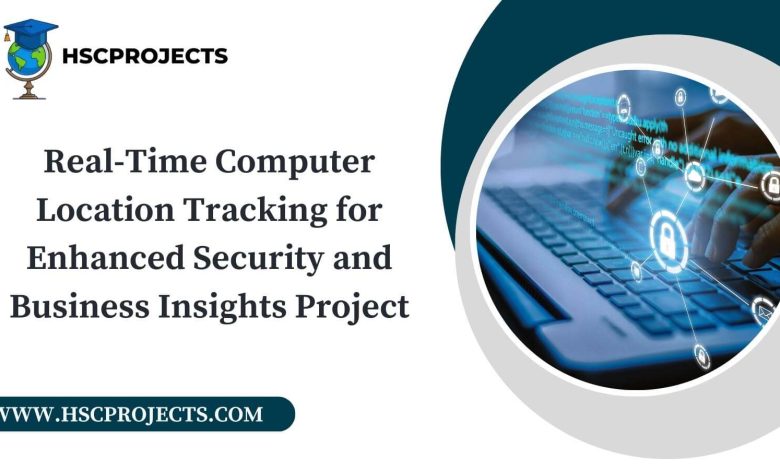
Real-Time Computer Location Tracking for Enhanced Security and Business Insights Project
Introduction
In today’s digital age, knowing the precise location of a computer can offer both significant security advantages and crucial business insights. Our Geo-Intelligent PC Tracker system utilizes advanced IP address tracking to provide a robust and efficient solution for tracking computer locations.
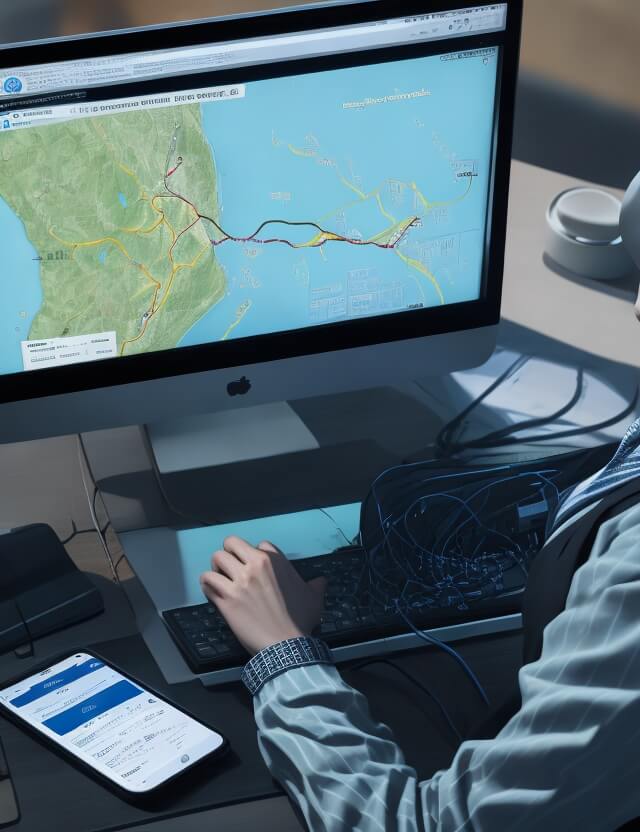
The Need for PC Tracking
Whether it’s for the purpose of cybersecurity or for identifying customer demographics, tracking a PC’s location has become imperative. Companies can analyze the geographic distribution of their customer base, thereby making informed marketing decisions.
How It Works
Our system captures live IP addresses using a Windows-based application and processes this data to map the associated geographical locations. Here’s the step-by-step breakdown:
- IP Capture: The system records the IP addresses of various computer terminals.
- Reverse Lookup: It then reverse-engineers these IP addresses to derive city coordinates.
- Mapping on Google Map: Finally, the coordinates are plotted on a Google map, visually representing the computer locations.
Technical Architecture
The entire system runs on the .NET framework, using an SQL backend to store and retrieve data. This ensures not only reliability but also the scalability of the solution.
Benefits
- Business Analytics: Helps in mapping the geographic distribution of customers.
- Security Measures: Assists in tracking lost or stolen devices and identifying suspicious activity.
- Real-Time Data: Provides instantaneous location tracking for rapid response scenarios.
Conclusion
Geo-Intelligent PC Tracker transcends traditional IP address tracking by converting raw IP data into actionable geographical information. This paves the way for advanced security protocols and more strategic business decision-making.
Sample Code
import requests
import json
def get_ip_address():
# Normally, you would obtain the IP address from the incoming request or your system's logs
return "8.8.8.8" # This is a sample public Google DNS IP address
def get_geolocation(ip_address):
api_url = f"http://ip-api.com/json/{ip_address}"
response = requests.get(api_url)
data = json.loads(response.text)
if data['status'] == 'fail':
return None
return {
'latitude': data['lat'],
'longitude': data['lon'],
'city': data['city']
}
def main():
ip_address = get_ip_address()
geolocation = get_geolocation(ip_address)
if geolocation:
print(f"IP Address: {ip_address}")
print(f"Latitude: {geolocation['latitude']}")
print(f"Longitude: {geolocation['longitude']}")
print(f"City: {geolocation['city']}")
# Here you can integrate the data with Google Maps API to plot the location
else:
print(f"Could not determine location for IP Address: {ip_address}")
if __name__ == "__main__":
main()
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Subscribed? Click on Confirm
Download Real-Time Computer Location Tracking for Enhanced Security and Business Insights Project PDF
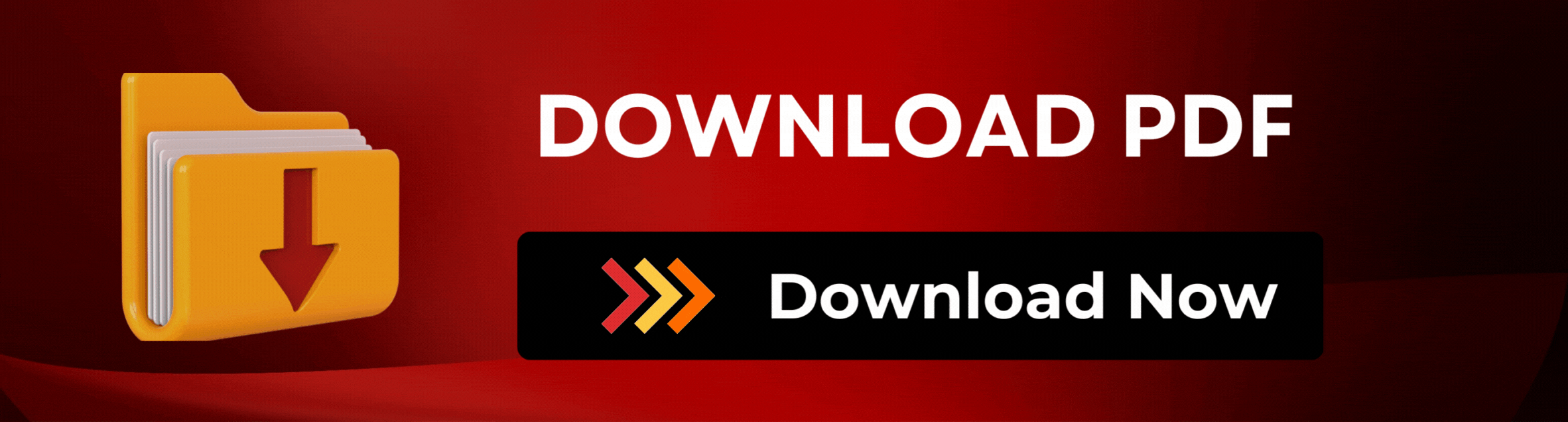