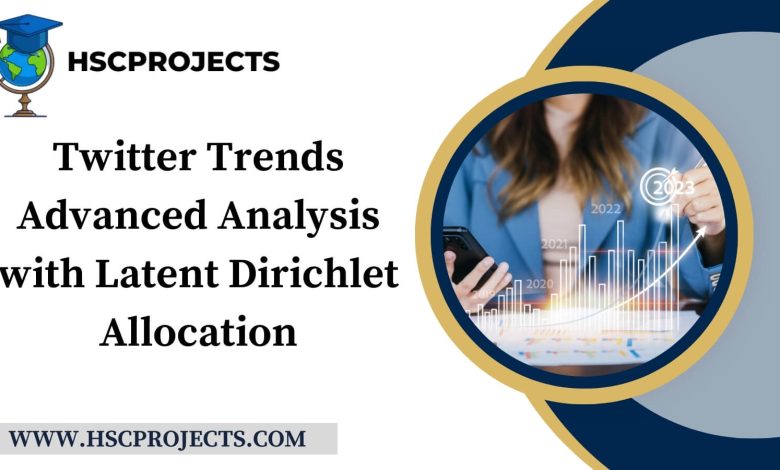
Twitter Trends Advanced Analysis with Latent Dirichlet Allocation
Introduction to Twitter Trend Analysis
Twitter, a bustling microblogging platform, serves as a barometer for public opinion and interests. Trends on Twitter encapsulate the voices of millions, reflecting topics that capture collective attention at any given moment. These trends can be sparked by various triggers, including news, ongoing events, memes, and commemorative occasions.
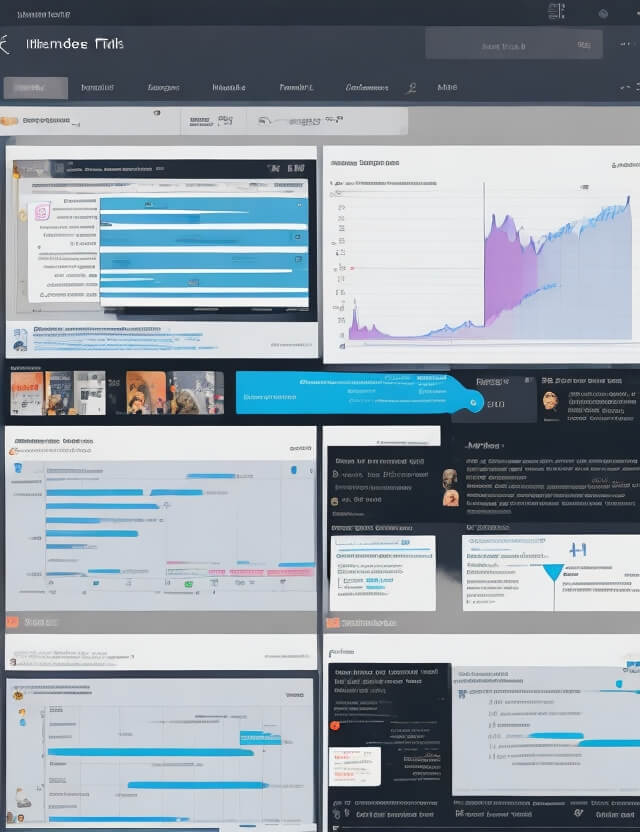
How Latent Dirichlet Allocation Enhances Twitter Trend Analysis
Latent Dirichlet Allocation (LDA) is a sophisticated machine learning technique used for topic modeling. In the context of Twitter, LDA helps to categorize tweets into coherent topics, providing a deeper understanding of what drives a trend. This approach is particularly effective in unraveling complex, multi-faceted discussions that are common on Twitter.
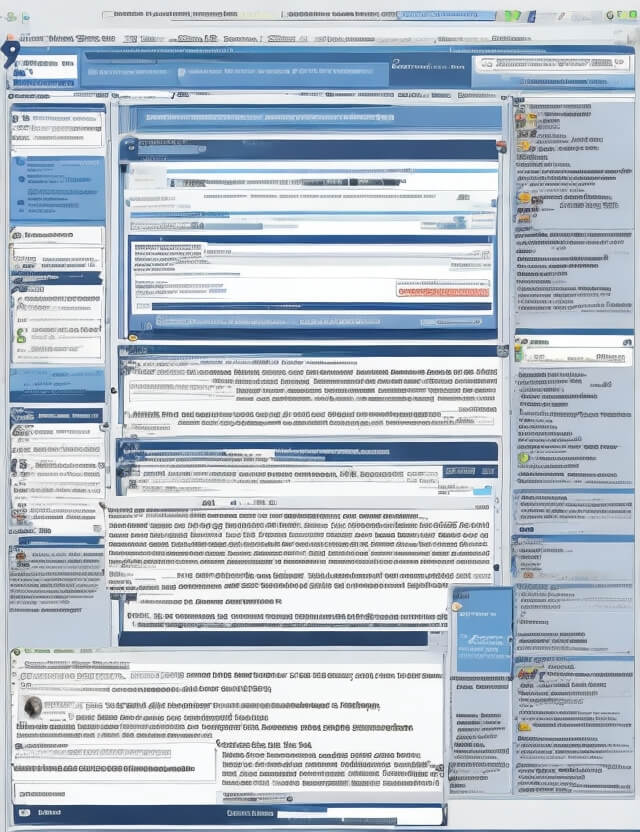
User Interaction with the Trend Analysis System
- Keyword Search: Users can input keywords to search for relevant trends. This feature allows for targeted exploration of specific topics.
- Trending Tweet Display: Based on the keyword, the system fetches and displays trending tweets, prioritizing those with hashtags.
- Detailed Tweet Insights: By selecting a trend, users can view detailed conversations, offering a window into the public discourse surrounding a topic.
Advantages of the System
- Real-Time Trend Insights: Stay updated with the most talked-about topics on Twitter.
- Targeted Search Capability: Tailor your trend exploration with keyword-based searches.
- Accurate Trend Analysis: Leverage LDA for precise categorization of tweet topics.
Potential Limitations
- Dependency on Accurate Keyword Input: The system’s effectiveness hinges on correctly entered keywords.
This Twitter trend analysis tool stands out as a valuable asset for marketers, researchers, and the general public, offering a real-time pulse of the online world. With the power of Latent Dirichlet Allocation, it transforms vast tweet volumes into meaningful insights.
Sample Code
Fetch Tweets using Tweepy
pip install tweepy
import tweepy
# Twitter API credentials
consumer_key = 'YOUR_CONSUMER_KEY'
consumer_secret = 'YOUR_CONSUMER_SECRET'
access_token = 'YOUR_ACCESS_TOKEN'
access_token_secret = 'YOUR_ACCESS_TOKEN_SECRET'
# Authenticate to Twitter
auth = tweepy.OAuthHandler(consumer_key, consumer_secret)
auth.set_access_token(access_token, access_token_secret)
api = tweepy.API(auth)
# Function to fetch tweets
def fetch_tweets(keyword, max_tweets):
for tweet in tweepy.Cursor(api.search, q=keyword, lang="en", tweet_mode='extended').items(max_tweets):
yield tweet.full_text
# Example usage
for tweet in fetch_tweets("example keyword", 10):
print(tweet)
Preprocess Tweets
pip install nltk
import re
import nltk
from nltk.corpus import stopwords
from nltk.stem.wordnet import WordNetLemmatizer
nltk.download('stopwords')
nltk.download('wordnet')
def preprocess_tweet(text):
# Lowercasing
text = text.lower()
# Remove URLs, mentions, hashtags
text = re.sub(r'http\S+|www\S+|https\S+|@\S+|#\S+', '', text)
# Remove punctuations
text = re.sub(r'[^a-z0-1\s]', '', text)
# Tokenization
tokens = text.split()
# Remove stopwords
stop_words = set(stopwords.words('english'))
tokens = [token for token in tokens if token not in stop_words]
# Lemmatization
lemmatizer = WordNetLemmatizer()
tokens = [lemmatizer.lemmatize(token) for token in tokens]
return tokens
# Example usage
processed_tweets = [preprocess_tweet(tweet) for tweet in fetch_tweets("example keyword", 10)]
Apply LDA using Gensim
pip install gensim
from gensim import corpora, models
# Creating the term dictionary
dictionary = corpora.Dictionary(processed_tweets)
# Converting list of documents (corpus) into Document Term Matrix
doc_term_matrix = [dictionary.doc2bow(tweet) for tweet in processed_tweets]
# Creating the LDA model
lda = models.LdaModel(doc_term_matrix, num_topics=3, id2word = dictionary, passes=50)
# Print the topics
for idx, topic in lda.print_topics(-1):
print(f"Topic: {idx} \nWords: {topic}")
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Twitter Trends Advanced Analysis with Latent Dirichlet Allocation PDF
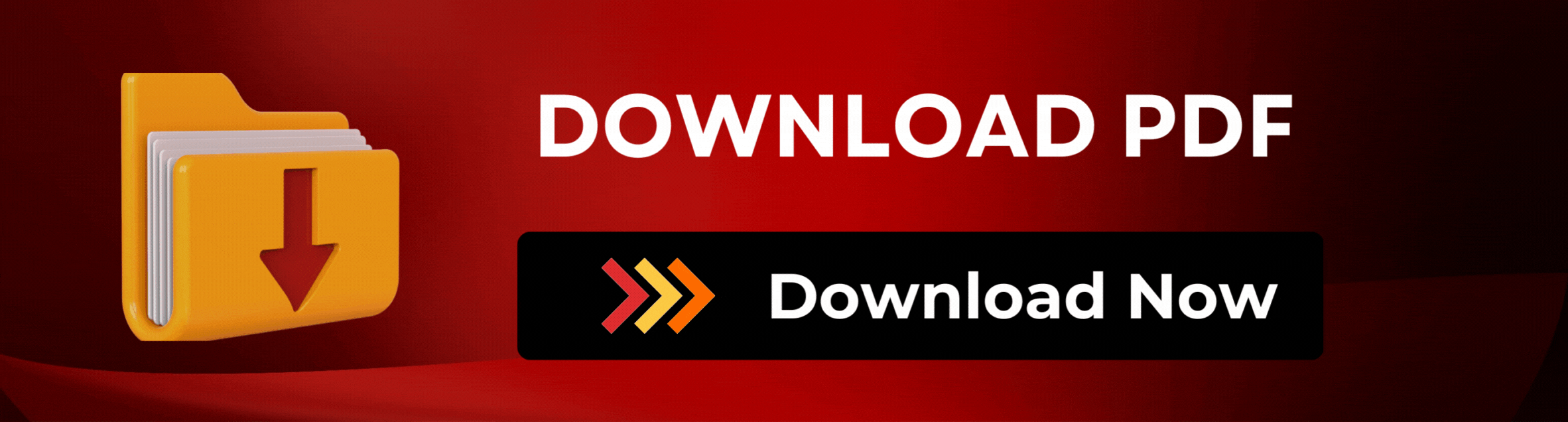