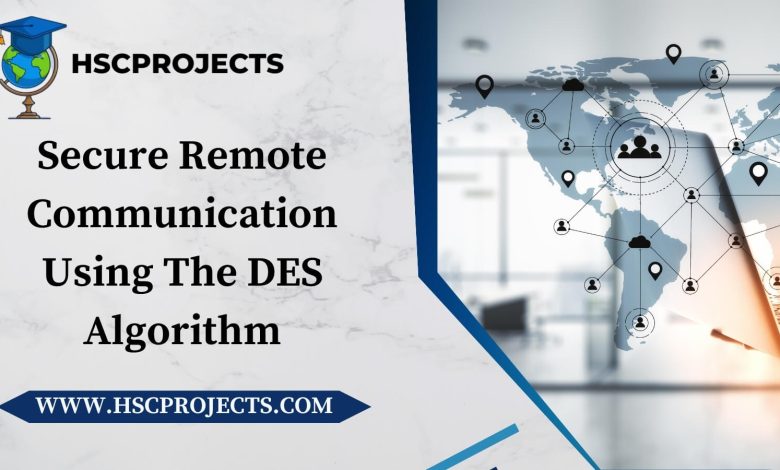
Secure Remote Communication Using The DES Algorithm
Introduction
The Data Encryption Standard (DES) algorithm stands as a cornerstone in the field of data encryption and security. Developed to protect sensitive information, DES employs a private key system, presenting a formidable challenge to unauthorized decryption efforts. This article delves into the implementation of DES for secure remote communication, highlighting its significance in an era where data breaches are increasingly common.
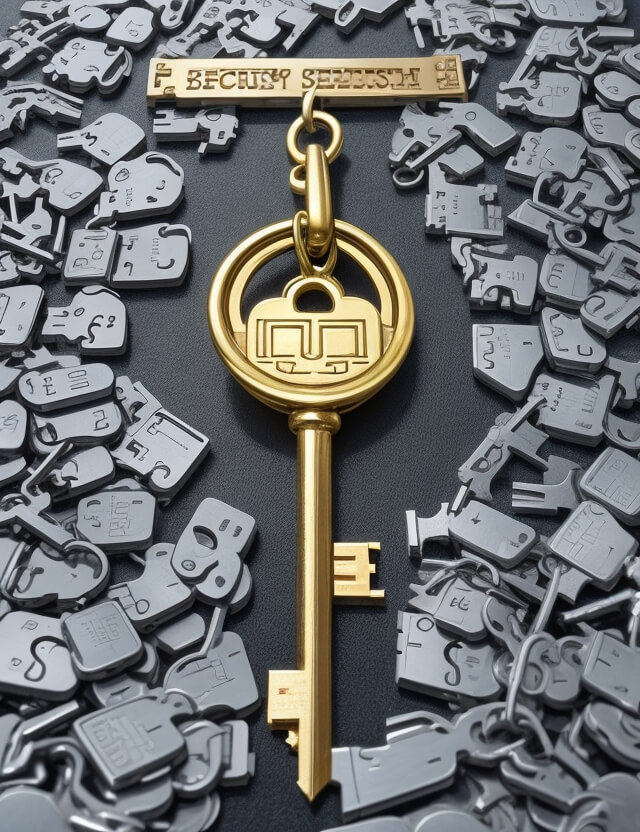
Understanding DES Algorithm
- Private Key Encryption: DES is a symmetric key algorithm, meaning the same private key is used for both encryption and decryption of data. The key is a closely guarded secret between the sender and receiver.
- Complexity and Security: With over 71 quadrillion possible encryption keys, the DES algorithm’s complexity ensures that each message is virtually impregnable to brute force attacks.
- Widespread Usage: Initially restricted due to its strength, DES now underpins secure data transfers in various sectors, including business, government, and military.
Implementation of DES in Remote Communication
- Secure Data Transfer: By encrypting data at the sending end and decrypting it at the receiving end with the DES algorithm, communication over unsecured networks is significantly safeguarded.
- Key Transfer: The private key, crucial for decryption, is transmitted securely, often as a one-time password (OTP) via email or another secure channel.
- Automated Process: The system automates the encryption, transmission, and decryption processes, streamlining secure communication between remote computers.
Advantages
- Robust Security: DES’s complexity and private key system make it an exceedingly secure communication method.
- Confidentiality: Ensures that the content of the communication remains inaccessible to unauthorized parties.
- Versatility: Applicable across various fields requiring secure data transfer.
Disadvantages
- Memory Requirement: Encrypted messages typically require more storage space compared to plain text.
- Speed: The encryption and decryption process may introduce delays compared to unsecured communications.
Conclusion
The DES Algorithm remains a fundamental tool in secure communication. Its implementation in remote data transfers provides an essential shield against data breaches and unauthorized access. As digital communication becomes increasingly integral to personal and professional life, the importance of such encryption standards cannot be overstated. With continuous advancements in cryptography, DES serves as a critical layer of defense in safeguarding digital information.
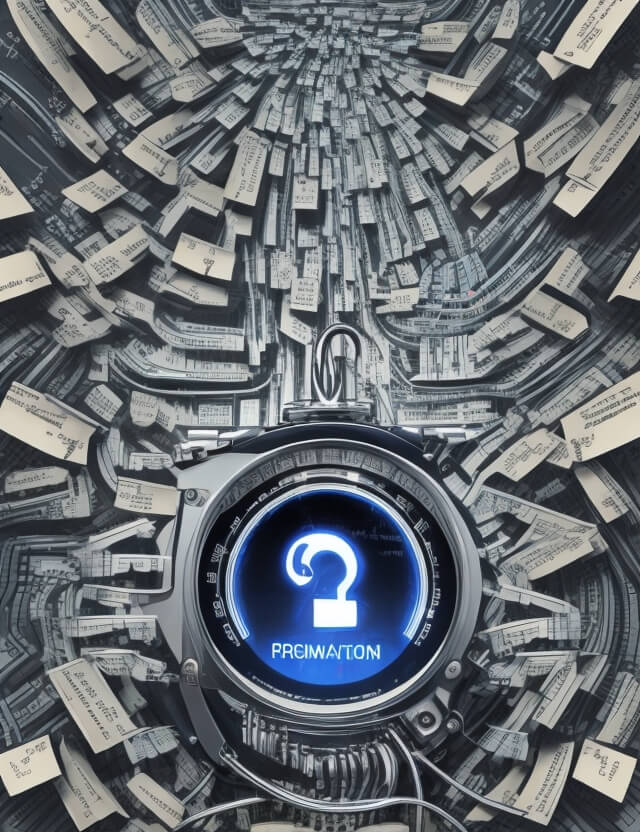
Sample Code
Setup and Requirements:
pip install pycryptodome
Python Script for DES Encryption and Decryption:
des_encryption.py
from Crypto.Cipher import DES
import binascii
# Make sure the key is 8 bytes long
key = b'8bytekey'
def pad(text):
while len(text) % 8 != 0:
text += b' '
return text
def encrypt(message, key):
des = DES.new(key, DES.MODE_ECB)
padded_message = pad(message)
encrypted_text = des.encrypt(padded_message)
return binascii.hexlify(encrypted_text).decode('utf-8')
def decrypt(encrypted_text, key):
des = DES.new(key, DES.MODE_ECB)
decrypted_text = des.decrypt(binascii.unhexlify(encrypted_text))
return decrypted_text.strip().decode('utf-8')
# Example usage
message = b'Hello World'
encrypted = encrypt(message, key)
print(f'Encrypted: {encrypted}')
decrypted = decrypt(encrypted, key)
print(f'Decrypted: {decrypted}')
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Secure Remote Communication Using The DES Algorithm PDF
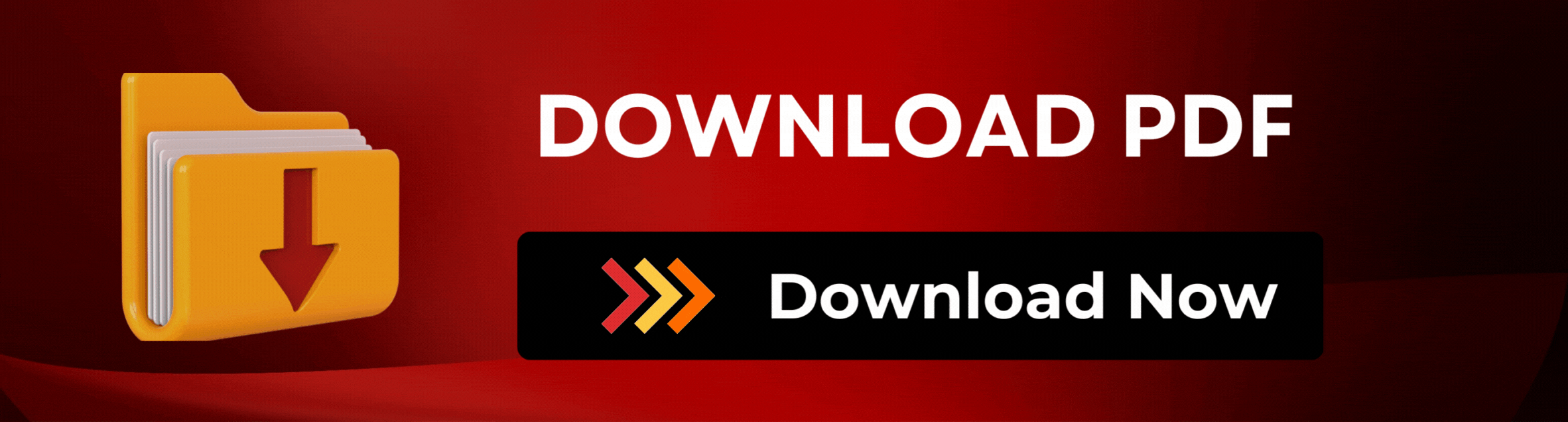