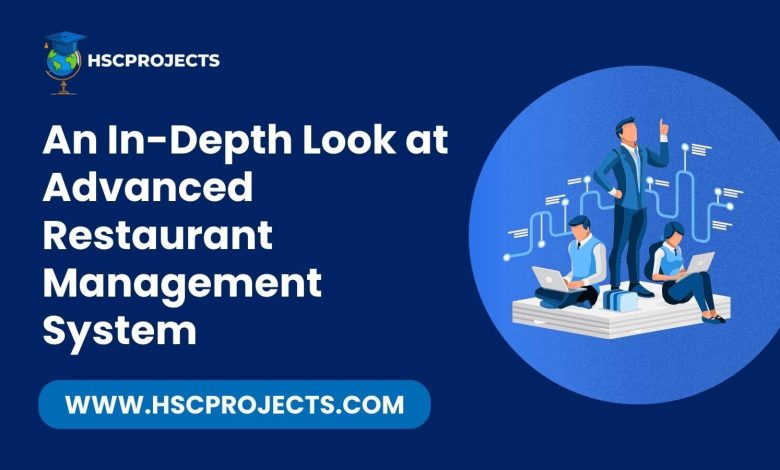
An In-Depth Look at Advanced Restaurant Management System
Introduction
The restaurant industry is booming, but with growth comes the need for more effective management. Advanced restaurant management systems are increasingly becoming the backbone of successful restaurants, offering a range of features that automate and streamline operations. This article will delve into the intricacies of these systems, from their advantages to how they can be implemented in various programming languages like Java and Python.
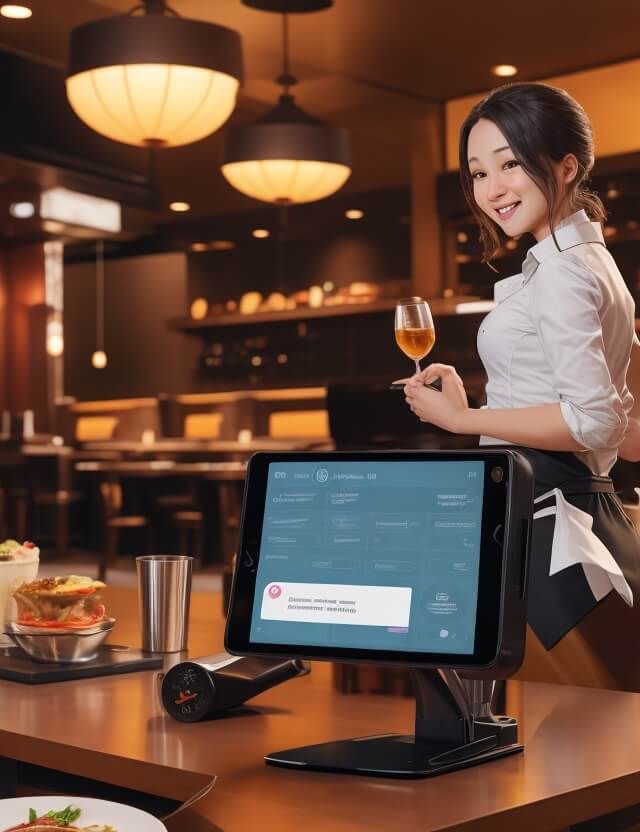
The Need for a Restaurant Management System
As restaurants expand, the complexity of their operations grows exponentially. Managing reservations, inventory, staff schedules, and customer service can become overwhelming. An advanced restaurant management system project aims to simplify these tasks, allowing restaurants to operate more efficiently and focus on what they do best: providing excellent food and service.
Key Features
- Table Booking: Allows customers to book tables in advance, especially useful during peak seasons.
- Inventory Management: Keeps track of stock levels, reducing waste and ensuring availability.
- Online Billing: Generates bills automatically, speeding up the payment process.
- Customer Information: Stores customer preferences and order history, enabling personalized service.
Advantages
- Efficiency: Automates various tasks, saving time and reducing errors.
- Cost-Effectiveness: Reduces the need for manual labor, cutting down on expenses.
- Customer Satisfaction: Enhances the dining experience through quick service and personalized interactions.
Disadvantages
- Limited Physical Inspection: Customers can’t see the table they’ve booked in person before arriving.
- Reduced Human Interaction: While efficiency is increased, some customers may miss the personal touch of human service.
Implementation
Depending on your needs and technical skills, a restaurant management system project can be developed in various programming languages:
- Java: Suitable for large-scale, complex systems.
- Python: Ideal for smaller restaurants looking for quick deployment.
Conclusion
An advanced restaurant management system is not just a trend but a necessity in today’s fast-paced restaurant industry. It offers a host of benefits that can significantly improve operational efficiency and customer satisfaction. Whether you’re a small café or a large fine-dining establishment, there’s a system out there that can meet your specific needs.
Sample Code
# Restaurant Management System in Python
class Restaurant:
def __init__(self):
self.tables = {1: 'Available', 2: 'Available', 3: 'Available'}
self.bill = 0
def show_tables(self):
print("Table Status")
for table, status in self.tables.items():
print(f"Table {table}: {status}")
def book_table(self, table_number):
if self.tables[table_number] == 'Available':
self.tables[table_number] = 'Booked'
print(f"Table {table_number} has been booked.")
else:
print(f"Table {table_number} is already booked.")
def release_table(self, table_number):
if self.tables[table_number] == 'Booked':
self.tables[table_number] = 'Available'
print(f"Table {table_number} is now available.")
else:
print(f"Table {table_number} is already available.")
def generate_bill(self, amount):
self.bill += amount
print(f"Your current bill is: ${self.bill}")
if __name__ == "__main__":
restaurant = Restaurant()
while True:
print("\n1. Show Tables")
print("2. Book Table")
print("3. Release Table")
print("4. Generate Bill")
print("5. Exit")
choice = int(input("Enter your choice: "))
if choice == 1:
restaurant.show_tables()
elif choice == 2:
table_number = int(input("Enter table number to book: "))
restaurant.book_table(table_number)
elif choice == 3:
table_number = int(input("Enter table number to release: "))
restaurant.release_table(table_number)
elif choice == 4:
amount = int(input("Enter amount to add to bill: "))
restaurant.generate_bill(amount)
elif choice == 5:
print("Exiting...")
break
else:
print("Invalid choice. Please try again.")
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download An In-Depth Look at Advanced Restaurant Management System PDF
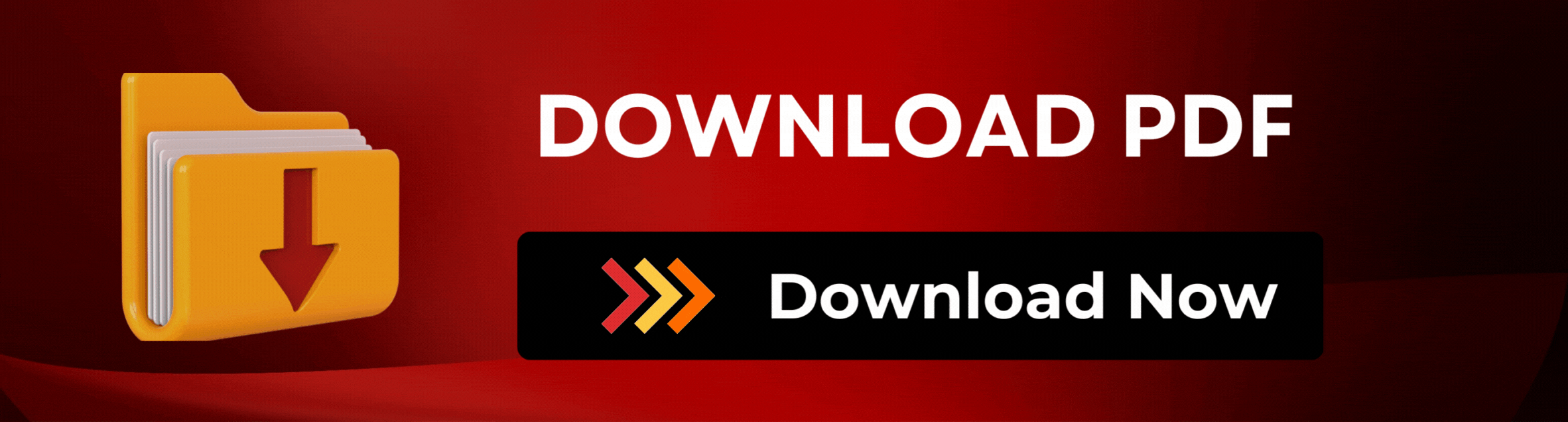