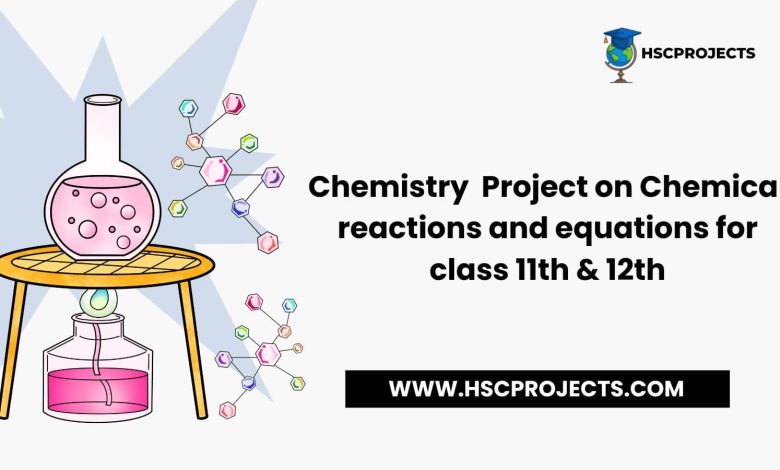
Chemistry Project On Chemical Reactions And Equations For Class 11th & 12th
Introduction
Chemical reactions, those captivating phenomena responsible for the metamorphosis of substances, are an intrinsic part of our daily existence. They are the bedrock of chemistry and hold a multifaceted significance. This all-encompassing project aims to unravel the intricate realm of chemical reactions and equations and their profound implications.
Variety of Chemical Reactions
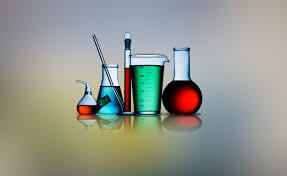
Chemical reactions come in diverse forms, each characterized by its unique attributes:
- Combination Reactions: These reactions witness the union of two or more substances to birth a novel compound. A prime example is the creation of water (H₂O) through synthesis.
- Decomposition Reactions: In stark contrast, decomposition reactions involve the disintegration of a compound into simpler constituents. A classic instance is the breakdown of hydrogen peroxide (H₂O₂) into water (H₂O) and oxygen (O₂).
- Displacement Reactions: This category involves the substitution of atoms or ions in one compound with those from another element. An illustrative case is the displacement of hydrogen from hydrochloric acid (HCl) by metals.
- Double Displacement Reactions: Within these reactions, ions residing in two distinct compounds swap places, ultimately forging new compounds. A tangible example is the reaction between sodium chloride (NaCl) and silver nitrate (AgNO₃).
- Redox Reactions: Redox reactions are characterized by the exchange of electrons between substances. They hold pivotal roles in energy generation and storage, seen in batteries and cellular respiration.
Conveying Chemical Reactions through Equations
Chemical equations serve as concise vehicles to represent these reactions. They employ symbols and notations to convey critical information. For instance, the equation illustrating the combustion of methane (CH₄) in the presence of oxygen (O₂) is articulated as:
CH₄ + 2O₂ → CO₂ + 2H₂O
The Crucial Act of Balancing Chemical Equations: Balancing chemical equations is an essential step, adhering to the Law of Conservation of Mass, which dictates that matter cannot be created nor destroyed in chemical processes. This meticulous process ensures that the count of atoms for each element remains identical on both sides, preserving mass. In the case of methane combustion, it’s imperative to balance the equation to ensure the consistent count of carbon, hydrogen, and oxygen atoms on both sides.
The Law of Conservation of Mass: This law firmly establishes that the total mass of reactants in a chemical reaction equals the total mass of the resulting products. Vibrant demonstrations or experiments substantiate this principle, affirming that matter remains unaltered during chemical metamorphoses. For example, performing a combustion reaction in a sealed environment will confirm the constancy of the total mass.
The Fascination of Chemical Kinetics: Chemical kinetics, a branch of chemistry dedicated to reaction rates, provides insight into the pace at which reactions unfold. Several factors influence these rates, including concentration, temperature, and the presence of catalysts. Augmenting the concentration of reactants generally expedites reactions, while higher temperatures furnish particles with added energy for more effective collisions. Catalysts, on the other hand, expedite reactions without undergoing consumption, showcasing their indispensable role in numerous industrial processes.
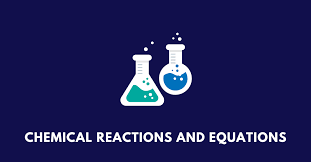
Applications Across Diverse Fields
Chemical reactions boast practical applications across an array of domains:
- Industry harnesses chemical reactions for the synthesis of a vast spectrum of products, spanning from chemicals to pharmaceuticals.
- Medicine relies on chemical reactions for drug synthesis and advancements in the realm of pharmaceuticals.
- Environmental science utilizes chemical reactions to tackle pollution, manage waste, and cultivate sustainable energy sources.
Engaging Case Studies:
Delve into enlightening case studies that spotlight the pivotal role of chemical reactions:
- The Haber Process, a landmark in industrial ammonia production with profound agricultural and industrial ramifications.
- Photosynthesis, the remarkable biological process by which plants convert carbon dioxide and water into glucose and oxygen, elucidating the essentiality of chemistry in sustaining life.
- Combustion reactions, the driving force behind engines and power plants, exemplify the harnessing of chemical energy for useful work.
Conclusion
A profound grasp of chemical reactions and equations is indispensable for anyone venturing into the realm of chemistry. These concepts underpin our ability to comprehend and manipulate transformations in matter, ensuring their omnipresence in both the natural world and our technologically advanced society. This project serves as an illuminating beacon, highlighting the significance of chemical reactions and equations in our world.
Certificate
[Your School Logo]Certificate of Academic Achievement
[Student’s Full Name] [Student’s ID or Enrollment Number] [Date]This is to certify that
[Student’s Full Name]has demonstrated outstanding dedication, commitment, and academic excellence throughout the academic year [Year] at
In recognition of their remarkable accomplishments, we are pleased to present this Certificate of Academic Achievement for [specific achievement or academic excellence].
[Additional information about the achievement, such as GPA, specific subjects, or extracurricular accomplishments] [Principal’s Name] [Principal’s Signature] [School Name] [School Address] [Contact Information]In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Chemistry Project On Chemical Reactions And Equations For Class 11th & 12th PDF
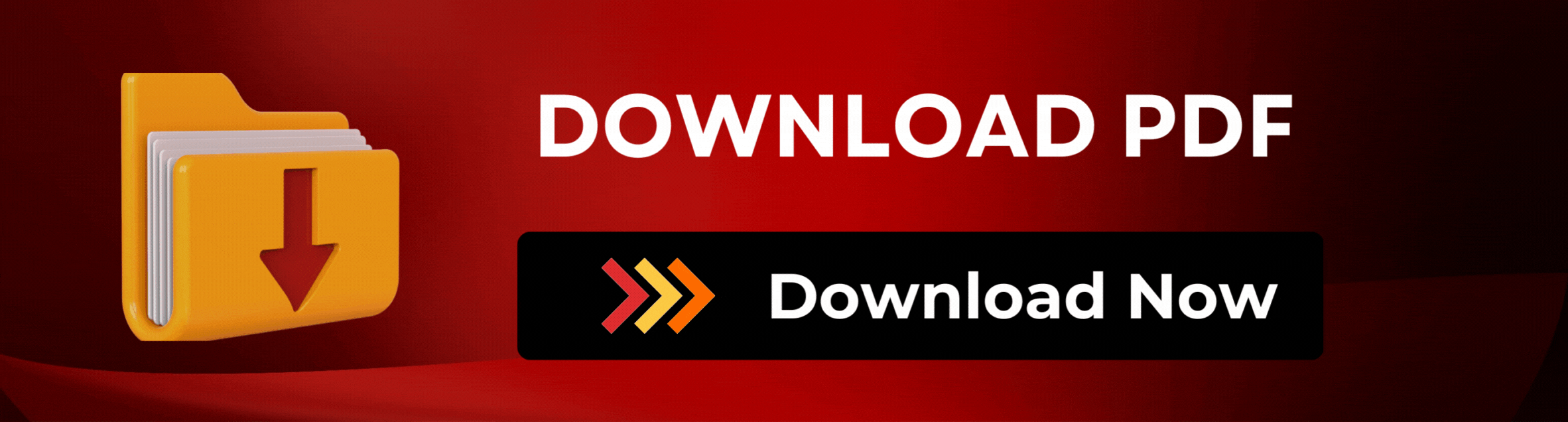