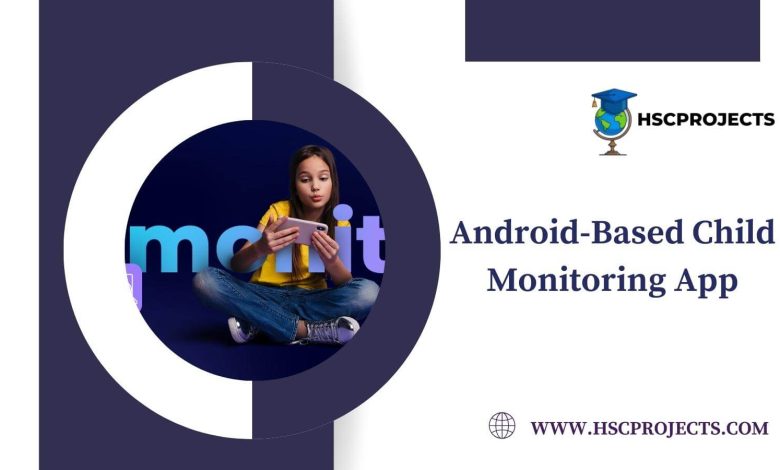
Android-Based Child Monitoring App
Introduction
In today’s digital world, ensuring child safety has moved beyond physical spaces to include virtual realms. That’s where Android-based child monitoring apps come into play. These applications use GPS and telephony services to create a comprehensive child tracking system. This article aims to elaborate on the features, benefits, and limitations of such systems.
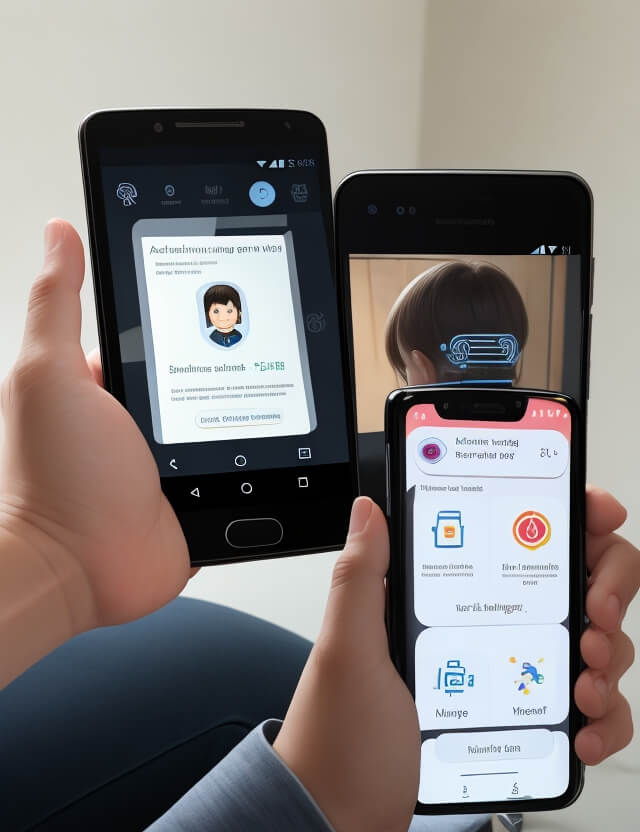
How Does It Work?
The core architecture of a child monitoring app for Android consists of two main services—GPS for location tracking and telephony services for monitoring SMS, call logs, and contacts. To utilize this baby monitoring system, both the parent and the child need GPS-enabled smartphones with an active internet connection.
Features
- Location Tracking: Real-time tracking of your child’s whereabouts.
- Call Logs: Access to a list of incoming and outgoing calls on the child’s phone.
- Messages: Monitoring SMS and other messaging apps.
- Contacts: Details of the saved contacts in the child’s phone.
Advantages of Child Tracking System
- Real-time Monitoring: Parents can track their child’s location in real-time.
- Comprehensive Data: Access to call logs, messages, and contact lists from the child’s phone.
- Accessibility: Parents can retrieve the child’s data anytime, anywhere with an active internet connection.
Limitations
- Requires an active internet connection for both the parent and the child.
- The child needs to log in at least once to activate the system.
- Accuracy may be compromised if data is not entered correctly.
Why Android?
Android OS is chosen for its widespread use, making it easier to target a larger user base. Its user-friendly interface ensures an easy-to-use experience for both parents and children.
Conclusion
Android-based child monitoring apps offer a robust solution for parents concerned about their child’s safety. These apps enable real-time tracking and offer various telephony services, making them a comprehensive child tracking system. However, it is crucial to be aware of the limitations and ensure proper setup to maximize benefits.
Sample Code
Add these permissions in AndroidManifest.xml:
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION"/>
<uses-permission android:name="android.permission.READ_CALL_LOG"/>
MainActivity.java
import android.Manifest;
import android.content.pm.PackageManager;
import android.location.Location;
import android.location.LocationListener;
import android.location.LocationManager;
import android.os.Bundle;
import android.provider.CallLog;
import android.widget.TextView;
import androidx.annotation.NonNull;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.app.ActivityCompat;
public class MainActivity extends AppCompatActivity {
private TextView locationText;
private TextView callLogsText;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
locationText = findViewById(R.id.locationText);
callLogsText = findViewById(R.id.callLogsText);
// Request permissions
ActivityCompat.requestPermissions(this, new String[]{Manifest.permission.ACCESS_FINE_LOCATION, Manifest.permission.READ_CALL_LOG}, 1);
}
@Override
public void onRequestPermissionsResult(int requestCode, @NonNull String[] permissions, @NonNull int[] grantResults) {
if (requestCode == 1) {
if (grantResults.length > 0 && grantResults[0] == PackageManager.PERMISSION_GRANTED) {
fetchLocation();
fetchCallLogs();
}
}
}
private void fetchLocation() {
LocationManager locationManager = (LocationManager) getSystemService(LOCATION_SERVICE);
LocationListener locationListener = new LocationListener() {
@Override
public void onLocationChanged(@NonNull Location location) {
locationText.setText("Latitude: " + location.getLatitude() + ", Longitude: " + location.getLongitude());
}
};
locationManager.requestLocationUpdates(LocationManager.GPS_PROVIDER, 0, 0, locationListener);
}
private void fetchCallLogs() {
// Simplified, fetching last call for example
Cursor managedCursor = getContentResolver().query(CallLog.Calls.CONTENT_URI, null, null, null, null);
int number = managedCursor.getColumnIndex(CallLog.Calls.NUMBER);
if (managedCursor.moveToLast()) {
String phNumber = managedCursor.getString(number);
callLogsText.setText("Last Call: " + phNumber);
}
managedCursor.close();
}
}
activity_main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/locationText"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Location will appear here"/>
<TextView
android:id="@+id/callLogsText"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Call logs will appear here"
android:layout_below="@id/locationText"/>
</RelativeLayout>
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Android-Based Child Monitoring App PDF
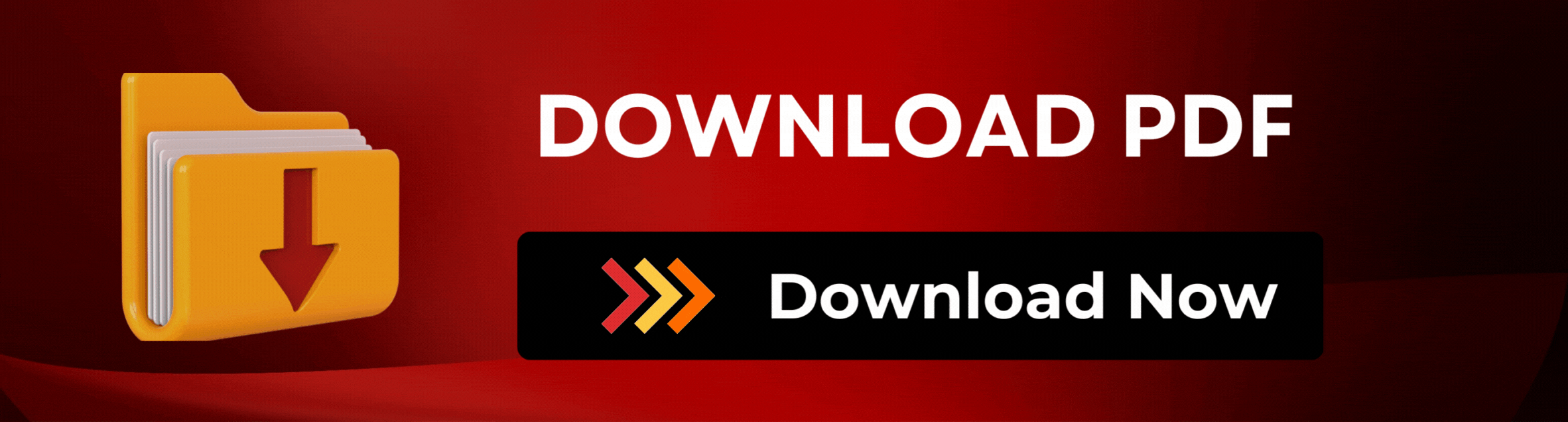