
The Online Examination Management System
In the epoch of digital transformation, educational institutions are incessantly seeking adept systems to refine the delivery and assessment of academic courses. The Online Examination Management System (OEMS) emerges as a linchpin in this regard, adeptly amalgamating course outline dissemination and examination orchestration under a unified digital canopy.
The cornerstone of this system is a meticulously crafted syllabus, a quintessential instructional blueprint that delineates the academic voyage awaiting the students. It meticulously lists the topics and concepts, forming the bedrock upon which the final examinations are structured. This digital syllabus not only elucidates the course objectives but meticulously outlines the structural design encompassing assignments, examinations, review sessions, and a plethora of other scholastic activities essential for an enriching learning experience.

The OEMS is structured into two principal entities – the Admin and the User. The Admin, armed with requisite credentials, navigates the helm, orchestrating the course structure by selecting the pertinent stream and subject. They are entrusted with the management of chapters and sub-chapters, facilitating additions and pruning redundancies. Their realm extends to crafting Multiple Choice Questions (MCQs) based on the chapters selected by users, alongside generating practice questions for individual chapters to foster a comprehensive understanding.
On the flip side, users, post registration and course selection, dive into a realm of academic exploration. They can effortlessly select subjects, delve into chapters, and engage in MCQ tests to gauge their grasp on the syllabus. Additionally, they can access questions tailored for each chapter, aiding in a thorough revision and preparation for the impending examinations.
Advantages
- The OEMS is a beacon of transparency, illuminating the course structure, objectives, and learning outcomes for students, paving the path towards their academic success.
- It meticulously outlines the responsibilities and actions requisite for a student’s success, fostering a culture of accountability and self-driven learning.
Disadvantages
- A relentless reliance on an active internet connection is a requisite for accessing the system, which could be a hindrance in areas with erratic internet connectivity.
- The system’s efficiency is contingent on the accuracy of the data input by the user, mandating a level of diligence to ensure the system functions optimally.
Sample Code
pip install Flask Flask-SQLAlchemy
Project Structure
/online-exam-system
/templates
index.html
admin.html
user.html
/static
/css
styles.css
app.py
models.py
models.py
from flask_sqlalchemy import SQLAlchemy
db = SQLAlchemy()
class Course(db.Model):
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(100))
questions = db.relationship('Question', backref='course', lazy=True)
class Question(db.Model):
id = db.Column(db.Integer, primary_key=True)
text = db.Column(db.String(200))
options = db.Column(db.String(200)) # JSON string of options
answer = db.Column(db.String(50))
course_id = db.Column(db.Integer, db.ForeignKey('course.id'), nullable=False)
app.py
from flask import Flask, render_template, request, redirect, url_for
from models import db, Course, Question
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///exam.db'
db.init_app(app)
@app.route('/')
def index():
return render_template('index.html')
@app.route('/admin', methods=['GET', 'POST'])
def admin():
if request.method == 'POST':
course_name = request.form['course_name']
new_course = Course(name=course_name)
db.session.add(new_course)
db.session.commit()
return redirect(url_for('admin'))
courses = Course.query.all()
return render_template('admin.html', courses=courses)
@app.route('/user')
def user():
courses = Course.query.all()
return render_template('user.html', courses=courses)
if __name__ == '__main__':
with app.app_context():
db.create_all() # Create tables on startup
app.run(debug=True)
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download The Online Examination Management System PDF
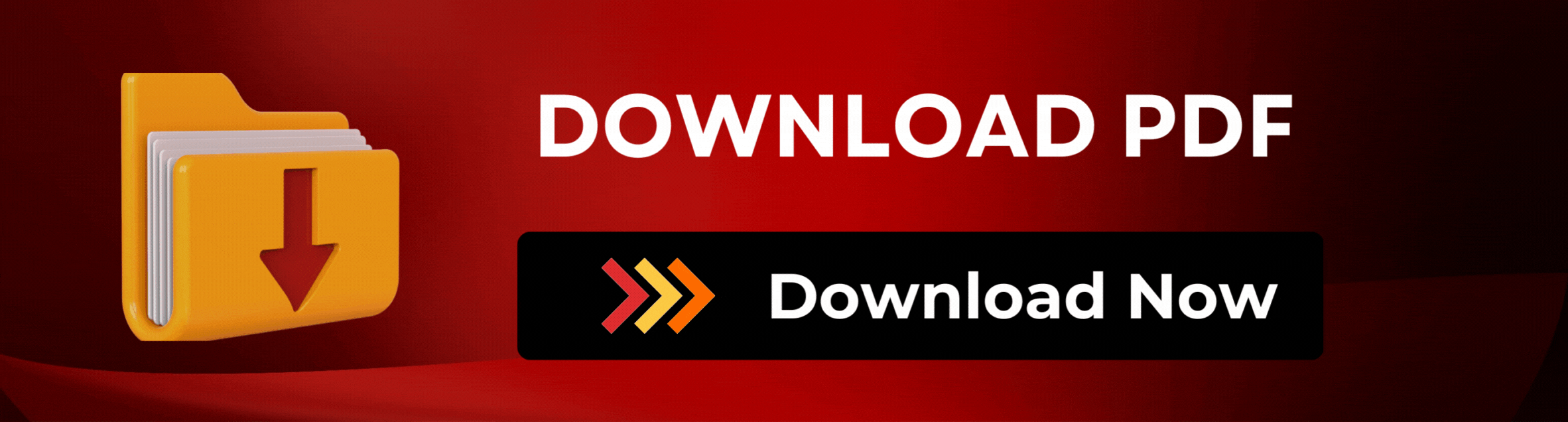