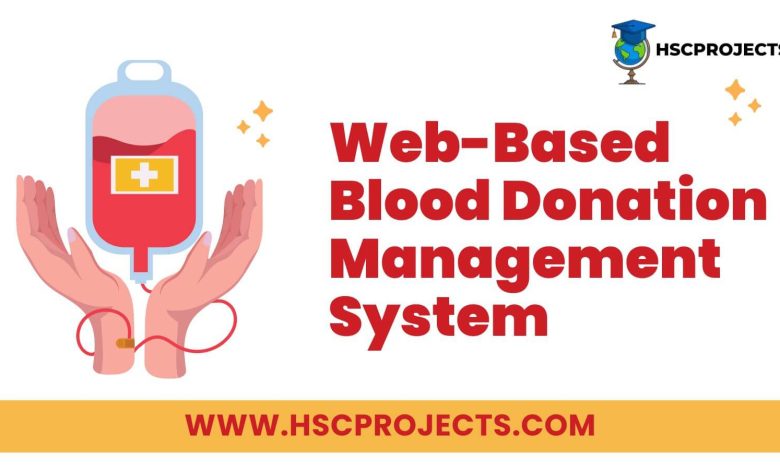
Web-Based Blood Donation Management System
Introduction
Blood donation is a critical component of healthcare systems worldwide. However, traditional methods of connecting donors with recipients have been fraught with inefficiencies and limitations. This article delves into the transformative potential of web-based blood donation management systems, which offer a streamlined, efficient approach to blood donation and transfusion.
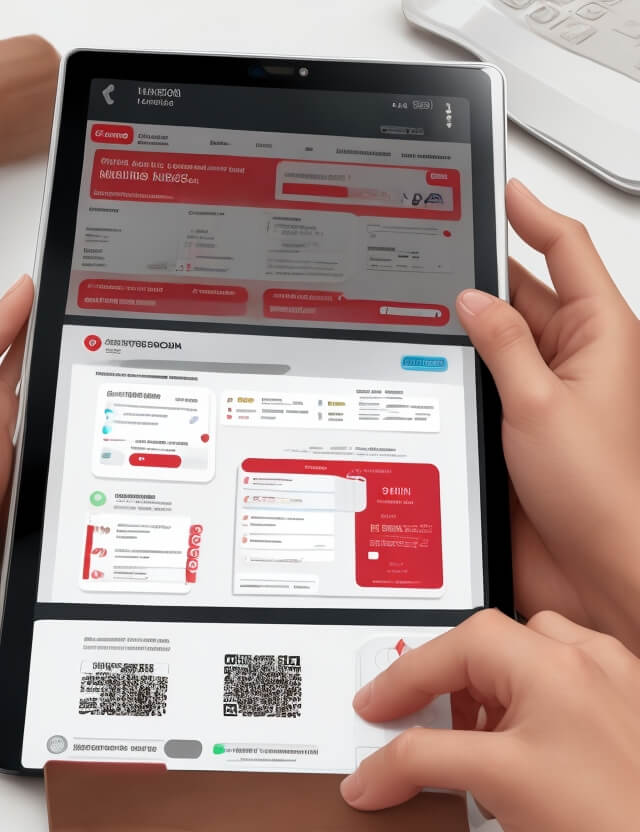
The Problem with Traditional Systems
Traditional blood donation systems often rely on manual processes, which can be time-consuming and error-prone. These systems lack real-time information on blood availability, especially for rare blood types, making it challenging for recipients to find the right match in emergencies.
The Web-Based Solution
Web-based blood donation management systems are designed to overcome these challenges. These platforms maintain comprehensive databases that include not just information about blood donors and blood banks but also about blood donation camps. This makes it easier for potential donors to find opportunities to donate and for recipients to locate the specific blood type they need.
Key Features
- Real-Time Tracking: The system provides real-time updates on blood availability, helping recipients find donors more efficiently.
- Admin and User Modules: The system is designed with separate modules for administrators and users. Administrators can add and manage blood banks and donation camps, while users can easily register as donors or request blood.
- Emergency Notifications: In case of urgent need, the system can send out notifications to potential donors with the required blood type.
Advantages
- Efficiency: The system significantly reduces the time required to connect donors with recipients.
- Accessibility: With the online blood donation system, recipients can easily find donors in their area, even for rare blood types.
- Community Building: The system also helps in organizing blood donation camps, making it easier for donors to contribute to their communities.
Disadvantages
- The primary limitation is the need for an active internet connection, which may not be available in all areas.
Conclusion
Web-based blood donation management systems offer a revolutionary approach to managing the blood donation process. By leveraging technology, these systems make it easier for donors to find opportunities to give and for recipients to find the life-saving blood they need.
Sample Code
First, install Flask:
pip install Flask
Create a file named app.py
:
from flask import Flask, render_template, request, redirect, url_for
app = Flask(__name__)
# Simulated database
users = []
requests = []
@app.route('/')
def home():
return render_template('index.html')
@app.route('/register', methods=['POST'])
def register():
username = request.form.get('username')
blood_type = request.form.get('blood_type')
users.append({'username': username, 'blood_type': blood_type})
return redirect(url_for('home'))
@app.route('/request_blood', methods=['POST'])
def request_blood():
blood_type = request.form.get('blood_type')
requests.append({'blood_type': blood_type})
return redirect(url_for('home'))
@app.route('/view_requests')
def view_requests():
return render_template('view_requests.html', requests=requests)
if __name__ == '__main__':
app.run(debug=True)
Create an HTML file named templates/index.html
:
<!DOCTYPE html>
<html>
<head>
<title>Blood Donation Management System</title>
</head>
<body>
<h1>Welcome to the Blood Donation Management System</h1>
<h2>Register as a Donor</h2>
<form action="/register" method="post">
Username: <input type="text" name="username"><br>
Blood Type: <input type="text" name="blood_type"><br>
<input type="submit" value="Register">
</form>
<h2>Request Blood</h2>
<form action="/request_blood" method="post">
Blood Type: <input type="text" name="blood_type"><br>
<input type="submit" value="Request">
</form>
<a href="/view_requests">View Blood Requests</a>
</body>
</html>
Create another HTML file named templates/view_requests.html
:
<!DOCTYPE html>
<html>
<head>
<title>View Blood Requests</title>
</head>
<body>
<h1>Blood Requests</h1>
<ul>
{% for request in requests %}
<li>Blood Type: {{ request.blood_type }}</li>
{% endfor %}
</ul>
<a href="/">Back to Home</a>
</body>
</html>
To run the example:
- Save the Python code in
app.py
. - Save the HTML code in a folder named
templates
. - Run
app.py
.
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Web-Based Blood Donation Management System PDF
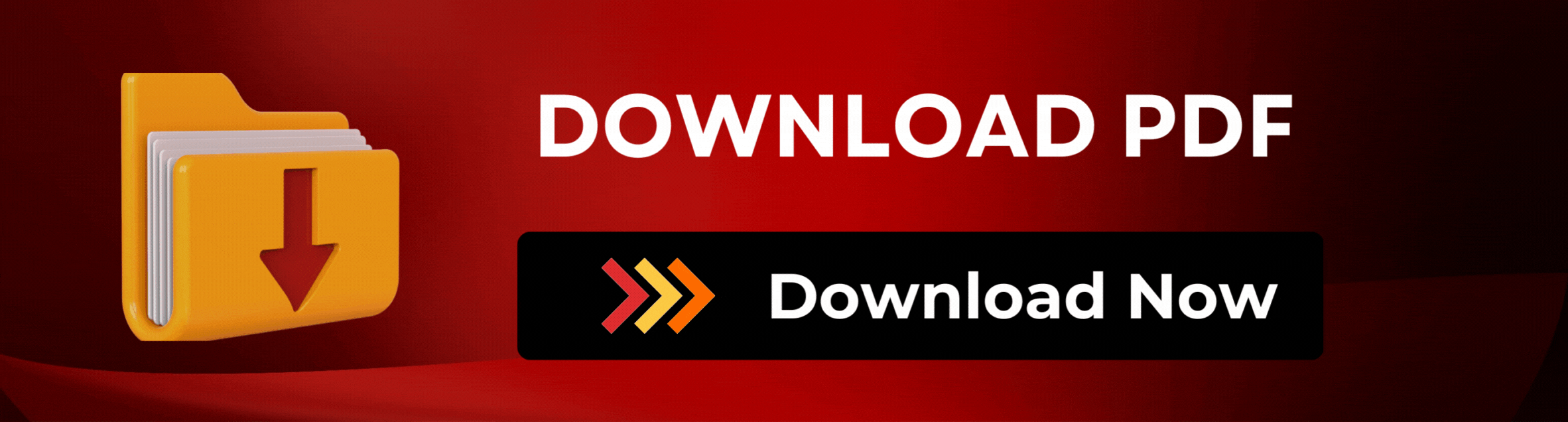