
SQL Injection Prevention and Mitigation in Cyber Security
Introduction
SQL injection is a critical security vulnerability that has plagued web applications for years. Despite its notoriety, it continues to serve as a potent tool for cybercriminals. This article aims to shed light on how to prevent SQL injection attacks effectively. We will delve into coding techniques to prevent SQL injection, SQL injection in DBMS, and its significance in cyber security.

What is SQL Injection?
SQL injection (SQLi) is a type of cyber-attack where an attacker injects malicious SQL code into a web application’s database query. The primary aim is to gain unauthorized access to a database, alter, delete, or even download the entire dataset. This type of attack is highly detrimental and could compromise the security and integrity of an organization.
How Does SQL Injection Work?
In the context of a web form for user authentication, when a user enters their credentials, these values get inserted into a SQL query. If the entered credentials match the database records, access is granted. However, attackers can exploit these forms to input malicious SQL queries, manipulating the database to perform actions that it is not supposed to, such as downloading data.
Coding Techniques to Prevent SQL Injection
To prevent SQL injection attacks, one of the most effective methods is to use Prepared Statements, which ensure that an application checks the data before executing a SQL command. Parameterized queries and stored procedures also serve as robust defenses against SQL injection.
SQL Injection in Cyber Security
SQL injection forms a significant part of the risk landscape in cyber security. It has been used in various high-profile attacks, undermining the security postures of even the most advanced organizations. A key aspect of SQL injection mitigation is continuous monitoring and adapting newer forms of SQL injection prevention techniques.
SQL Injection in DBMS
In the realm of Database Management Systems (DBMS), SQL injection can wreak havoc by altering tables, deleting data, and executing admin operations. It is crucial to implement multiple layers of security like firewalls, input validation, and least privilege access to mitigate the risks associated with SQL injection.
Download SQL Injection Tools for PC
Various tools can help you test your system’s vulnerability to SQL injection. Some popular tools include SQLmap, Havij, and jSQL. These tools can simulate SQL injection attacks, helping you understand potential vulnerabilities.
Advantages of SQL Injection Prevention Systems
- Effectively blocks SQL injection attacks
- Detects and neutralizes malicious code
- Utilizes signature-based authentication techniques to verify authenticity
Conclusion
SQL injection is not a new phenomenon but remains a potent threat. Understanding how to avoid SQL injection through effective coding techniques, regular monitoring, and the use of advanced SQL injection prevention systems can help secure your organization’s valuable data.
Sample Code
pip install Flask
pip install sqlite3
from flask import Flask, request, render_template
import sqlite3
app = Flask(__name__)
# Initialize SQLite database
conn = sqlite3.connect('users.db')
cursor = conn.cursor()
cursor.execute('CREATE TABLE IF NOT EXISTS users (username TEXT, password TEXT)')
conn.close()
@app.route('/')
def index():
return render_template('index.html')
@app.route('/login', methods=['POST'])
def login():
username = request.form['username']
password = request.form['password']
# Use prepared statements to prevent SQL injection
conn = sqlite3.connect('users.db')
cursor = conn.cursor()
cursor.execute('SELECT * FROM users WHERE username = ? AND password = ?', (username, password))
user = cursor.fetchone()
conn.close()
if user:
return "Logged in successfully"
else:
return "Invalid credentials"
if __name__ == '__main__':
app.run(debug=True)
<!DOCTYPE html>
<html>
<head>
<title>Login</title>
</head>
<body>
<h2>Login</h2>
<form method="post" action="/login">
Username: <input type="text" name="username"><br>
Password: <input type="password" name="password"><br>
<input type="submit" value="Login">
</form>
</body>
</html>
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download SQL Injection Prevention and Mitigation in Cyber Security PDF
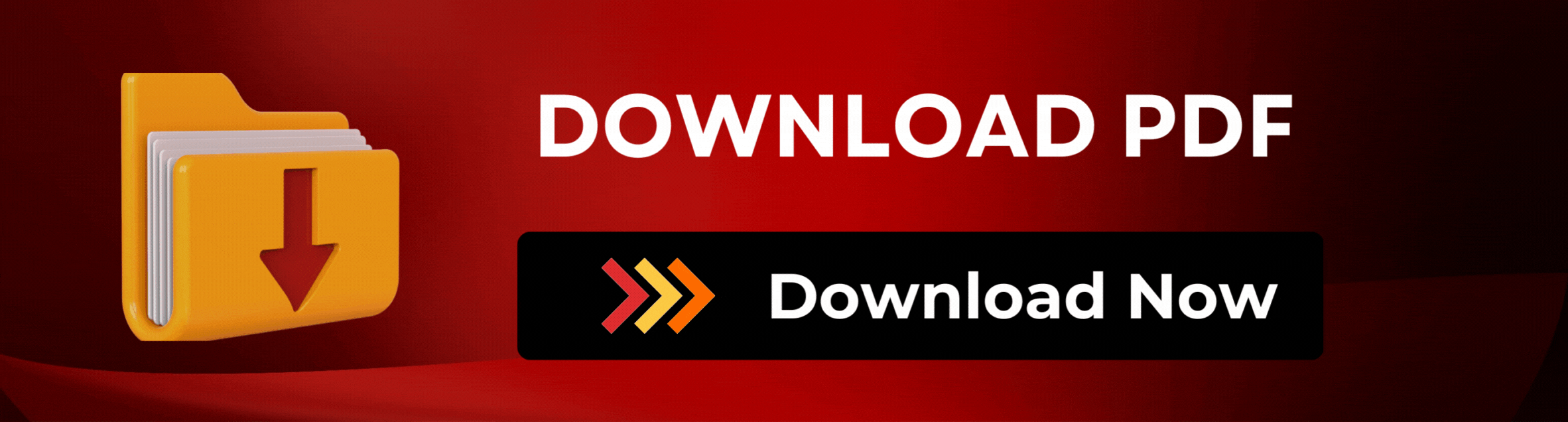