of an Android-based driver hiring app that aims to make the process of finding and booking drivers as seamless as possible.
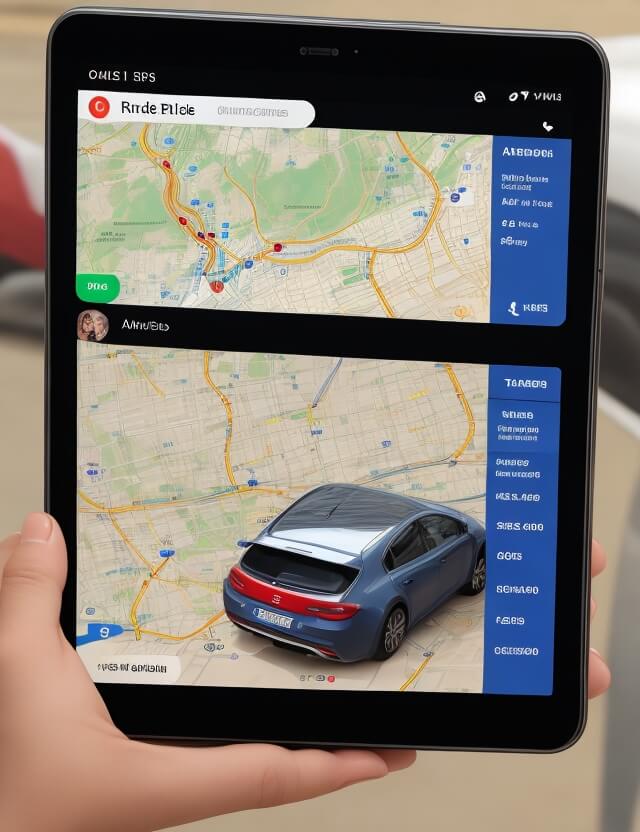
Why an Android Driver Hiring App?
Android smartphones are ubiquitous, making them the perfect platform for a driver booking app. With just a few taps, users can find, book, and even track drivers for their rides. The app offers a user-friendly interface for both drivers and those seeking their services.
How It Works
- User Registration: Both drivers and users need to register on the app.
- Driver Profile: Drivers can set up their profiles, showcasing their experience and uploading necessary documents.
- Ride Details: Both parties can view details of upcoming, completed, and canceled rides.
- Search and Book: Users can search for drivers based on various criteria like experience, vehicle type, and ratings.
- Real-Time Tracking: Users can track the driver’s location during the ride.
- Ratings and Reviews: After the ride, users can rate the driver, which helps in maintaining the quality of service.
Advantages
- Tailored Choices: The app filters drivers based on experience, vehicle type, and user ratings.
- Real-Time Tracking: Users can track the driver’s current location during the ride.
- Flexibility: Rides can be canceled by either party, with users eligible for refunds.
Limitations
- Data Accuracy: Incorrect information can affect the service.
- Internet Dependency: An active internet connection is required.
- Unverified Data: The app does not verify the driver’s data, relying on user reviews for quality assurance.
Conclusion
The Android driver hiring app offers a convenient solution for those looking to hire drivers for their rides. Its user-friendly features, such as tailored driver profiles and real-time tracking, make it a go-to app for both drivers and users alike.
Sample Code
AndroidManifest.xml
<uses-permission android:name="android.permission.INTERNET" />
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
MainActivity.java
import android.os.Bundle;
import com.google.firebase.database.DatabaseReference;
import com.google.firebase.database.FirebaseDatabase;
public class MainActivity extends AppCompatActivity {
private DatabaseReference mDatabase;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mDatabase = FirebaseDatabase.getInstance().getReference();
// For demonstration, let's add a user and a driver
addUser("John", "[email protected]");
addDriver("Mike", "[email protected]", "5 years");
}
private void addUser(String name, String email) {
String userId = mDatabase.push().getKey();
User user = new User(name, email);
mDatabase.child("users").child(userId).setValue(user);
}
private void addDriver(String name, String email, String experience) {
String driverId = mDatabase.push().getKey();
Driver driver = new Driver(name, email, experience);
mDatabase.child("drivers").child(driverId).setValue(driver);
}
}
User.java
public class User {
public String name;
public String email;
public User(String name, String email) {
this.name = name;
this.email = email;
}
}
Driver.java
public class Driver {
public String name;
public String email;
public String experience;
public Driver(String name, String email, String experience) {
this.name = name;
this.email = email;
this.experience = experience;
}
}
Firebase Realtime Database Rules
{
"rules": {
"users": {
".read": "auth != null",
".write": "auth != null"
},
"drivers": {
".read": "auth != null",
".write": "auth != null"
}
}
}
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Online Driver Hiring Through Android App PDF
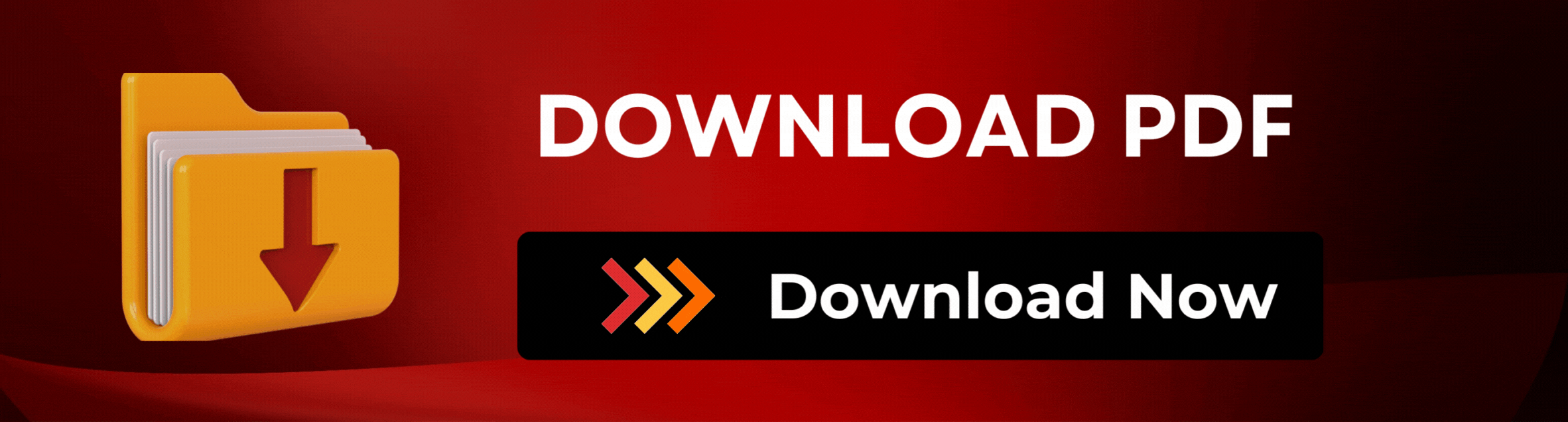