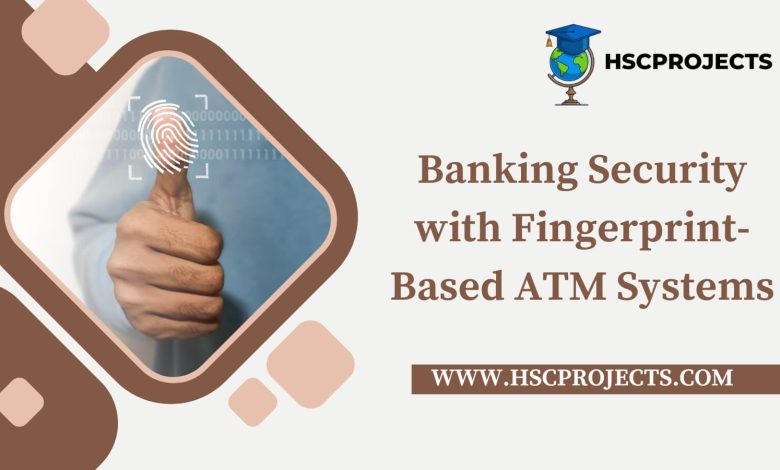
Banking Security with Fingerprint-Based ATM Systems
Introduction
The world is rapidly moving towards digitalization, and with this change comes the need for heightened security, especially in the banking sector. The fingerprint-based ATM system is a revolutionary concept that utilizes biometric technology to ensure a more secure and efficient banking experience. Gone are the days of worrying about lost ATM cards; all you need now is your fingerprint.
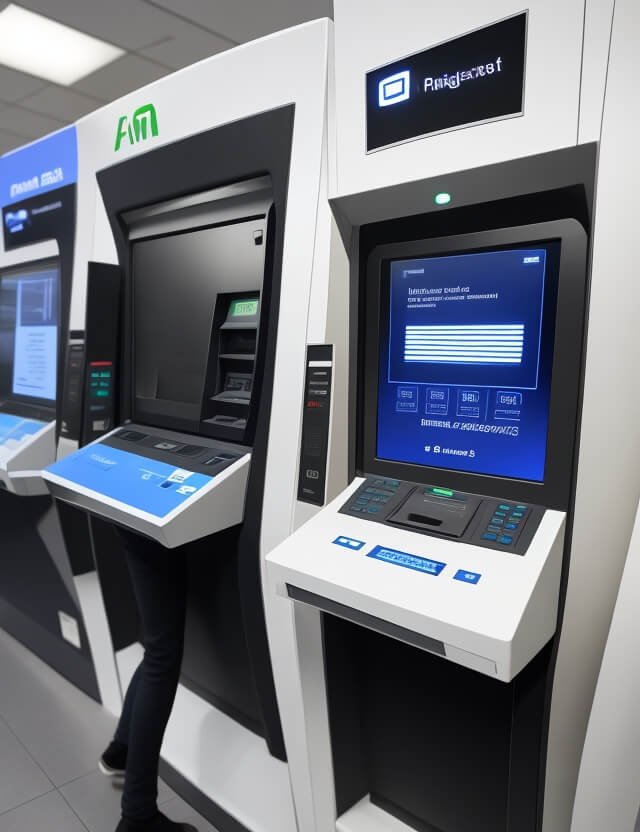
How It Works
With a fingerprint ATM, the unique minutiae features of a human fingerprint are utilized for authentication. Unlike traditional ATM cards, fingerprints can’t be lost, stolen, or easily replicated. Upon placing your finger on the scanner, the system matches the scanned fingerprint with the pre-registered fingerprint data. Once verified, the user can proceed to input a PIN code for further authentication and carry out various transactions such as money withdrawal, funds transfer, and balance checking.
Features
- Secure Login: The system employs dual security checks: your fingerprint and a PIN code.
- Cash Withdrawal: Specify the amount and the account type (savings or current) from which you want to withdraw.
- Money Transfer: Easily transfer money by entering the recipient’s account number.
- Balance Inquiry: Instantly view your account balance with just a touch.
- Transaction History: Keep tabs on your spending with the option to view your last five transactions.
Advantages and Disadvantages
Advantages
- Enhanced Security: Your fingerprint is unique, making this system far more secure than traditional ATMs.
- Convenience: No more carrying around a physical ATM card, as your fingerprint becomes your access key.
Disadvantages
- Fingerprint Recognition: A cut or abrasion on your finger could cause the system to fail to recognize you, although this is a rare occurrence.
ATM Project in Technological Context
For developers, this project opens a new domain in ATM management system projects. It has applications in ATM security systems and can be implemented using various platforms such as ATM simulator Python, ATM project in Visual Studio, and even in a mini ATM machine with a fingerprint sensor.
Sample Code
# Sample Python Code for Fingerprint-Based ATM System
class ATMSimulator:
def __init__(self):
self.accounts = {
'123456': {'pin': '1111', 'balance': 1000, 'transactions': []},
'654321': {'pin': '2222', 'balance': 2000, 'transactions': []},
}
self.current_account = None
def login(self, account_number, pin):
if account_number in self.accounts and self.accounts[account_number]['pin'] == pin:
self.current_account = account_number
return True
return False
def logout(self):
self.current_account = None
def withdraw(self, amount):
if self.current_account:
if self.accounts[self.current_account]['balance'] >= amount:
self.accounts[self.current_account]['balance'] -= amount
self.accounts[self.current_account]['transactions'].append(f'Withdrawn: {amount}')
return True
else:
return False
else:
return False
def deposit(self, amount):
if self.current_account:
self.accounts[self.current_account]['balance'] += amount
self.accounts[self.current_account]['transactions'].append(f'Deposited: {amount}')
return True
return False
def view_balance(self):
if self.current_account:
return self.accounts[self.current_account]['balance']
else:
return None
def view_last_five_transactions(self):
if self.current_account:
return self.accounts[self.current_account]['transactions'][-5:]
else:
return None
# Main program
atm = ATMSimulator()
# Assuming fingerprint is authenticated, proceed to login
if atm.login('123456', '1111'):
print("Login successful.")
# Perform transactions
atm.withdraw(100)
atm.deposit(200)
# View balance
print("Current Balance:", atm.view_balance())
# View last 5 transactions
print("Last 5 Transactions:", atm.view_last_five_transactions())
atm.logout()
else:
print("Login failed.")
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Subscribed? Click on Confirm
Download Banking Security with Fingerprint-Based ATM Systems PDF
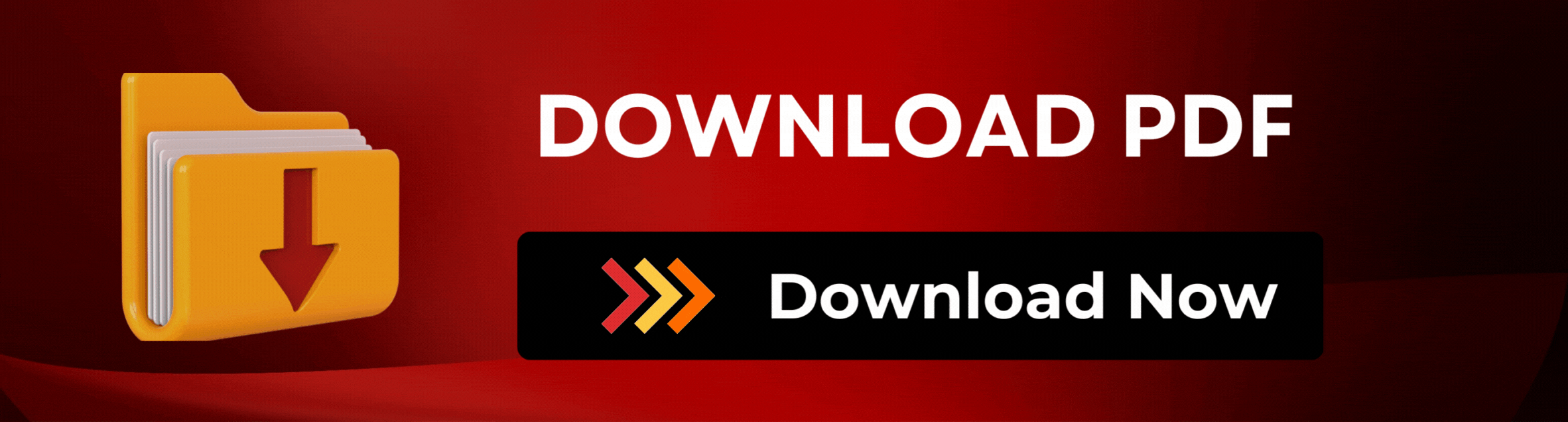