
Voice Logger Software System
Introduction
In an era where data is king, having an effective voice logger software system is crucial. Whether it’s for customer service, quality control, or compliance, a voice logger is indispensable for storing and managing voice calls along with related data.

Key Features
The primary function of a voice logger system is the recording and storage of voice calls. Not only does it capture the audio but it also collates additional details like the date/time of the call, caller/receiver numbers, and the call duration. All this data is securely stored in a database for easy retrieval.
How It Works
Upon initiating a call, the system begins the recording process and tracks various call parameters. It computes the call duration based on the start and end times, and archives it along with other call details in a database. Administrators can query this database based on specific times or dates to retrieve relevant call records.
Data Retrieval
An administrator can search through the database for specific call records based on different criteria such as date or time of the call. The system then generates a list of all matching calls, complete with their corresponding data and recorded audio.
Use Cases
- Call Centers: For quality assurance and training
- Financial Institutions: For compliance with regulatory standards
- Emergency Services: For reviewing crisis calls
Security and Compliance
Security is a major concern. The system ensures that all voice recordings and associated data are encrypted and stored securely, accessible only to authorized personnel.
Conclusion
A voice logger system is an invaluable tool for any organization that needs to keep track of call interactions for compliance, quality assurance, or data analysis. Its ability to record, store, and retrieve voice and data efficiently makes it a key asset in modern communication infrastructures.
Sample Code
pip install sounddevice numpy
import sounddevice as sd
import numpy as np
import sqlite3
import datetime
# Initialize SQLite database
conn = sqlite3.connect('voice_logger.db')
c = conn.cursor()
# Create table if it doesn't exist
c.execute('''CREATE TABLE IF NOT EXISTS calls
(date text, start_time text, end_time text, duration integer, audio BLOB)''')
# Commit the changes
conn.commit()
def record_call(duration, samplerate=44100):
audio_data = sd.rec(int(samplerate * duration), samplerate=samplerate, channels=2, dtype='int16')
sd.wait()
return audio_data.tobytes()
def log_call(audio_data):
date = datetime.datetime.now().strftime("%Y-%m-%d")
start_time = datetime.datetime.now().strftime("%H:%M:%S")
end_time = (datetime.datetime.now() + datetime.timedelta(seconds=5)).strftime("%H:%M:%S")
duration = 5 # in seconds
c.execute("INSERT INTO calls (date, start_time, end_time, duration, audio) VALUES (?, ?, ?, ?, ?)",
(date, start_time, end_time, duration, audio_data))
conn.commit()
def main():
print("Recording call for 5 seconds...")
audio_data = record_call(5)
print("Logging call to database...")
log_call(audio_data)
print("Call logged successfully.")
if __name__ == '__main__':
main()
# Close the database connection
conn.close()
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Voice Logger Software System PDF
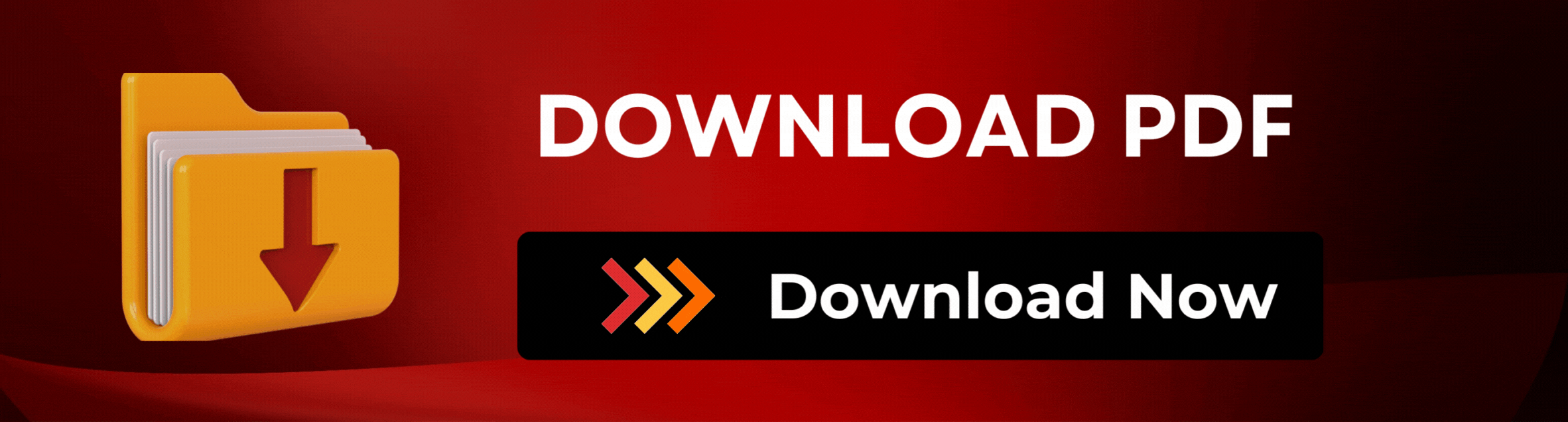