
Society Management System Project
Introduction
Managing a housing society is no small feat. With various responsibilities like maintenance billing, fund management, and resident data management, the task can become overwhelming. Enter our Society Management System Project—an all-in-one solution designed to simplify society management.

Features
Automated Billing
Our society management app offers a robust automated billing feature. Say goodbye to manual calculations for maintenance, parking, cultural funds, and emergency funds. Our system will automatically calculate these and include them in individual flat bills, saving you time and effort.
Comprehensive Data Management
The system allows administrators to input flat owner data, including pictures and contact information. This enables easier communication and better management. Need to send out a notice or update residents about an upcoming event? It’s all possible with just a few clicks.
Fund Management
Managing society funds can be tricky, but not with our society maintenance app. Allocate, track, and manage cultural funds, emergency funds, and general society maintenance with efficiency and transparency.
Advantages
- Ease of use for society secretaries in handling flat owner data.
- Efficient management of society funds.
- Greater transparency and trust among residents.
Disadvantages
- Currently optimized for single societies.
- Does not support bank payment status, dd, or cheque status. We are working on integrating these features in future updates.
Conclusion
Our society management system project is designed to bring simplicity and efficiency to the complicated task of housing society management. With features like automated billing and comprehensive data management, we aim to revolutionize how housing societies function.
Sample Code
class SocietyManagement:
def __init__(self):
self.flat_owners = {} # Stores flat owner data
self.bills = {} # Stores bills
# Function to add flat owner
def add_flat_owner(self, flat_no, name, contact_info):
self.flat_owners[flat_no] = {
'name': name,
'contact_info': contact_info,
'maintenance': 0,
'parking': 0,
'cultural_fund': 0,
'emergency_fund': 0
}
# Function to generate bill for a flat
def generate_bill(self, flat_no, maintenance, parking, cultural_fund, emergency_fund):
if flat_no in self.flat_owners:
self.flat_owners[flat_no]['maintenance'] = maintenance
self.flat_owners[flat_no]['parking'] = parking
self.flat_owners[flat_no]['cultural_fund'] = cultural_fund
self.flat_owners[flat_no]['emergency_fund'] = emergency_fund
total_bill = maintenance + parking + cultural_fund + emergency_fund
self.bills[flat_no] = total_bill
return f"Bill generated for flat {flat_no}: {total_bill}"
else:
return "Flat owner not found"
# Function to view all bills
def view_all_bills(self):
return self.bills
if __name__ == "__main__":
society = SocietyManagement()
# Adding flat owners
society.add_flat_owner("101", "John", "[email protected]")
society.add_flat_owner("102", "Emily", "[email protected]")
# Generating bills
print(society.generate_bill("101", 2000, 300, 100, 50)) # Output: Bill generated for flat 101: 2450
print(society.generate_bill("102", 2100, 300, 110, 60)) # Output: Bill generated for flat 102: 2570
# Viewing all bills
print(society.view_all_bills()) # Output: {'101': 2450, '102': 2570}
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Society Management System Project PDF
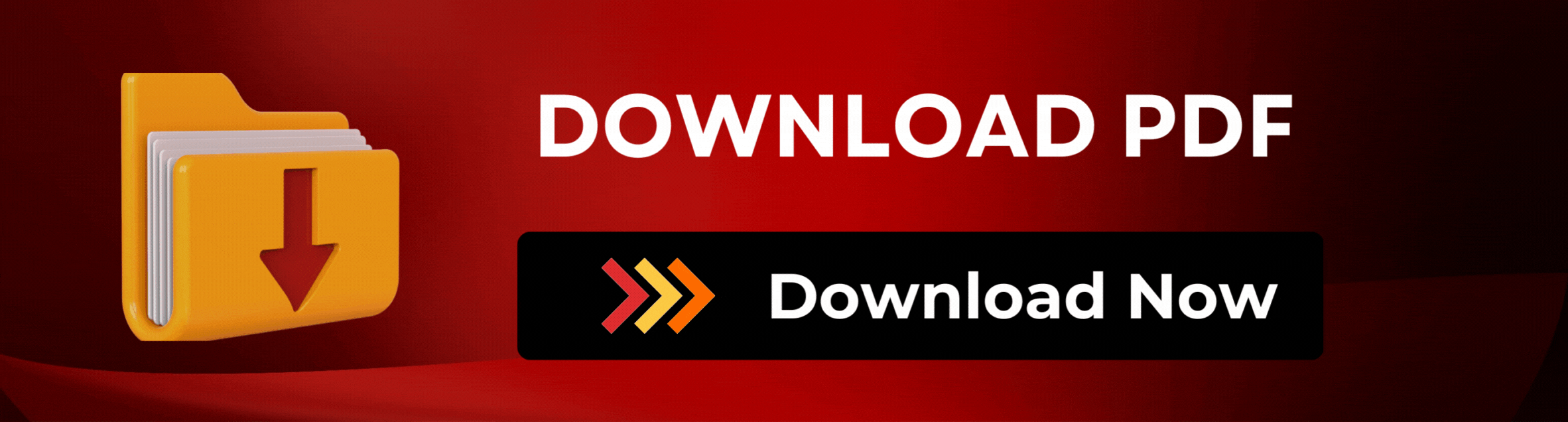