
Website For Real-Time Cricket Scores
Introduction
In the age of digitization, keeping track of live cricket scores has never been easier. Whether you’re stuck in traffic, working late, or don’t have access to television, our live cricket score website ensures that you never miss a ball.
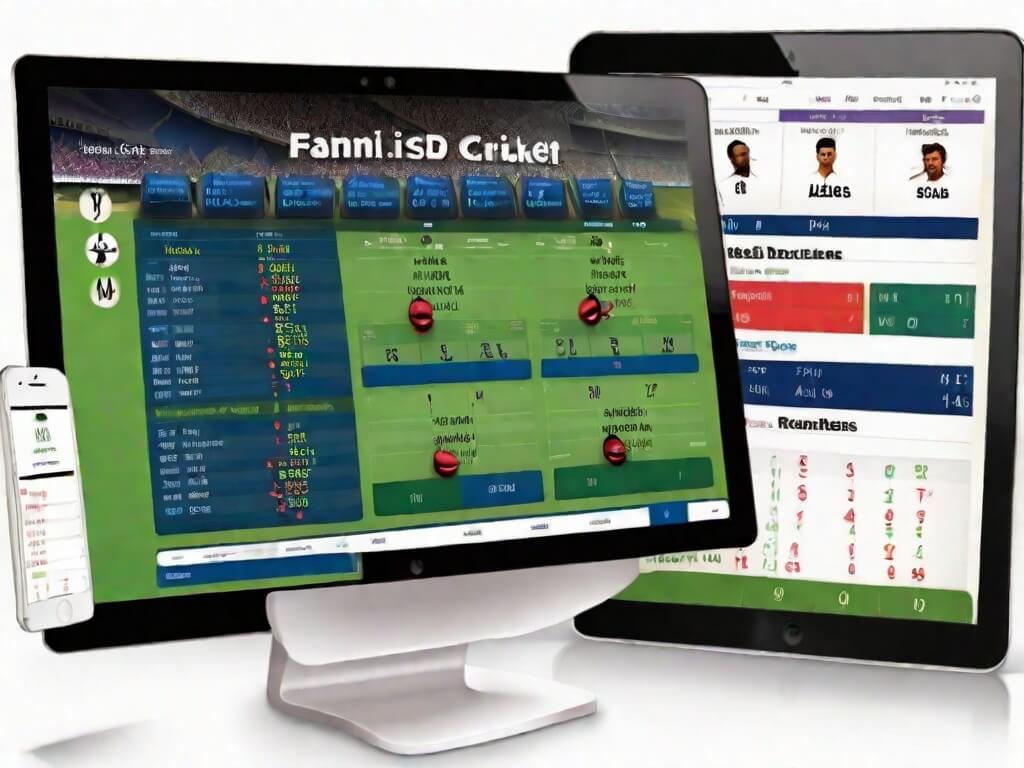
Features and Usability
Our web-based cricket scoring software doesn’t just update you on the current score; it offers a plethora of features:
- Real-time Score Updates: Scores are updated in real-time by our admins.
- Match Timetable: Know in advance about upcoming matches and plan your schedule accordingly.
- Multi-Platform Access: Available as a web cricket app, the project ensures that you can check scores from any internet-enabled device.
Technology Stack
Built with the latest in web technologies, this project uses C# for its front-end and SQL for its back-end. This robust framework ensures reliability and smooth user experience.
Advantages
- Accessibility: Check live cricket scores from anywhere.
- Stay Updated: Never miss out on any match.
- Cost-Effectiveness: Our project is budget-friendly.
Limitations
- Internet Connection Required: To access our live cricket score result, an active internet connection is mandatory.
- No Live Match Streaming: While we offer live score updates, the actual match cannot be viewed on our platform.
Conclusion
Our cricket scorecard maker and design features offer a comprehensive and engaging platform for cricket enthusiasts. Tailored to meet the demands of modern cricket followers, this website provides an immersive experience unlike any other cricket scoreboard online.
Sample Code
Install-Package Microsoft.EntityFrameworkCore.SqlServer -Version 5.0.0
Create a Model for CricketMatch:
public class CricketMatch
{
public int Id { get; set; }
public string Team1 { get; set; }
public string Team2 { get; set; }
public int Team1Score { get; set; }
public int Team2Score { get; set; }
public DateTime MatchDate { get; set; }
}
Set up Database Context:
using Microsoft.EntityFrameworkCore;
public class CricketContext : DbContext
{
public DbSet<CricketMatch> CricketMatches { get; set; }
protected override void OnConfiguring(DbContextOptionsBuilder options)
=> options.UseSqlServer(@"Server=localhost;Database=CricketDB;Trusted_Connection=True;");
}
Create Controller for API Endpoints:
using Microsoft.AspNetCore.Mvc;
using Microsoft.EntityFrameworkCore;
using System.Collections.Generic;
using System.Threading.Tasks;
[ApiController]
[Route("[controller]")]
public class CricketController : ControllerBase
{
private readonly CricketContext _context;
public CricketController(CricketContext context)
{
_context = context;
}
[HttpGet]
public async Task<IEnumerable<CricketMatch>> GetMatchesAsync()
{
return await _context.CricketMatches.ToListAsync();
}
[HttpPost]
public async Task UpdateMatchAsync(CricketMatch updatedMatch)
{
_context.Entry(updatedMatch).State = EntityState.Modified;
await _context.SaveChangesAsync();
}
}
Front-end: HTML and JavaScript
<!DOCTYPE html>
<html>
<head>
<title>Live Cricket Score</title>
<script>
async function fetchScores() {
const res = await fetch('/Cricket');
const data = await res.json();
let output = "<h1>Upcoming and Live Matches</h1>";
data.forEach(match => {
output += `<p>${match.Team1} vs ${match.Team2} - ${match.Team1Score}-${match.Team2Score} on ${new Date(match.MatchDate).toDateString()}</p>`;
});
document.getElementById("matchData").innerHTML = output;
}
fetchScores();
setInterval(fetchScores, 10000); // Update every 10 seconds
</script>
</head>
<body>
<div id="matchData"></div>
</body>
</html>
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Website For Real-Time Cricket Scores PDF
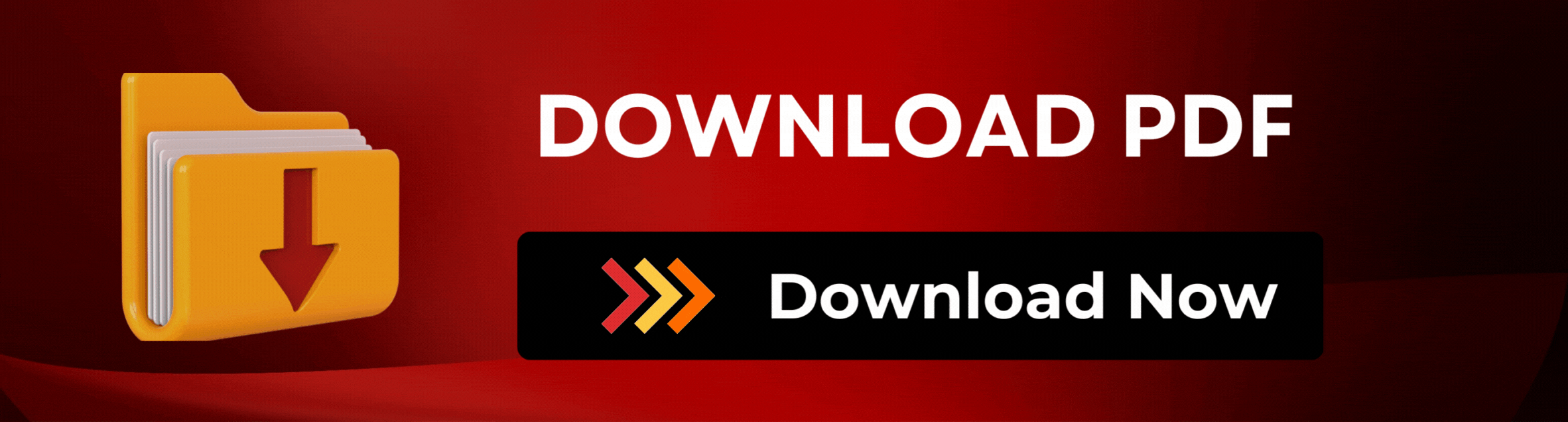