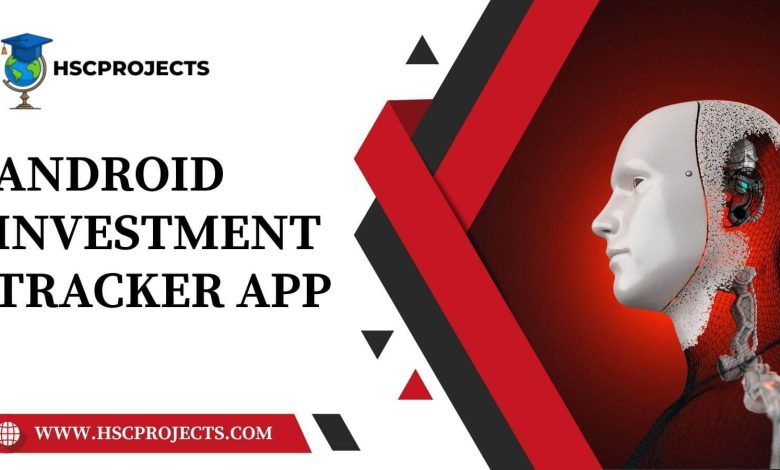
Android Investment Tracker App
Introduction
Investing has never been easier, thanks to the advent of technology. The Android Investment Tracker App is specifically designed to serve as your personal financial assistant. No longer do you have to sift through paperwork or use cumbersome spreadsheets; this investment tracking app consolidates all your financial data in one place.

How Does it Work?
Built with cutting-edge Android-Java for the front-end and SQLite for the back-end, our application is optimized for high performance. The IDE of choice is Android Studio, offering a seamless development environment for both amateurs and professionals. To get started, simply sign up with a username and password, and you’ll gain access to a comprehensive dashboard. Here, you’ll find an overview of your total investments and expected returns.
Features
- Easy to Maintain: The app offers easy navigation, making it simpler to input new investments and keep track of existing ones.
- User-Friendly Interface: With an intuitive design, even those who are not tech-savvy can manage their investments effortlessly.
- Asset Management: The app allows you to add new assets by entering relevant information, making it convenient to keep track of diversified portfolios.
- Real-Time Updates: The app updates the list of your assets and their performance metrics in real-time, providing accurate data at your fingertips.
- Modification and Deletion: You have complete control over your investment data. Modify or delete assets whenever you need to restructure your portfolio.
Advantages
The primary benefit of using our Android Investment Tracker App is the convenience it offers. Here are some of the standout advantages:
- Efficient Tracking: Never miss a beat when it comes to your investments. Monitor their performance and make informed decisions.
- User Convenience: Why be confined to a desktop when you can manage your assets on the go?
- Security: With robust backend encryption, your financial data is in safe hands.
Conclusion
The Android Investment Tracker App is not just an application; it’s your financial companion. Efficient, secure, and user-friendly, this app is a must-have tool for anyone serious about making the most of their investments.
Sample Code
XML layout (‘activity_main.xml
‘):
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<Button
android:id="@+id/btn_addInvestment"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Add Investment"/>
<ListView
android:id="@+id/list_investments"
android:layout_width="match_parent"
android:layout_height="wrap_content">
</ListView>
</LinearLayout>
The Java class (‘MainActivity.java
‘):
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.ListView;
import android.widget.Toast;
public class MainActivity extends Activity {
InvestmentDbHelper dbHelper;
ListView listView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
dbHelper = new InvestmentDbHelper(this);
listView = findViewById(R.id.list_investments);
Button addInvestment = findViewById(R.id.btn_addInvestment);
addInvestment.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Your logic for adding investment
dbHelper.addInvestment("Stock", 1000);
Toast.makeText(MainActivity.this, "Investment Added", Toast.LENGTH_SHORT).show();
}
});
// Initialize and populate listView
// ...
}
}
The SQLite helper class for database operations (‘InvestmentDbHelper.java
‘):
import android.content.ContentValues;
import android.content.Context;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteOpenHelper;
public class InvestmentDbHelper extends SQLiteOpenHelper {
private static final String DATABASE_NAME = "investments.db";
private static final int DATABASE_VERSION = 1;
public InvestmentDbHelper(Context context) {
super(context, DATABASE_NAME, null, DATABASE_VERSION);
}
@Override
public void onCreate(SQLiteDatabase db) {
String SQL_CREATE_INVESTMENTS_TABLE = "CREATE TABLE investments ("
+ "id INTEGER PRIMARY KEY AUTOINCREMENT, "
+ "name TEXT NOT NULL, "
+ "amount INTEGER NOT NULL);";
db.execSQL(SQL_CREATE_INVESTMENTS_TABLE);
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
// Handle database upgrade if needed
}
public void addInvestment(String name, int amount) {
SQLiteDatabase db = getWritableDatabase();
ContentValues values = new ContentValues();
values.put("name", name);
values.put("amount", amount);
db.insert("investments", null, values);
}
}
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Android Investment Tracker App PDF
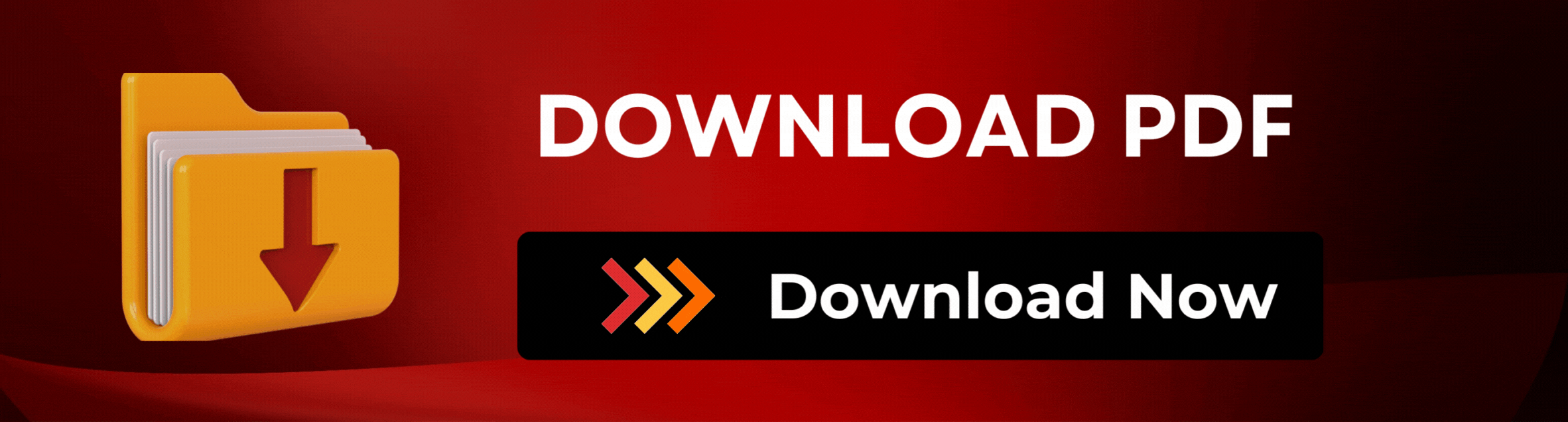