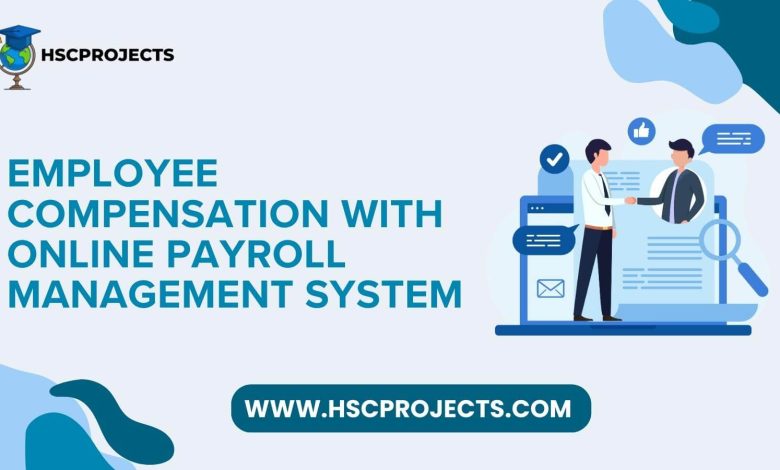
Employee Compensation with Online Payroll Management System
Introduction
In today’s fast-paced business environment, efficiency is key. Traditional methods of managing employee payroll are not only time-consuming but also prone to errors. Enter the Online Payroll Management System—a game-changing solution that automates the entire process of salary management. This article delves into the features, advantages, and minor limitations of implementing a payroll management system project in your organization.
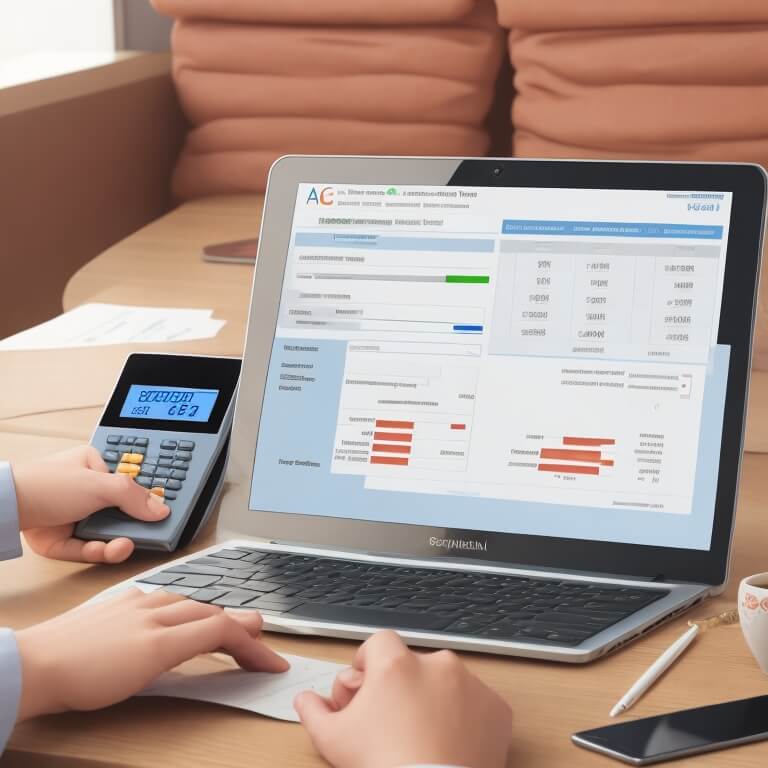
What is a Payroll Management System?
A payroll management system is a software application designed to automate the process of paying the company’s employees. It takes care of everything from calculating salaries and deductions to generating payslips and tax forms. In essence, it transforms the complex task of HR and salary management into a few simple clicks.
Core Features
- Automated Salary Calculation: Based on the attendance and leave records, the system automatically calculates the salary for each employee.
- Leave Management: The system keeps track of each employee’s leaves, ensuring accurate salary calculations.
- Instant Payslip Generation: Gone are the days of manual payslip generation. The system generates digital payslips that are emailed to employees.
- Employee Management: HR can easily add, update, or remove employee details in the system.
Advantages of Online Payroll Management System
- Efficiency: The system significantly reduces the time spent on payroll processing.
- Accuracy: Automated calculations minimize the risk of human error.
- Accessibility: Being an online system, it can be accessed from anywhere at any time, offering unparalleled convenience.
- Environmentally Friendly: It eliminates the need for paper, contributing to sustainability.
Minor Limitations
- Internet Dependency: The only downside is that it requires an active internet connection for access.
Conclusion
The Online Payroll Management System is an indispensable tool for any organization looking to modernize its payroll processes. It not only saves time but also increases the accuracy and efficiency of the HR department. With features like automated salary calculation and instant payslip generation, it’s time to say goodbye to manual processes and embrace the future of payroll management.
Sample Code
First, install the required packages:
pip install Flask
pip install Flask-SQLAlchemy
from flask import Flask, request, jsonify
from flask_sqlalchemy import SQLAlchemy
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///employees.db'
db = SQLAlchemy(app)
class Employee(db.Model):
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(50), nullable=False)
salary = db.Column(db.Float, nullable=False)
attendance = db.Column(db.Integer, nullable=False)
def __repr__(self):
return f"Employee('{self.name}', '{self.salary}', '{self.attendance}')"
@app.route('/add_employee', methods=['POST'])
def add_employee():
data = request.get_json()
new_employee = Employee(name=data['name'], salary=data['salary'], attendance=data['attendance'])
db.session.add(new_employee)
db.session.commit()
return jsonify({"message": "Employee added"}), 201
@app.route('/calculate_salary/<int:emp_id>', methods=['GET'])
def calculate_salary(emp_id):
employee = Employee.query.get_or_404(emp_id)
final_salary = employee.salary * employee.attendance
return jsonify({"final_salary": final_salary})
if __name__ == '__main__':
db.create_all()
app.run(debug=True)
To run this example:
- Save the code in a file, say
app.py
. - Run
app.py
to start the Flask application. - Use Postman or curl to test the API endpoints
To add an employee:
curl --request POST \
--url http://127.0.0.1:5000/add_employee \
--header 'Content-Type: application/json' \
--data '{
"name": "John",
"salary": 2000,
"attendance": 20
}'
To calculate the salary of an employee with ID 1:
curl --request GET \
--url http://127.0.0.1:5000/calculate_salary/1
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Employee Compensation with Online Payroll Management System PDF
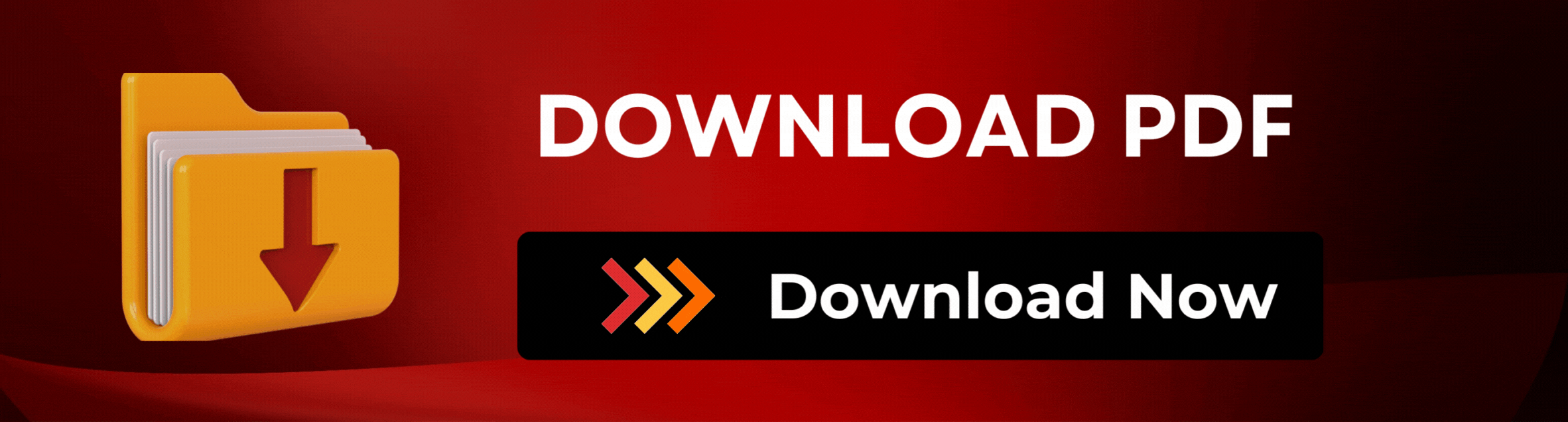