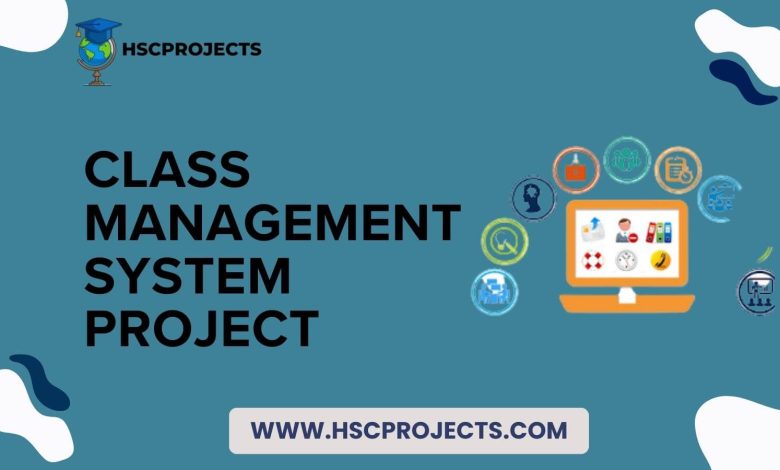
Class Management System Project
Introduction
The educational landscape is evolving, and so are the needs for efficient systems to manage classes, courses, and students. Our Course Management System Project is designed to meet these challenges head-on, offering a streamlined approach to managing educational institutions.
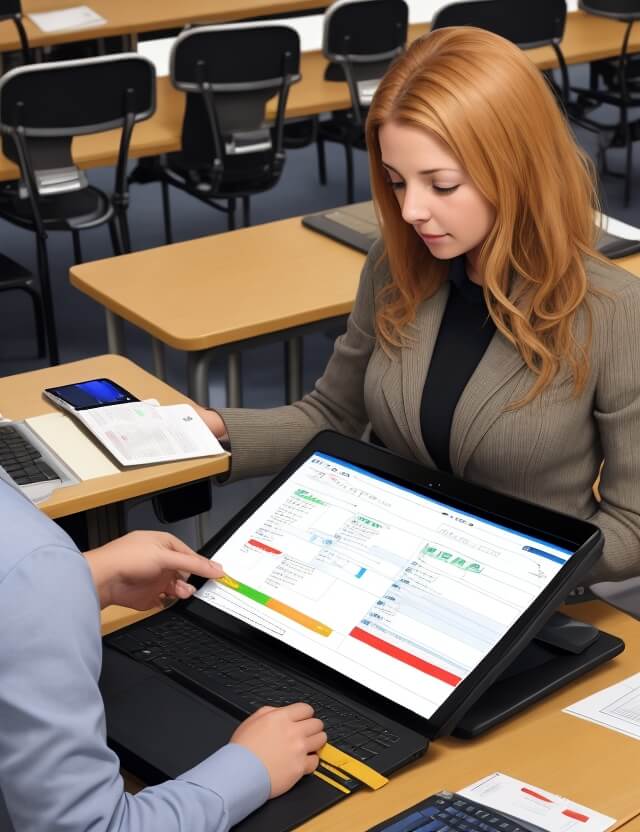
Features
Student Management System Abstract
Our system provides a robust student management interface, allowing administrators to track student progress, attendance, and performance. The system can also generate reports, making it easier for educators to make data-driven decisions.
Faculty Accounts
The Course Management System includes a dedicated portal for faculty members. This portal not only allows faculty to update course materials but also keeps track of their payment status and contract renewals.
Batch Scheduling
The system excels in creating and managing batches of students based on their courses and exam priorities. This feature ensures that each student receives the attention they deserve, thereby improving the overall quality of education.
Notifications
Administrators are notified of various important events such as faculty contract renewals, fee due dates, and other essential milestones. This feature ensures that nothing slips through the cracks.
Advantages
- Automated Operations: The system automates many of the tasks that were previously done manually, such as maintaining class information and calculating faculty and student fees.
- Efficient Record-Keeping: All employee records, including salary information, are systematically stored, ensuring a smooth payment process.
- Time and Cost-Effective: Automation saves both time and effort, making the system cost-effective in the long run.
Disadvantages
- Database Requirements: The system requires a large database to function effectively.
- Manual Updates: While the system is automated, administrators still need to manually update faculty and staff information.
Conclusion
The Course Management System Project is a comprehensive solution for educational institutions looking to streamline their operations. From student management to faculty accounts, the system offers a range of features designed to improve efficiency and effectiveness.
Sample Code
First, install the required packages if you haven’t already:
pip install Flask
pip install Flask-SQLAlchemy
from flask import Flask, request, jsonify
from flask_sqlalchemy import SQLAlchemy
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///class_management.db'
db = SQLAlchemy(app)
class Student(db.Model):
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(50), nullable=False)
class Faculty(db.Model):
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(50), nullable=False)
subject = db.Column(db.String(50), nullable=False)
@app.route('/add_student', methods=['POST'])
def add_student():
data = request.get_json()
new_student = Student(name=data['name'])
db.session.add(new_student)
db.session.commit()
return jsonify({"message": "Student added"}), 201
@app.route('/add_faculty', methods=['POST'])
def add_faculty():
data = request.get_json()
new_faculty = Faculty(name=data['name'], subject=data['subject'])
db.session.add(new_faculty)
db.session.commit()
return jsonify({"message": "Faculty added"}), 201
@app.route('/view_students', methods=['GET'])
def view_students():
student_list = Student.query.all()
students = [{"id": x.id, "name": x.name} for x in student_list]
return jsonify(students)
@app.route('/view_faculty', methods=['GET'])
def view_faculty():
faculty_list = Faculty.query.all()
faculties = [{"id": x.id, "name": x.name, "subject": x.subject} for x in faculty_list]
return jsonify(faculties)
if __name__ == '__main__':
db.create_all()
app.run(debug=True)
To run the code:
- Save the code in a file, ‘say
app.py
‘. - Open a terminal and navigate to the folder where ‘
app.py
‘ is saved. - Run’
python app.py
‘
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Class Management System Project PDF
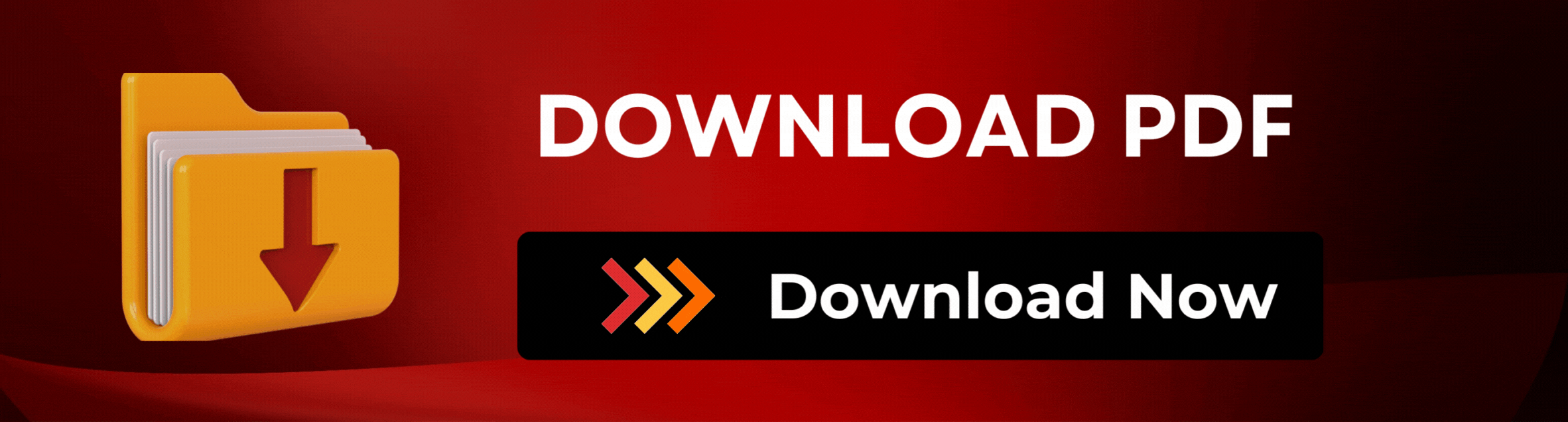